Refactoring Test Code for Fluent Assertion Pattern
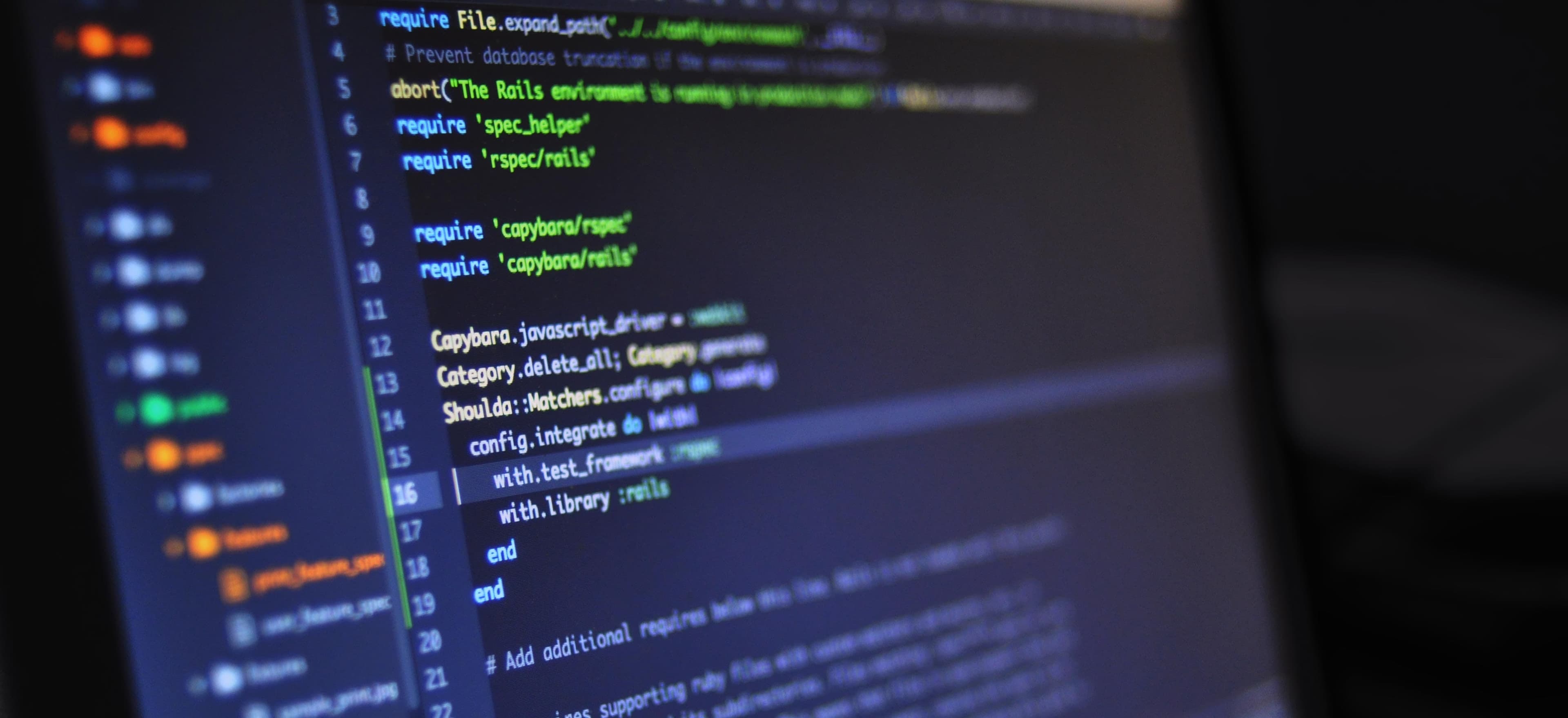
- Published on
Refactoring Test Code for Fluent Assertion Pattern
When writing unit tests in Java, it's crucial to ensure that the test code is not only effective in catching bugs but also remains maintainable and easy to understand. One technique that can significantly improve the readability and robustness of test code is the use of fluent assertion patterns.
In this blog post, we'll explore the concept of fluent assertion patterns and demonstrate how to refactor existing test code to incorporate this approach. We'll be using the popular testing framework JUnit along with the Fluent Assertions library to showcase examples of refactoring.
Understanding Fluent Assertion Patterns
Fluent assertion patterns, also known as fluent assertions, involve expressing assertions in a way that reads like natural language. This allows for more descriptive and readable test code, making it easier to understand the expected behavior and outcomes.
Consider the following example using traditional JUnit assertions:
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 2);
assertEquals(4, result);
}
While this test effectively verifies the addition operation, the assertion statement assertEquals(4, result)
might not be immediately clear to someone reading the test for the first time. This is where fluent assertion patterns come into play.
Refactoring with Fluent Assertions
Let's refactor the previous test using fluent assertions provided by the AssertJ library, a popular choice for fluent assertions in Java:
import static org.assertj.core.api.Assertions.assertThat;
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 2);
assertThat(result).isEqualTo(4);
}
In this refactored test, we've replaced the JUnit assertEquals
with AssertJ's assertThat
and isEqualTo
, resulting in a more readable and natural language-like assertion. This improvement can make a significant difference, especially in more complex test scenarios.
Benefits of Fluent Assertions
Fluent assertions offer several benefits:
Readability
Using fluent assertions can enhance the readability of test code, making it clearer and more expressive. This is particularly valuable when working in a team where different members need to understand and maintain the test suite.
Chaining and Composition
Fluent assertions often support chaining and composition, allowing multiple assertions to be combined in a fluid, readable manner. This can lead to more concise and focused test cases.
Error Messages
When assertions fail, fluent assertion libraries often provide detailed and contextual error messages, aiding in the diagnosis and resolution of issues.
Refactoring Existing Test Code
Now, let's consider a more complex example where we have an existing test with multiple assertions:
@Test
public void testEmployeeDetails() {
Employee employee = new Employee("John Doe", 30, "Engineering");
assertEquals("John Doe", employee.getName());
assertEquals(30, employee.getAge());
assertEquals("Engineering", employee.getDepartment());
}
Refactoring this test using fluent assertions can lead to more concise and readable code:
import static org.assertj.core.api.Assertions.assertThat;
@Test
public void testEmployeeDetails() {
Employee employee = new Employee("John Doe", 30, "Engineering");
assertThat(employee.getName()).isEqualTo("John Doe");
assertThat(employee.getAge()).isEqualTo(30);
assertThat(employee.getDepartment()).isEqualTo("Engineering");
}
By leveraging fluent assertions, the test code becomes more expressive and conveys the expected outcomes in a natural language style.
Closing Remarks
Incorporating fluent assertion patterns into test code can greatly enhance the readability, maintainability, and effectiveness of unit tests. While this blog post has focused on the AssertJ library, other libraries such as Hamcrest and Truth also provide similar capabilities for fluent assertions in Java.
By refactoring existing test code to embrace fluent assertions, developers can create test suites that not only serve as effective quality gates but also act as living documentation of the expected behavior of the code under test.
Start refactoring your test code with fluent assertions and experience the benefits firsthand in your Java projects.
Happy testing!