Optimizing JVM Performance with Apache Arrow
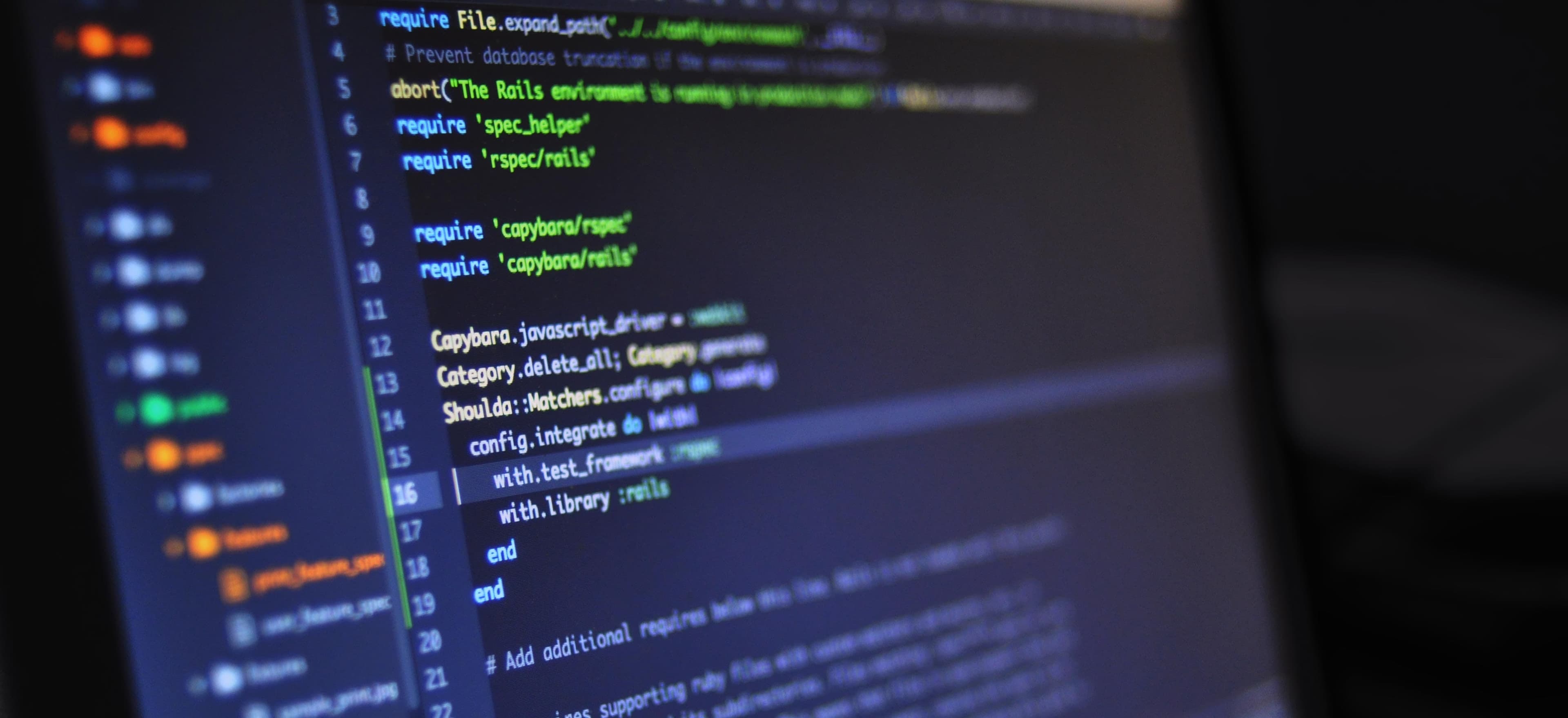
- Published on
Optimizing JVM Performance with Apache Arrow
Java Virtual Machine (JVM) performance optimization is crucial for ensuring the efficiency of Java applications, especially those dealing with large datasets. In this article, we will explore how Apache Arrow can be used to enhance JVM performance by improving memory management and data processing.
Understanding Apache Arrow
Apache Arrow is a cross-language development platform for in-memory data that specifies a standardized language-independent columnar memory format for flat and hierarchical data, organized for efficient analytic operations on modern hardware. It provides a set of technologies that enable big data systems to process and move data more effectively.
