Troubleshooting Image Upload Issues in Dropbox
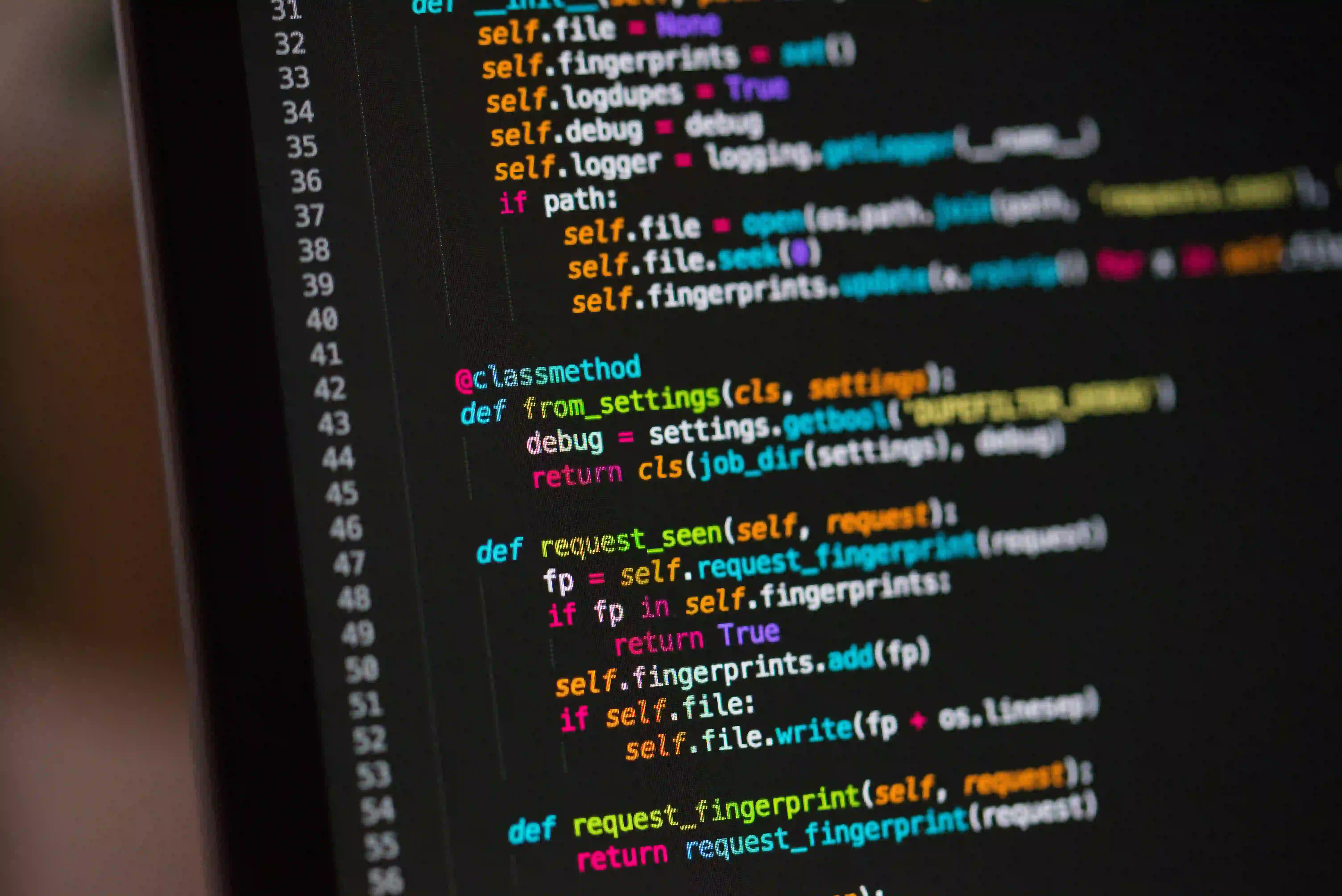
Understanding the issue
So, you are trying to upload images to Dropbox through your Java application, but for some reason, it's not working as expected. Before delving into the code, let's first understand why this issue might be occurring.
Common issues
- Authentication: Incorrect authentication credentials could prevent the upload.
- File Path: The file path might be incorrect, causing the upload to fail.
- File Size: Large file sizes can sometimes lead to upload failures.
- Network Issues: Unstable network connections can interrupt the upload process.
Now that we have a general understanding of the potential causes, let's explore how to troubleshoot and fix these issues in a Java application.
