Improving User Experience for Read-Only Access
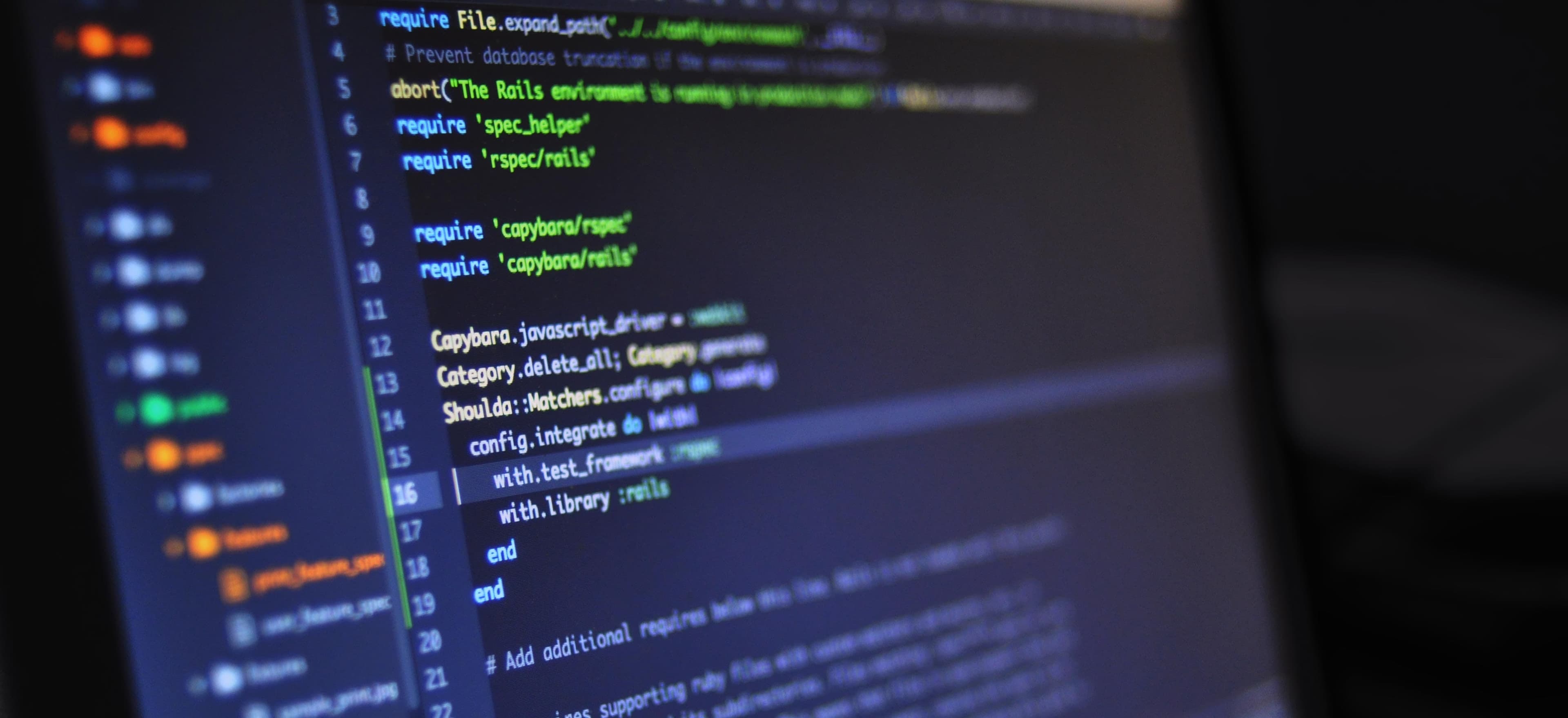
- Published on
How to Enhance User Experience for Read-Only Access in Java
As a Java developer, you may encounter situations where you need to provide read-only access to data for users. It's important to ensure that the user experience for read-only access is seamless and intuitive. In this article, we'll explore some strategies for enhancing the user experience in such scenarios using Java.
