How to Monitor Apache Kafka Consumer Lag
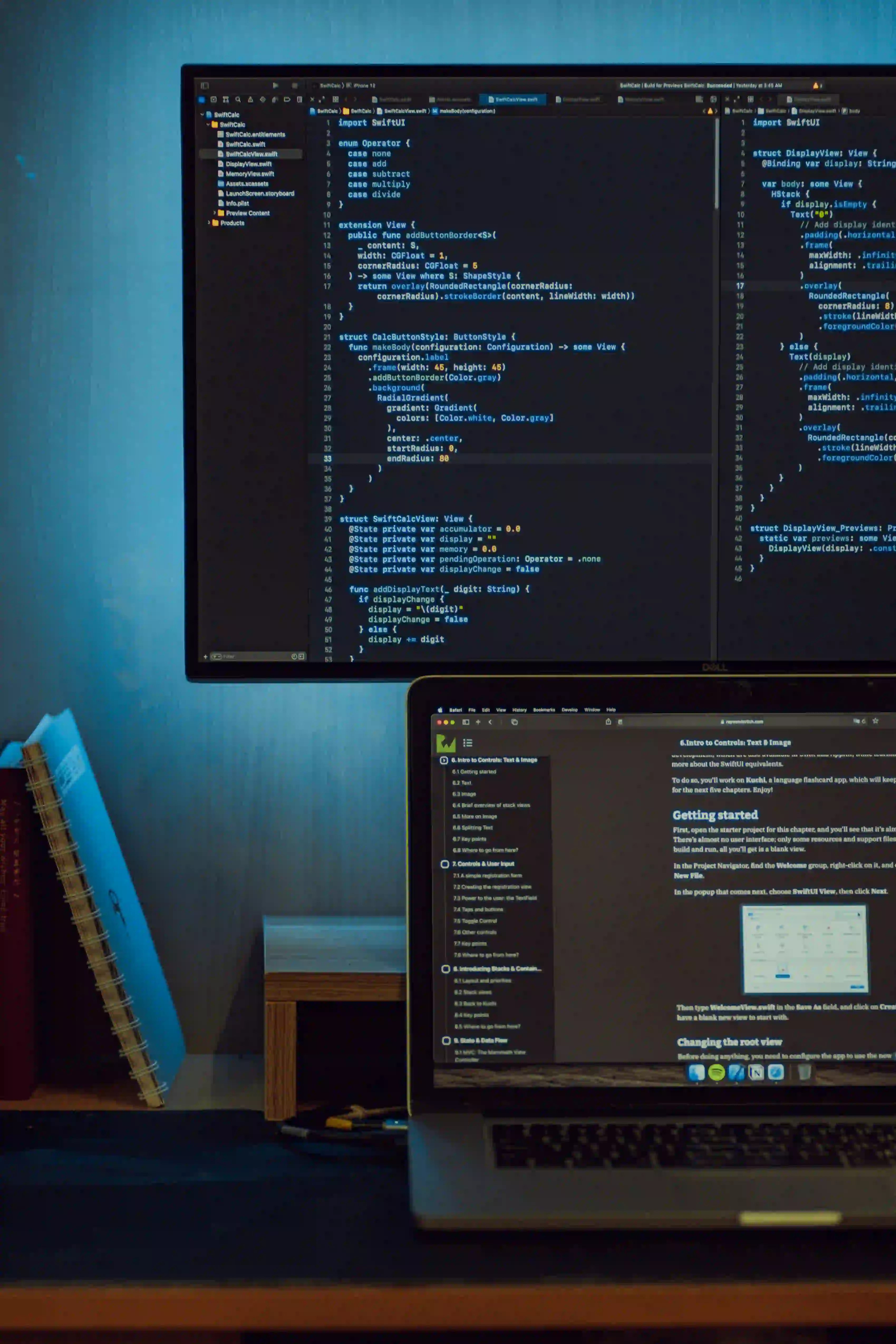
Monitoring Apache Kafka Consumer Lag Using Java
Apache Kafka is a popular distributed streaming platform that is widely used for building real-time data pipelines and streaming applications. In a Kafka cluster, it's essential to monitor the lag of consumers to ensure the efficient processing of messages. Consumer lag represents the delay in consuming messages from a Kafka topic and can indicate issues with consumer processing or cluster performance.
In this blog post, we will explore how to monitor Apache Kafka consumer lag using Java. We will leverage the Kafka consumer API and integrate it with a monitoring tool to track consumer lag metrics.
