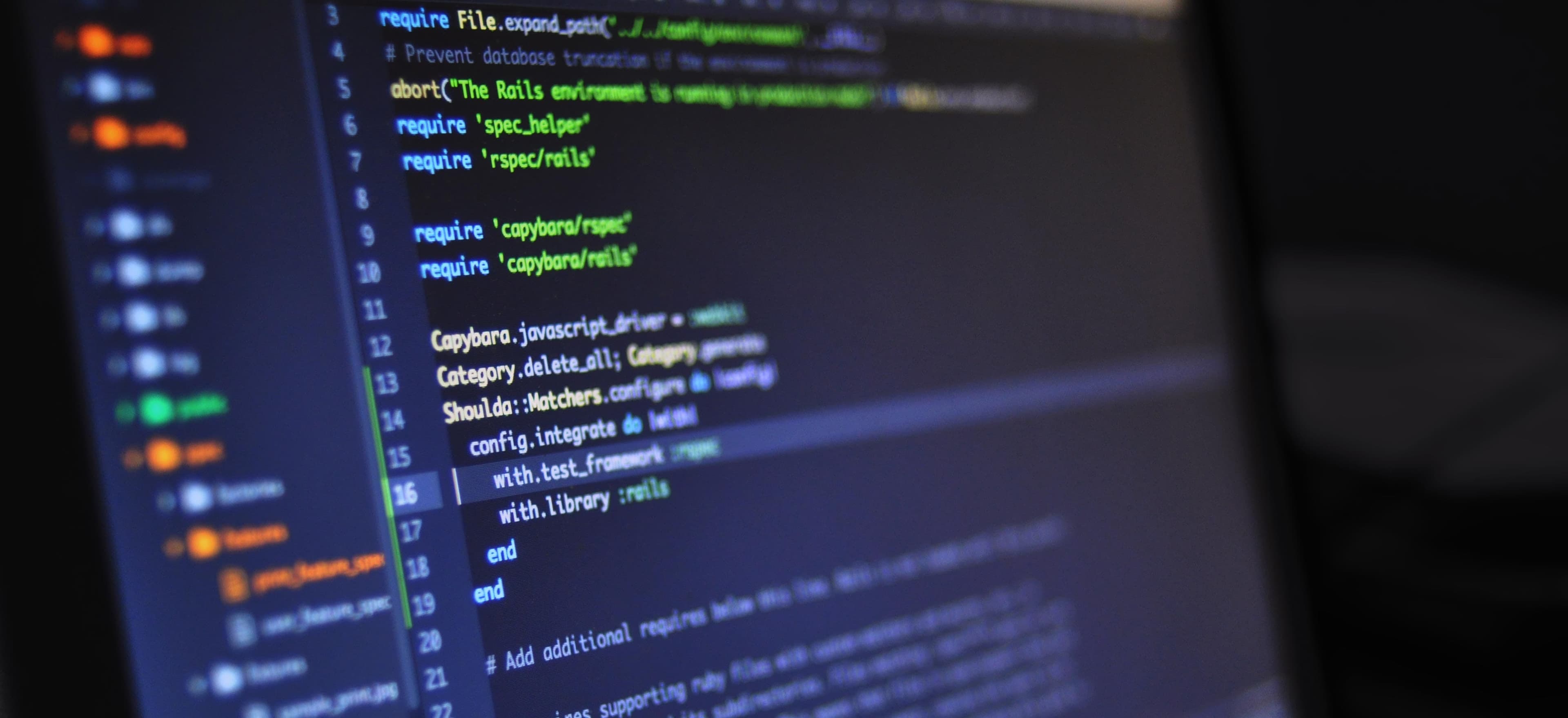
- Published on
Addressing Security Challenges in DevOps with Java
In today's fast-paced software development landscape, the adoption of DevOps practices has become increasingly prevalent. DevOps, as a culture, emphasizes collaboration, automation, and monitoring, aiming to shorten the development lifecycle and provide continuous delivery with high software quality. However, amidst the rapid delivery cycles, security can often be overlooked, leading to potential vulnerabilities and threats. In this article, we will explore how Java, as a widely-used programming language, can be leveraged to address security challenges in DevOps.
