Managing Dependencies: A Guide for Package-Module Conversion
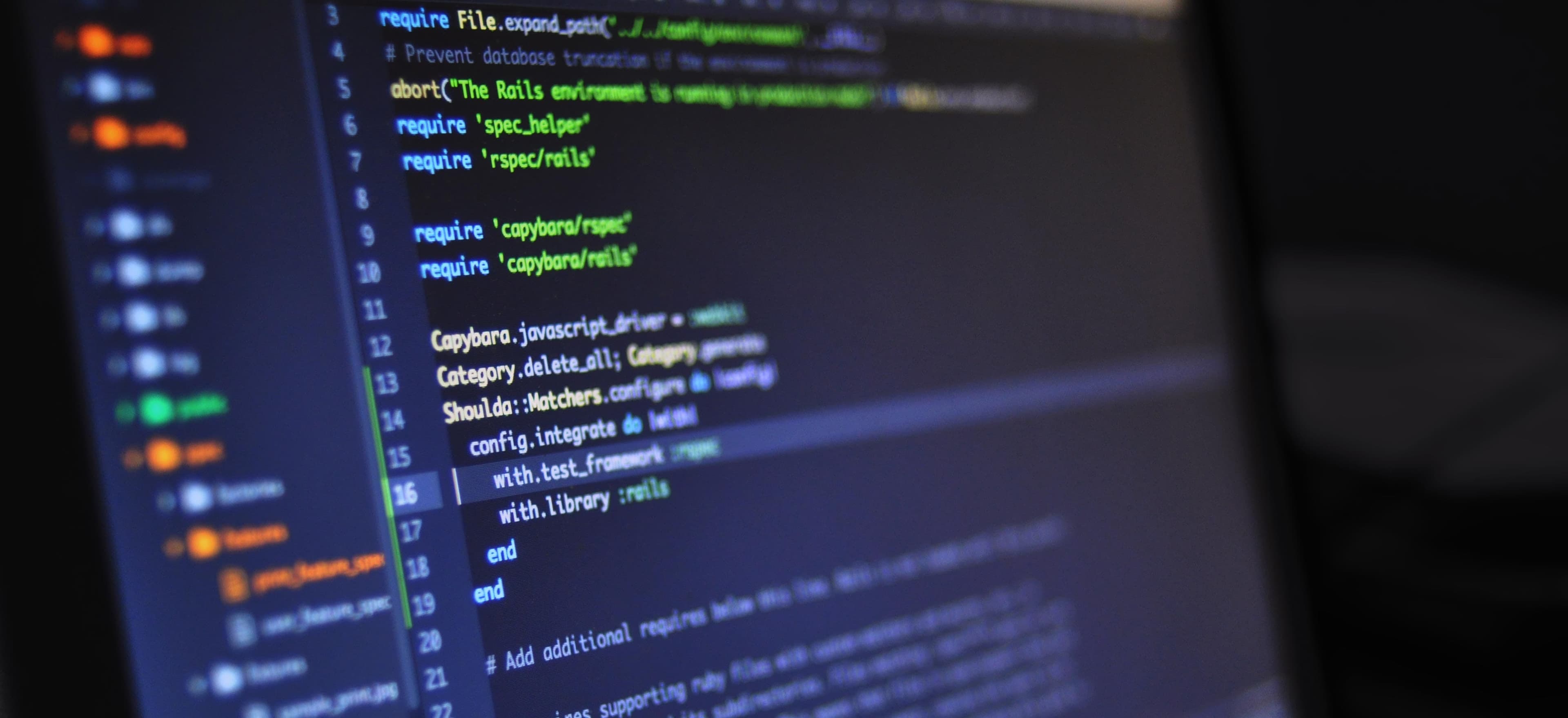
- Published on
Managing Dependencies: A Guide for Package-Module Conversion
As software development progresses, managing dependencies becomes a critical aspect of maintaining a stable and efficient codebase. In this guide, we will delve into the essential concepts of managing dependencies, focusing on the transition from traditional packages to modules in Java. We will explore the benefits of module systems, the process of converting packages to modules, and best practices for dependency management in a modular Java application.
Understanding Dependencies in Java
In Java, dependencies refer to external libraries or modules that a project relies on. Traditionally, Java applications have used the import
statement to access classes and packages from external libraries. However, with the introduction of modules in Java 9, a more robust and encapsulated system for managing dependencies was introduced.
Benefits of Module Systems
The Java Platform Module System (JPMS) brings several advantages over the traditional classpath, including:
- Encapsulation: Modules allow you to explicitly specify which packages are accessible to other modules, reducing the risk of unwanted dependencies and improving encapsulation.
- Dependency Management: Modules provide a clear definition of dependencies, making it easier to understand and manage the libraries used in an application.
- Improved Security: Modules enhance the security of your application by controlling the visibility of internal APIs.
- Performance: The module system can optimize the loading and resolution of modules, potentially improving the startup time and overall performance of the application.
Converting Packages to Modules
The process of converting a package-based project to a modular one involves several key steps:
1. Module Declaration
To convert a package to a module, you need to create a module-info.java
file at the root of the package. This file declares the module and specifies its dependencies, as well as the packages it exports.
module com.example.myapp {
requires transitive org.slf4j;
exports com.example.myapp.api;
}
In this example, the module
declaration specifies the module name, requires
specifies its dependencies, and exports
specifies the packages that are accessible to other modules.
2. Resolving Dependencies
Once the module declaration is in place, you need to ensure that the dependencies are available to the module system. This typically involves using a build tool like Maven or Gradle to manage dependencies and ensure they are included in the module path.
3. Accessibility and Encapsulation
Review the accessibility of classes and packages within the module. Ensure that only the necessary packages are exported, while keeping others encapsulated within the module.
4. Testing and Refactoring
Thoroughly test the modularized code to ensure that it functions as expected. Refactor the code as needed to align with the modular structure.
Best Practices for Dependency Management in Modular Java Applications
1. Clearly Define Module Boundaries
When creating modules, it’s important to clearly define their boundaries and dependencies. Avoid exposing internal packages unless necessary, and only let essential packages be accessible to other modules.
2. Leverage Modular JDK and Third-Party Libraries
Utilize the modular structure of the JDK and third-party libraries to benefit from their encapsulation and optimized dependencies.
3. Maintain Compatibility with Non-Modularized Code
If your application interfaces with non-modularized code, ensure compatibility and interoperability by carefully managing dependencies and access control.
4. Use Services for Loose Coupling
Employ the service provider interface mechanism to achieve loose coupling between modules, allowing for extensibility and plug-and-play functionality.
To Wrap Things Up
Managing dependencies in a modular Java application involves a thoughtful approach to module declaration, dependency resolution, accessibility, and best practices for maintaining a modular codebase. By transitioning from traditional packages to modules, developers can take advantage of improved encapsulation, dependency management, and security. Embracing the principles of the Java Platform Module System enables developers to build more maintainable, scalable, and secure applications in the Java ecosystem.
For further reading, check out the official Java Platform Module System documentation for a comprehensive understanding of modules in Java.
Through strategic dependency management and module conversion, Java developers can streamline their projects, enhance code maintainability, and ensure a robust foundation for future development.