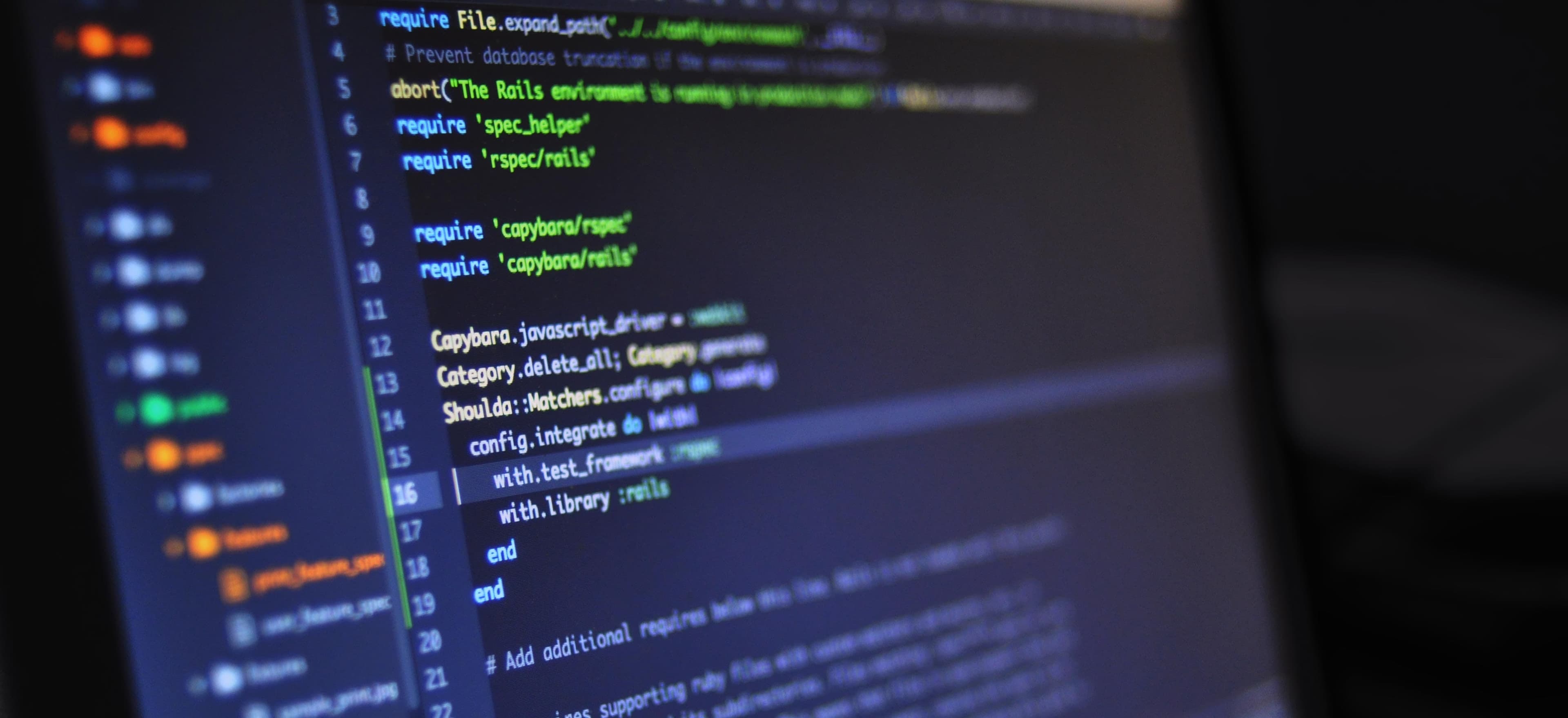
- Published on
Handling Data Changes in RecyclerView with Espresso Testing
RecyclerView is a powerful component in Android development for displaying large sets of data efficiently, but it comes with its own set of challenges when it comes to testing. One common scenario is testing how the RecyclerView behaves when its underlying data changes. This can happen when your app retrieves new data from a remote server, when the user performs a search, or when filtering the data based on certain criteria. In this blog post, we'll explore how to handle data changes in RecyclerView and how to write Espresso tests to ensure that the UI is updated correctly.