Setting Up the Kafka Consumer
Firstly, let's set up a Kafka consumer within a Java application. We will use the KafkaConsumer
class provided by the Apache Kafka client library to subscribe to a Kafka topic and start consuming messages.
Properties props = new Properties();
props.put("bootstrap.servers", "your-kafka-broker:9092");
props.put("group.id", "consumer-group-1");
props.put("enable.auto.commit", "true");
props.put("auto.commit.interval.ms", "1000");
props.put("key.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
props.put("value.deserializer", "org.apache.kafka.common.serialization.StringDeserializer");
KafkaConsumer<String, String> consumer = new KafkaConsumer<>(props);
consumer.subscribe(Collections.singletonList("your-topic"));
while (true) {
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, String> record : records) {
// Process the received message
}
}
Above, we've initialized a KafkaConsumer
with essential configurations such as the bootstrap servers, group ID, deserializers, and subscribed topics. The consumer then enters a loop to continuously poll for new records and process them.
Tracking Consumer Lag
To monitor the consumer lag, we need to keep track of the latest offset consumed by the consumer and the latest offset available in the Kafka topic. This information allows us to calculate the lag for each partition and eventually for the entire consumer.
Let's enhance our consumer code to include lag tracking.
Map<TopicPartition, Long> lastOffsetMap = new HashMap<>();
// Inside the loop for consuming messages
for (TopicPartition partition : records.partitions()) {
List<ConsumerRecord<String, String>> partitionRecords = records.records(partition);
long lastOffset = partitionRecords.get(partitionRecords.size() - 1).offset();
lastOffsetMap.put(partition, lastOffset);
}
// Calculate lag for each partition
Map<TopicPartition, Long> endOffsets = consumer.endOffsets(lastOffsetMap.keySet());
for (TopicPartition partition : lastOffsetMap.keySet()) {
long endOffset = endOffsets.get(partition);
long currentOffset = lastOffsetMap.get(partition);
long lag = endOffset - currentOffset;
System.out.println("Consumer lag for partition " + partition.partition() + ": " + lag);
}
In the above code, we maintain a map lastOffsetMap
to store the latest offsets consumed for each partition within the consumed records. After consuming messages, we retrieve the end offsets for these partitions using consumer.endOffsets()
and calculate the lag.
Integrating with Monitoring Tools
Now that we can calculate the consumer lag within our Java application, the next step is to integrate this information with a monitoring tool or system for visualization and alerting.
We can employ various monitoring solutions such as Prometheus, Grafana, or InfluxDB to aggregate and visualize consumer lag metrics. One common approach is to expose these metrics through an HTTP endpoint using a library like Micrometer or Dropwizard Metrics.
Let's see how we can use Micrometer to expose consumer lag metrics as custom metrics for Prometheus.
import io.micrometer.core.instrument.MeterRegistry;
import io.micrometer.core.instrument.Tag;
import io.micrometer.core.instrument.binder.MeterBinder;
import io.micrometer.core.instrument.simple.SimpleMeterRegistry;
public class ConsumerLagMetrics implements MeterBinder {
private final KafkaConsumer<String, String> consumer;
public ConsumerLagMetrics(KafkaConsumer<String, String> consumer) {
this.consumer = consumer;
}
@Override
public void bindTo(MeterRegistry registry) {
registry.gauge("kafka.consumer.lag",
Tags.of("group", "consumer-group-1"),
consumer,
c -> {
// Calculate and return the overall consumer lag
return calculateOverallLag(consumer);
}
);
}
private double calculateOverallLag(KafkaConsumer<String, String> consumer) {
// Logic to calculate overall lag across all partitions
}
}
// Create and register the metrics binder
MeterRegistry meterRegistry = new SimpleMeterRegistry();
ConsumerLagMetrics consumerLagMetrics = new ConsumerLagMetrics(consumer);
consumerLagMetrics.bindTo(meterRegistry);
In the above code, we implemented a custom MeterBinder
using Micrometer to create a gauge metric for Kafka consumer lag. This metric is registered in a MeterRegistry
, which could be configured to integrate with Prometheus or other monitoring systems.
Lessons Learned
In this blog post, we've outlined the steps to monitor Apache Kafka consumer lag using Java. We started by setting up a Kafka consumer and then extended it to track consumer lag. Furthermore, we explored how to integrate the lag metrics with monitoring tools using Micrometer.
Monitoring consumer lag is crucial for maintaining the health and performance of Kafka consumers in a distributed system. By leveraging Java and appropriate monitoring tools, organizations can ensure the efficient processing of messages within their Kafka clusters.
For further reading on Apache Kafka and Java integration, check out the official documentation and confluent.io.
Happy monitoring!