Choosing Between Constructors and Static Factory Methods
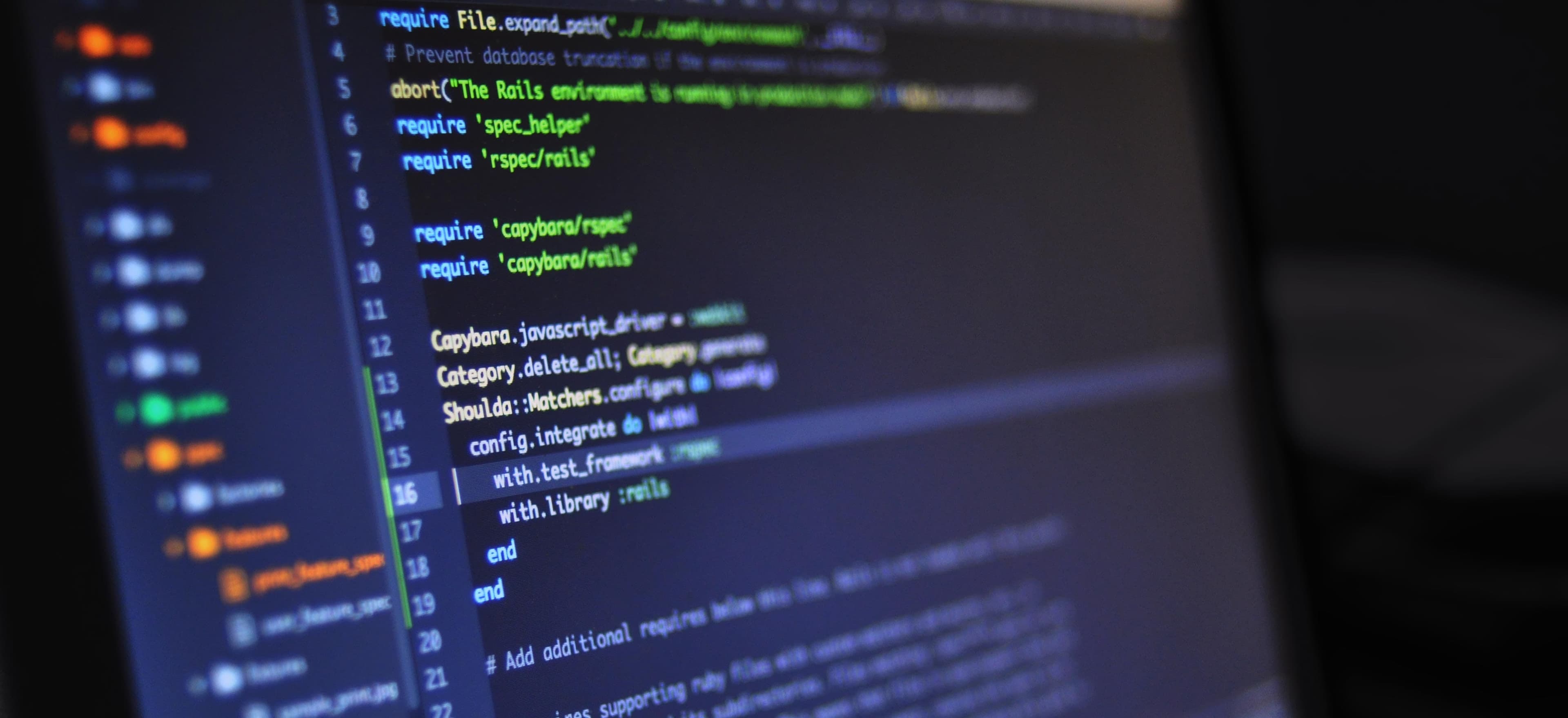
- Published on
When it comes to creating objects in Java, developers often have to choose between using constructors or static factory methods. Both approaches have their advantages and disadvantages, and understanding when to use each can greatly impact the design and flexibility of your code. In this blog post, we will explore the differences between constructors and static factory methods, discuss their respective use cases, and provide insights into when to use one over the other.
Constructors
Constructors are special methods in Java that are used for initializing objects. They have the same name as the class and do not have a return type. Constructors are called implicitly when an object is created using the new
keyword.
public class Car {
private String make;
private String model;
// Constructor
public Car(String make, String model) {
this.make = make;
this.model = model;
}
}
Advantages of Constructors:
- Clarity: Constructors make it clear that a new instance of a class is being created.
- Immutable: Constructors can be used to create immutable objects by initializing all the required fields during object creation.
Disadvantages of Constructors:
- Inflexibility: If you need to change the logic of object creation or return different instances based on certain conditions, constructors might not be the best choice.
- No Descriptive Names: Constructors cannot have meaningful names to describe the object creation process.
Static Factory Methods
Static factory methods are public static methods that return an instance of the class. Unlike constructors, they have a name that can describe the purpose of creating the object.
public class Car {
private String make;
private String model;
// Static factory method
public static Car createCar(String make, String model) {
return new Car(make, model);
}
}
Advantages of Static Factory Methods:
- Descriptive Names: Static factory methods can have meaningful names that describe the object creation process, making the code more readable.
- Flexibility: They provide flexibility in object creation logic, allowing for caching, returning a subtype, or initializing objects based on certain conditions.
Disadvantages of Static Factory Methods:
- Hidden Object Creation: It may not be immediately clear that an object is being instantiated when calling a static factory method.
- Subclassing: Subclassing can be more complicated when using static factory methods, as they do not have direct access to the constructor of the superclass.
When to Use Constructors
Use constructors when:
- You want to clearly signal that a new instance of a class is being created.
- You need to ensure that all required fields are initialized during object creation.
- You don't anticipate the need to change the object creation logic in the future.
When to Use Static Factory Methods
Use static factory methods when:
- You need descriptive names for object creation that convey the intent of the method.
- You want flexibility in the object creation process, such as caching instances or returning a subtype.
- You want to hide the details of object creation from the caller.
To Wrap Things Up
In conclusion, the choice between constructors and static factory methods depends on the requirements and flexibility needed in object creation. Constructors are best suited for simple object creation without the need for complex logic, whereas static factory methods provide more flexibility and descriptive naming. Understanding the trade-offs involved in each approach is essential for writing clean, maintainable, and flexible Java code.
By carefully considering the pros and cons of both constructors and static factory methods, developers can make informed decisions about which approach best suits their specific use case, leading to more robust and adaptable codebases.
Remember, the primary goal is to write effective and maintainable code, so always choose the approach that best aligns with your project's requirements and future scalability.
For further insights on Java development and best practices, check out Oracle's Java documentation.