Understanding the Challenge
When the underlying data of a RecyclerView changes, the UI needs to be updated to reflect those changes. This can involve adding or removing items, updating the content of items, or reordering the items. Ensuring that the UI behaves as expected when the data changes is crucial for providing a seamless user experience.
Making Data Changes Observable
To handle data changes in RecyclerView, we need a way to observe these changes. One common approach is to use the Observer pattern. By making the underlying data observable, we can notify the RecyclerView adapter when changes occur, triggering the necessary UI updates.
Let's consider a scenario where we have a list of items displayed in a RecyclerView, and the user can add new items to the list. We can use LiveData from the Android Architecture Components to make the list of items observable. When a new item is added to the list, the RecyclerView adapter will be notified and the UI will be updated accordingly.
// Create a LiveData object to hold the list of items
LiveData<List<Item>> itemsLiveData = new MutableLiveData<>();
// Observe the LiveData in the fragment or activity
itemsLiveData.observe(this, items -> {
// Update the RecyclerView adapter with the new list of items
adapter.submitList(items);
});
In this example, the itemsLiveData
holds the list of items, and the observe
method is used to listen for changes to the list. When the list of items changes, the observer is notified and the RecyclerView adapter is updated with the new list of items, triggering the UI update.
Writing Espresso Tests for Data Changes
Now that we have made the data observable, we can write Espresso tests to ensure that the RecyclerView behaves as expected when the data changes. Let's consider a test scenario where we want to verify that the RecyclerView is updated correctly when a new item is added to the list.
@Test
public void testRecyclerViewDataChange() {
// Perform the action that adds a new item to the list
// ...
// Check if the RecyclerView is updated with the new item
onView(withId(R.id.recyclerView))
.check(withItemCount(expectedItemCount));
}
In this Espresso test, we first perform the action that adds a new item to the list, such as clicking a button or entering new data. Then, we use the check
method to verify that the RecyclerView is updated with the expected item count. This ensures that the UI is updated correctly when the underlying data changes.
Verifying Item Content Changes
In addition to adding or removing items, the content of individual items in the RecyclerView may also change. For example, the text or images displayed in the items may be updated based on user interactions or data from a remote server. To verify these content changes, we can write Espresso tests that target specific items in the RecyclerView and assert their content.
@Test
public void testRecyclerViewItemContentChange() {
// Perform the action that updates the content of a specific item
// ...
// Check if the content of the specific item is updated correctly
onView(withId(R.id.recyclerView))
.perform(actionOnItemAtPosition(0, click()));
onView(withId(R.id.itemTextView))
.check(matches(withText("Updated Content")));
}
In this Espresso test, we first perform the action that updates the content of a specific item, such as clicking on the item to view its details or updating it through user input. Then, we use the check
method to verify that the content of the specific item is updated correctly. This ensures that the UI reflects the changes in the underlying data.
Testing Item Removal
Another common scenario is testing the removal of items from the RecyclerView. This can happen when the user deletes an item or when the underlying data no longer includes a specific item. To test item removal, we can write Espresso tests that simulate the removal action and verify that the RecyclerView reflects the change.
@Test
public void testRecyclerViewItemRemoval() {
// Perform the action that removes a specific item from the list
// ...
// Check if the specific item is no longer displayed in the RecyclerView
onView(withId(R.id.recyclerView))
.check(withItemCount(expectedItemCount));
}
In this Espresso test, we first perform the action that removes a specific item from the list, such as swiping to delete or selecting a delete button. Then, we use the check
method to verify that the specific item is no longer displayed in the RecyclerView, ensuring that the UI reflects the removal of the item.
Closing Remarks
Handling data changes in RecyclerView is crucial for ensuring a smooth and responsive user interface. By making the underlying data observable and writing comprehensive Espresso tests, we can verify that the RecyclerView updates correctly when the data changes. This not only ensures the correctness of the UI but also enhances the reliability of the app. By incorporating these testing strategies into your development process, you can build robust and resilient RecyclerViews that can gracefully handle dynamic data changes.
In conclusion, managing data changes in RecyclerView and testing its behavior with Espresso is an essential aspect of Android app development. By effectively making data observable and devising comprehensive tests, you can ensure that your UI is updated correctly in response to data changes, providing a seamless user experience. Embracing these techniques will not only bolster the stability of your app but also streamline the development process.