Running Ant Targets in Maven: A How-To Guide
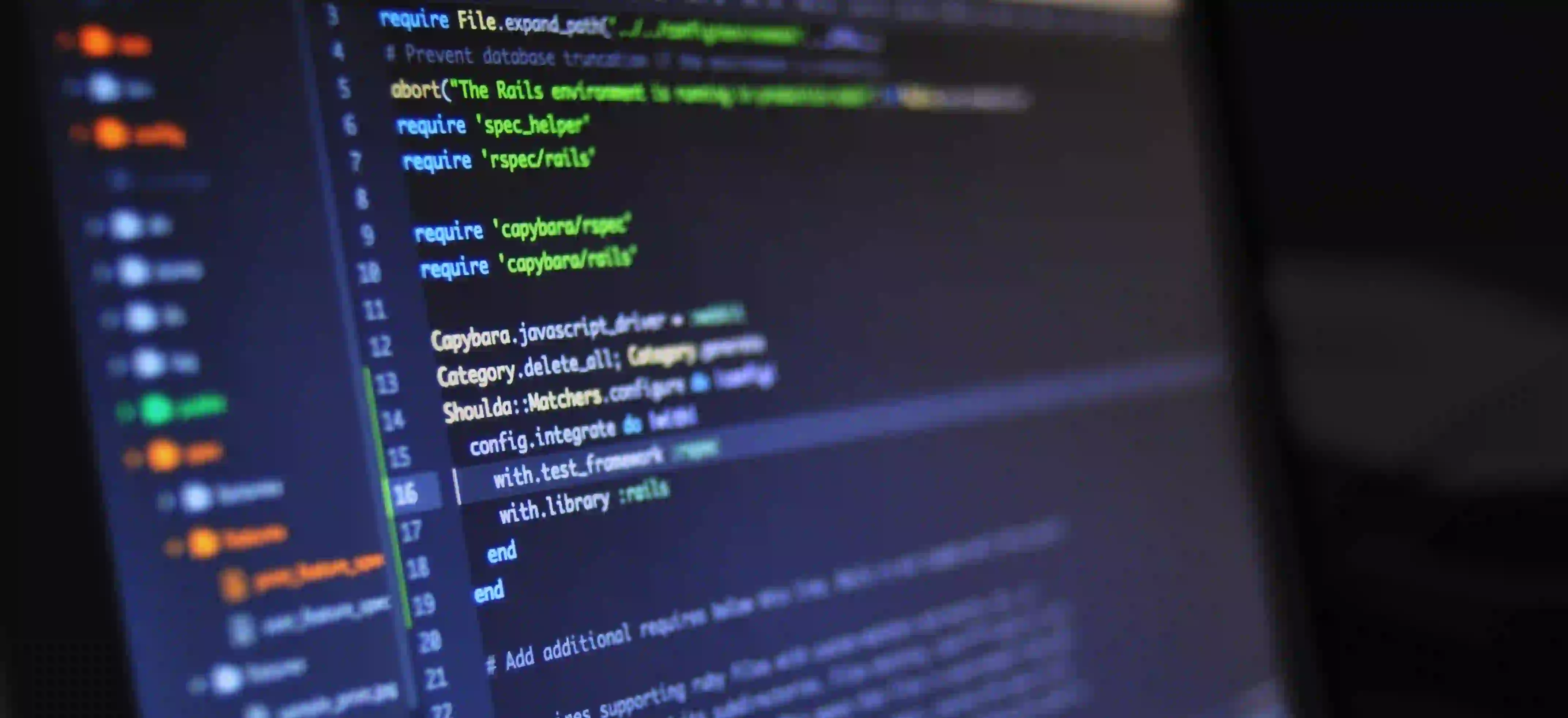
Running Ant Targets in Maven: A How-To Guide
In the world of Java development, Apache Maven has established itself as a powerful and versatile build automation tool. However, there are times when you may have an existing Ant build script that you want to integrate with Maven. This could be due to legacy projects or because certain tasks are better suited to Ant.
In this guide, we'll explore how you can run Ant targets within a Maven build. We'll cover the necessary configurations and provide code examples to illustrate the process.
Why Run Ant Targets in Maven?
Before delving into the technical details, let's briefly discuss why you might want to run Ant targets within a Maven build. While Maven provides a wide range of plugins for various tasks, there are situations where Ant excels. For instance, if you have complex, custom build processes already defined in Ant, migrating everything to Maven may not be practical.