Authenticating with Dropbox API
To interact with Dropbox in a Java application, you will need to use the Dropbox API. The first step is to authenticate and obtain the necessary access token for making API requests.
DbxRequestConfig config = new DbxRequestConfig("your-app-name");
DbxClientV2 client = new DbxClientV2(config, "your-access-token");
Here, your-app-name
should be replaced with your actual app name, and your-access-token
should be replaced with the access token obtained after registering your app with Dropbox.
Uploading an Image to Dropbox
Now, let's take a look at how to upload an image to Dropbox using the Dropbox API in a Java application.
File inputFile = new File("/path/to/local/image.jpg");
try (InputStream in = new FileInputStream(inputFile)) {
FileMetadata metadata = client.files().uploadBuilder("/image.jpg")
.uploadAndFinish(in);
}
In this snippet, we create a File
object representing the local image file and then use a FileInputStream
to read the file's contents. We then use the uploadBuilder
method to initiate the upload process to a specific path on Dropbox.
Troubleshooting the Upload Issues
Now, let's address the potential issues that could be causing the image upload problems and implement solutions.
1. Authentication Issues
If the authentication is failing, make sure that you have the correct access token and that your app has the necessary permissions to upload files. You can regenerate the access token from the Dropbox developer console.
DbxRequestConfig config = new DbxRequestConfig("your-app-name");
DbxClientV2 client = new DbxClientV2(config, "new-access-token");
2. File Path Errors
If the file path is incorrect, the upload will fail. Verify that the file path is accurate and properly formatted.
File inputFile = new File("/correct/path/to/local/image.jpg");
3. Handling Large File Sizes
Large file sizes can lead to upload failures, especially if there are network issues. Consider implementing chunked uploads for larger files to handle interruptions and resume uploads if they fail.
File inputFile = new File("/path/to/large/image.jpg");
long size = inputFile.length();
long chunkSize = 1L << 20; // 1 MB chunks
try (InputStream in = new FileInputStream(inputFile)) {
UploadSessionCursor cursor = new UploadSessionCursor(" ");
FileMetadata metadata;
if (size > chunkSize) {
UploadSessionStartUploader uploader = client.files().uploadSessionStart(false);
byte[] chunk = new byte[(int) chunkSize];
int bytesRead = in.read(chunk);
UploadSessionAppendV2Result appendResult = client.files().uploadSessionAppendV2(cursor, bytesRead, new ByteArrayInputStream(chunk));
cursor.setOffset(cursor.getOffset() + bytesRead);
while ((bytesRead = in.read(chunk)) != -1) {
if (size - cursor.getOffset() < chunkSize) {
chunk = Arrays.copyOf(chunk, (int) (size - cursor.getOffset()));
}
client.files().uploadSessionAppendV2(cursor, chunk.length, new ByteArrayInputStream(chunk));
cursor.setOffset(cursor.getOffset() + bytesRead);
}
metadata = client.files().uploadSessionFinish(cursor, new CommitInfo("/large-image.jpg"), new ByteArrayInputStream(chunk));
} else {
metadata = client.files().uploadBuilder("/large-image.jpg").uploadAndFinish(in);
}
}
4. Network Issues
Handle network issues gracefully by implementing retry logic with exponential backoff. This can be done using libraries like okhttp
for making HTTP requests to the Dropbox API.
RetryPolicy retryPolicy = new ExponentialRetry(5, Duration.ofSeconds(1), Duration.ofSeconds(5), Duration.ofMinutes(1));
OkHttpClient client = OkHttpClients.createDefault("your-app-name", retryPolicy);
InputStream in = new FileInputStream(inputFile);
RequestBody requestBody = RequestBody.create(MediaType.parse("image/jpeg"), toByteArray(in));
Request request = new Request.Builder()
.url("https://content.dropboxapi.com/2/files/upload")
.addHeader("Authorization", "Bearer your-access-token")
.addHeader("Dropbox-API-Arg", "{\"path\": \"/image.jpg\"}")
.addHeader("Content-Type", "application/octet-stream")
.post(requestBody)
.build();
Response response = client.newCall(request).execute();
Lessons Learned
By carefully considering authentication, file path accuracy, handling large file sizes, and managing network issues, you can troubleshoot and resolve image upload problems in a Dropbox Java application. Remember to leverage appropriate error handling and logging to diagnose and resolve any unforeseen issues.
With these solutions in place, you can ensure a robust and reliable image uploading process in your Java application, providing a seamless experience for your users.
For further reading, you can refer to the Dropbox Java SDK documentation.