Understanding the Importance of User Experience for Read-Only Access
When users have read-only access, it means they can view the data but cannot modify it. This often occurs in scenarios where the data is sensitive or when users have limited permissions. Despite the restricted nature of read-only access, it's crucial to prioritize the user experience. A well-designed read-only interface can lead to increased user satisfaction and productivity.
Leveraging Java for Improving User Experience
Java offers a robust ecosystem for building applications with a focus on user experience. By leveraging Java, you can implement various features and design patterns to enhance the user interface for read-only access.
Implementing a Clear and Intuitive User Interface
When designing the user interface for read-only access, clarity and intuitiveness should be the primary goals. Use descriptive labels, organized layouts, and meaningful visual cues to help users understand the data they are viewing. In Java, you can achieve this by leveraging UI frameworks such as JavaFX or Swing.
// Example of using JavaFX to create a clear and intuitive UI for read-only access
Label nameLabel = new Label("Name:");
TextField nameField = new TextField();
nameField.setDisable(true); // Make the field read-only
In this example, we use JavaFX to create a label and a disabled text field, clearly indicating that the user can only view the name without being able to modify it. This approach enhances the user experience by providing a visually distinct display for read-only data.
Providing Contextual Help and Guidance
Even in read-only scenarios, users may benefit from contextual help and guidance. Incorporate tooltips, on-screen guidance, or informational pop-ups to provide additional insights about the data being viewed. In Java, you can easily implement this using various UI components and event listeners.
// Example of adding a tooltip to provide contextual help
Button infoButton = new Button("ℹ");
Tooltip tooltip = new Tooltip("This field is read-only");
Tooltip.install(infoButton, tooltip); // Attach the tooltip to the button
By attaching a tooltip to a button or any relevant UI component, you can offer users contextual help regarding the read-only nature of the data, thus improving their overall experience.
Handling User Interactions Gracefully
Even though users have read-only access, they may still attempt to interact with the interface. It's essential to handle such interactions gracefully by providing informative feedback and preventing unintended actions. In Java, you can achieve this by strategically managing event handling and input validation.
// Example of preventing unintended user input in a read-only field
nameField.setOnKeyTyped(event -> event.consume()); // Prevent user input through key typing
By consuming the key-typed event on a read-only field, you ensure that users cannot inadvertently modify the data, thereby enhancing the overall user experience.
Optimizing Performance for Read-Only Operations
In read-only scenarios, optimizing performance is crucial for delivering a responsive user experience. Utilize efficient data retrieval techniques, caching mechanisms, and asynchronous processing to minimize latency and enhance the responsiveness of the read-only interface. In Java, libraries such as Ehcache and CompletableFuture can be valuable for optimizing performance in read-only operations.
// Example of using CompletableFuture for asynchronous data retrieval in a read-only context
CompletableFuture<List<Data>> fetchData = CompletableFuture.supplyAsync(() -> dataService.getReadOnlyData());
fetchData.thenAccept(data -> {
// Update UI with retrieved data
});
By leveraging CompletableFuture to asynchronously retrieve read-only data, you ensure that the user interface remains responsive while data is being fetched, ultimately improving the overall user experience.
A Final Look
Enhancing the user experience for read-only access in Java involves designing a clear and intuitive interface, providing contextual help and guidance, handling user interactions gracefully, and optimizing performance for read-only operations. By prioritizing user experience even in read-only scenarios, you can significantly improve user satisfaction and the overall usability of your Java applications.
By implementing these strategies, you can elevate the user experience in read-only access scenarios, contributing to the success of your Java applications.
For further information about optimizing Java applications for improved user experience, you can refer to the official documentation and relevant resources within the Java community.
Remember, prioritizing the user experience, even in read-only scenarios, can make a significant impact on the overall success of your Java applications.