Additionally, leveraging Ant's built-in tasks or custom tasks developed over time can be advantageous. By integrating Ant targets into Maven, you can capitalize on the strengths of both tools and streamline your build process.
Configuring Maven to Run Ant Targets
To execute Ant targets within a Maven build, you'll need to use the maven-antrun-plugin. This plugin allows you to run Ant tasks and targets as part of your Maven build lifecycle. Here's a basic example of how you can configure the maven-antrun-plugin in your pom.xml to run an Ant target:
<build>
<plugins>
<plugin>
<artifactId>maven-antrun-plugin</artifactId>
<executions>
<execution>
<phase>compile</phase>
<goals>
<goal>run</goal>
</goals>
<configuration>
<target>
<!-- Your Ant target goes here -->
</target>
</configuration>
</execution>
</executions>
</plugin>
</plugins>
</build>
In this configuration, we've specified the maven-antrun-plugin and defined an execution within the <executions>
block. We've set the phase to 'compile' to run the Ant target during the Maven compile phase. Inside the <configuration>
block, you can define the specific Ant target you want to run.
Understanding the Configuration
The maven-antrun-plugin
configuration revolves around the <execution>
and <configuration>
elements. By specifying the phase, you determine when the Ant target should be executed within the Maven build lifecycle. The <target>
element allows you to define the actual Ant target that will be run.
Why Use Maven's Phases?
Maven's build lifecycle is organized into phases, each representing a stage in the build process. By associating the execution of an Ant target with a specific phase, you can effectively integrate the target into the overall build flow. For instance, attaching the target to the compile
phase ensures it runs when the project is compiled.
Example: Running Ant Clean Target in Maven
Let's illustrate the above configuration with a practical example. Suppose you have an Ant build script with a 'clean' target to delete build artifacts. You want to incorporate this into your Maven build process.
First, add the maven-antrun-plugin to your pom.xml as shown above. Then, within the <target>
element, specify the Ant 'clean' target:
<target>
<ant antfile="build.xml" target="clean" />
</target>
In this snippet, we're using the <ant>
task within the <target>
element to execute the 'clean' target defined in the build.xml file. The antfile
attribute specifies the location of the Ant build script, and the target
attribute indicates the specific target to execute.
Why Use the ant Task?
By utilizing the <ant>
task, you can seamlessly invoke Ant targets within the Maven build. This approach facilitates reusing existing Ant build scripts without needing to refactor them into Maven-specific constructs.
Handling Ant Properties and Dependencies
When running Ant targets in Maven, you may encounter scenarios where you need to pass properties or handle dependencies. The maven-antrun-plugin allows you to address these requirements efficiently.
Passing Properties to Ant
Suppose your Ant target expects certain properties to be set. You can pass these properties from Maven to Ant as follows:
<configuration>
<target>
<property name="foo" value="bar" />
<!-- Other Ant tasks go here -->
</target>
</configuration>
In this snippet, the <property>
element within the <target>
block sets the 'foo' property to 'bar', making it available to the Ant target.
Managing Dependencies
If your Ant build script depends on external libraries or JAR files, you can manage these dependencies within the maven-antrun-plugin configuration. For example, you can use the maven-dependency-plugin to copy dependencies to a specified directory before running the Ant target:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-dependency-plugin</artifactId>
<executions>
<execution>
<phase>generate-sources</phase>
<goals>
<goal>copy-dependencies</goal>
</goals>
<configuration>
<outputDirectory>${project.build.directory}/ant-lib</outputDirectory>
</configuration>
</execution>
</executions>
</plugin>
In this example, the maven-dependency-plugin will copy the project dependencies to the 'ant-lib' directory during the generate-sources
phase, ensuring that the Ant target has access to the required libraries.
Closing Remarks
Integrating Ant targets into your Maven build can enhance the flexibility and efficiency of your build process. By leveraging the maven-antrun-plugin, you can seamlessly incorporate existing Ant build scripts and make use of custom tasks within a Maven environment.
In this guide, we've explored the configuration of the maven-antrun-plugin, demonstrated running an Ant 'clean' target in a Maven build, and addressed handling properties and dependencies. This allows you to streamline your build process and leverage the strengths of both Maven and Ant.
By understanding and harnessing the interoperability between Maven and Ant, you can optimize your build automation and achieve a more robust and adaptable development workflow.
Remember, while Maven and Ant each have their strengths, knowing how to combine them effectively can truly elevate your Java development projects to new heights.