Understanding the Security Challenges
Before delving into the solutions, it's crucial to understand the security challenges that can arise in the DevOps environment. Some of the common challenges include:
-
Continuous Integration/Continuous Deployment (CI/CD) Pipelines: The automated nature of CI/CD pipelines can become a potential point of security vulnerability if not properly managed. Vulnerabilities in the code, dependencies, or misconfigurations can be inadvertently introduced into the deployment process.
-
Container Security: With the widespread adoption of containerization using platforms like Docker, ensuring the security of container images and the orchestrated containers is paramount. Vulnerabilities in container images or misconfigurations can pose significant risks.
-
API Security: As modern applications heavily rely on APIs for communication and integration, securing APIs against potential threats such as injection attacks, broken authentication, and sensitive data exposure is vital.
Leveraging Java for Secure DevOps Practices
Java, known for its strong focus on security and robustness, provides a variety of tools and frameworks that can be instrumental in addressing the security challenges in a DevOps environment. Let's delve into some notable strategies and tools:
Static Code Analysis with FindBugs
Static code analysis helps in identifying potential security vulnerabilities, coding errors, and bad practices within the codebase. FindBugs, a widely-used static analysis tool for Java, can be seamlessly integrated into the CI/CD pipelines to perform thorough code scans. By leveraging FindBugs, teams can proactively identify and rectify security issues at an early stage, ensuring that only secure code gets deployed.
// Example of a code snippet with a security vulnerability
public void executeQuery(String query) {
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery(query); // SQL injection vulnerability
// Process the result set
}
Dependency Scanning with OWASP Dependency-Check
Managing dependencies is crucial in ensuring the security of an application. Vulnerabilities in third-party libraries and components can pose significant risks. OWASP Dependency-Check is a powerful tool that can be utilized to scan project dependencies and identify known vulnerabilities. By incorporating it into the CI/CD process, teams can automatically detect and remediate any vulnerable dependencies, thereby enhancing the overall security posture.
Container Security with Java APIs
When it comes to securing containerized applications, Java provides robust APIs that facilitate secure coding practices within the containerized environment. By utilizing Java APIs for secure input/output handling, cryptographic operations, and secure communication, developers can fortify their containerized applications against common security threats.
// Example of using Java APIs for secure communication within a containerized application
SSLContext context = SSLContext.getInstance("TLS");
context.init(null, trustManagers, new SecureRandom());
HttpsURLConnection.setDefaultSSLSocketFactory(context.getSocketFactory());
Security Testing with JUnit and OWASP ZAP
Automated testing forms an integral part of the CI/CD process. By leveraging JUnit, a popular testing framework for Java, alongside OWASP ZAP (Zed Attack Proxy) for security testing, teams can automate the execution of security tests within their pipelines. This ensures that security testing becomes an inherent part of the automated deployment process, enabling rapid feedback on the application's security posture.
// Example of using JUnit for security testing
@Test
public void testAuthentication() {
// Perform authentication tests
assertTrue(authenticationService.authenticate("username", "password"));
}
Key Takeaways
In the realm of DevOps, integrating security practices seamlessly into the development and deployment pipelines is imperative. Java, with its emphasis on security and a rich ecosystem of tools and frameworks, can play a pivotal role in fortifying the DevOps practices against potential security challenges. By incorporating static code analysis, dependency scanning, leveraging Java APIs for container security, and automating security testing, teams can ensure that security remains a prime focus throughout the DevOps lifecycle.
As organizations continue their journey towards DevOps maturity, prioritizing security alongside speed and agility will be essential to maintain the integrity of the software delivery process. With Java's robust security capabilities and the right set of practices and tools, DevOps teams can navigate the security landscape with confidence, fostering a culture of secure and efficient software delivery.
In conclusion, by embracing Java's security features, integrating robust security practices, and leveraging the vibrant ecosystem of security tools and frameworks, DevOps teams can effectively address the security challenges and build a resilient and secure software delivery pipeline.
Remember, security and agility can go hand in hand, and Java is here to make that happen.
Adapt, innovate, and secure your DevOps journey with Java! 🚀
Checkout our other articles