Benefits of Apache Arrow for JVM Performance
Efficient Data Representation
Apache Arrow's columnar memory format represents data in a way that is highly optimized for modern hardware architectures. This allows for better cache locality and vectorized processing, which are essential for improving JVM performance, especially when dealing with large datasets.
Memory Offloading
By utilizing Apache Arrow, Java applications can offload memory more efficiently, reducing the overhead associated with Java's native memory management. This leads to better memory utilization and improved garbage collection performance, resulting in overall enhanced JVM performance.
Zero-Copy Data Sharing
Apache Arrow enables zero-copy data sharing between different components of the software stack. This means that data can be transferred between systems or processes without the need for unnecessary data copying, resulting in significant performance improvements and reduced memory consumption.
Integrating Apache Arrow with Java
Using Apache Arrow in Java
To integrate Apache Arrow with Java, you can utilize the arrow-java
library, which provides Java bindings for Apache Arrow. This allows Java applications to work with Arrow data structures and benefit from the performance optimizations it offers.
Serialization and Deserialization
Apache Arrow provides efficient serialization and deserialization methods for data, allowing seamless integration with Java applications. This ensures that data can be processed and transferred between systems with minimal overhead, contributing to improved JVM performance.
Working with Arrow Data Structures
Let's take a look at an example of how we can work with Arrow data structures in Java:
import org.apache.arrow.memory.RootAllocator;
import org.apache.arrow.vector.Float8Vector;
import org.apache.arrow.vector.types.pojo.FieldType;
import org.apache.arrow.vector.types.pojo.Schema;
// Create a root allocator
RootAllocator allocator = new RootAllocator();
// Define a field type
FieldType fieldType = new FieldType(false, new ArrowType.FloatingPoint(FloatingPointPrecision.DOUBLE), null);
// Create a Float8Vector with the defined field type
Float8Vector vector = new Float8Vector("myVector", fieldType, allocator);
// Set values in the vector
vector.setSafe(0, 10.5);
// Access values from the vector
double value = vector.get(0);
In this example, we are creating a Float8Vector
using Apache Arrow's Java bindings and performing operations to set and access values within the vector.
Optimizing JVM Performance with Apache Arrow
Now, let's discuss how Apache Arrow can be leveraged to optimize JVM performance in Java applications.
Efficient Data Processing
Apache Arrow's columnar memory format and vectorized processing capabilities enable Java applications to process data more efficiently. By utilizing Arrow's data structures and algorithms, developers can optimize data processing pipelines for improved performance.
Reduced Memory Overhead
Java applications often face challenges related to memory management and garbage collection. By leveraging Apache Arrow's memory offloading capabilities and zero-copy data sharing, Java applications can significantly reduce memory overhead, leading to improved JVM performance and lower resource consumption.
Interoperability with Other Technologies
Apache Arrow facilitates seamless interoperability between different technologies and programming languages. Java applications can benefit from Arrow's standardized data format when interacting with other systems, enabling efficient data exchange and improved performance across the software stack.
Parallel Execution
Apache Arrow enables parallel execution of data processing tasks, leveraging modern hardware architectures to achieve higher throughput and lower latency. Java applications can take advantage of Arrow's parallel processing capabilities to improve performance in multi-threaded and distributed computing scenarios.
Closing Remarks
In conclusion, Apache Arrow offers significant benefits for optimizing JVM performance in Java applications. By leveraging its efficient data representation, memory offloading, and zero-copy data sharing capabilities, developers can enhance the performance of data-intensive Java applications. Integrating Apache Arrow with Java allows for seamless data processing and serialization, leading to improved JVM performance and better utilization of hardware resources.
Apache Arrow's interoperability and support for parallel execution further contribute to its value in optimizing JVM performance. As Java continues to be a popular choice for developing data-intensive applications, leveraging Apache Arrow can provide a competitive advantage by improving efficiency and scalability in handling large datasets.
Incorporating Apache Arrow into JVM performance optimization strategies can result in tangible performance improvements and cost savings for organizations running Java applications at scale.
By adopting Apache Arrow, Java developers can tap into a powerful toolset for optimizing JVM performance and ensuring the efficient processing of large-scale data workloads.
For more in-depth insights into Apache Arrow and its impact on JVM performance, you can refer to the official Apache Arrow documentation.
Start leveraging Apache Arrow today to unlock the full potential of your Java applications and elevate their performance to new heights!