Implementing Real-time Chat Functionality
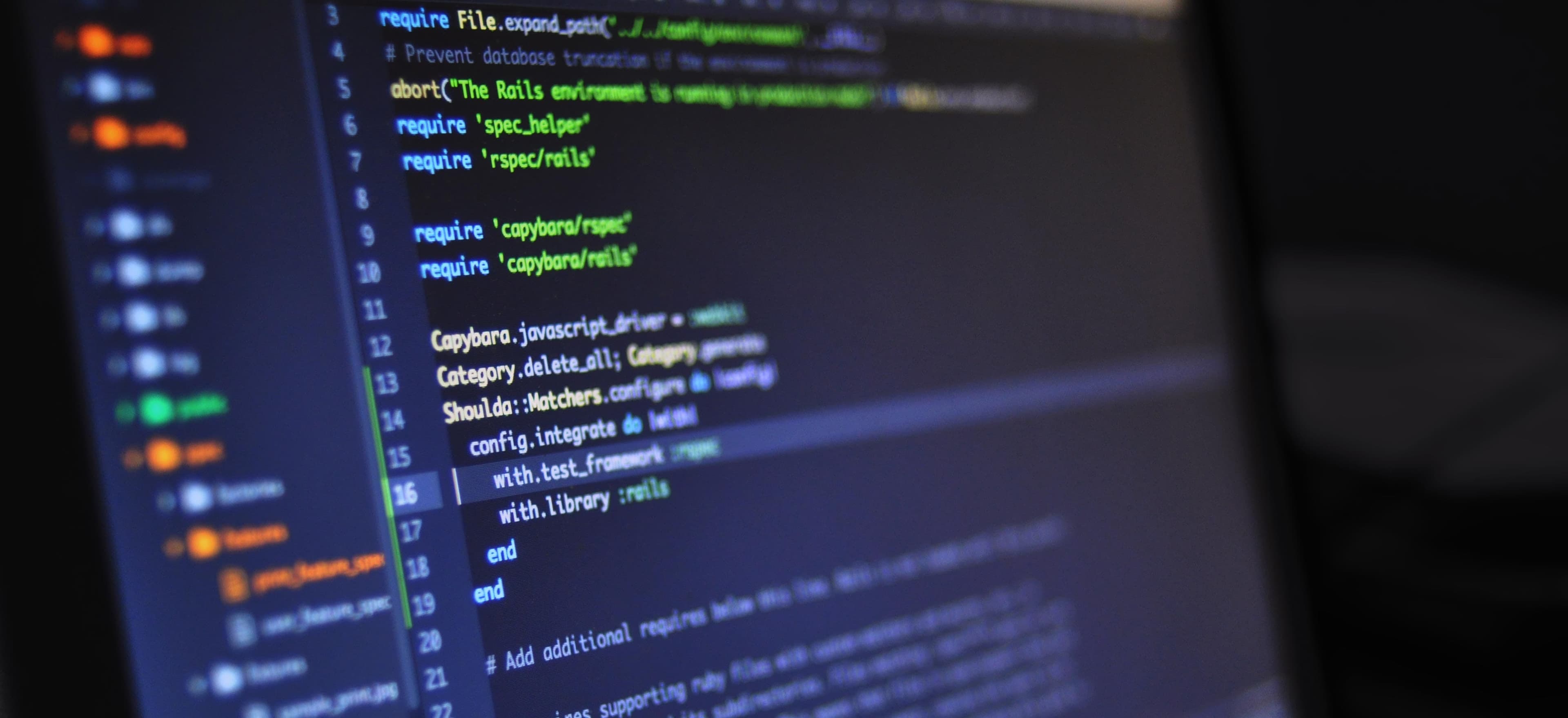
- Published on
Implementing Real-time Chat Functionality in Java
Real-time chat functionality is a crucial aspect of many modern applications, enabling users to communicate with each other instantly. In this blog post, we will explore how to implement real-time chat functionality in Java using the WebSocket protocol. WebSocket provides a full-duplex communication channel over a single TCP connection, making it ideal for building real-time chat applications.
Getting Started with WebSocket in Java
To implement real-time chat functionality in Java, we can leverage the javax.websocket API, which provides support for creating WebSocket endpoints.
@ServerEndpoint(value = "/chat")
public class ChatEndpoint {
@OnOpen
public void onOpen(Session session) {
// Logic for handling new chat session
}
@OnMessage
public void onMessage(String message, Session session) {
// Logic for handling incoming messages
}
@OnClose
public void onClose(Session session) {
// Logic for handling chat session closure
}
}
In the above code snippet, we define a WebSocket endpoint for handling chat functionality. The @ServerEndpoint
annotation specifies the URI at which the endpoint will be available, and the ChatEndpoint
class contains methods annotated with @OnOpen
, @OnMessage
, and @OnClose
to handle the respective WebSocket lifecycle events.
Integrating WebSocket with Frontend
To enable real-time chat in a web application, we need to establish a WebSocket connection from the frontend using JavaScript. Let's take a look at a simple example of how this can be achieved using the WebSocket
API.
const socket = new WebSocket('ws://localhost:8080/chat');
socket.onopen = () => {
console.log('WebSocket connection established');
};
socket.onmessage = (event) => {
const message = event.data;
// Logic for handling incoming messages
};
socket.onclose = () => {
console.log('WebSocket connection closed');
};
In the above JavaScript code, we create a new WebSocket
object and specify the URI of the WebSocket endpoint. We then define event handlers for the onopen
, onmessage
, and onclose
events to handle the WebSocket lifecycle and incoming messages.
Broadcasting Messages to Connected Clients
In a real-time chat application, it's essential to broadcast messages to all connected clients. We can achieve this by maintaining a collection of active sessions and iterating through them to send messages.
@ServerEndpoint(value = "/chat")
public class ChatEndpoint {
private static Set<Session> sessions = Collections.synchronizedSet(new HashSet<>());
@OnOpen
public void onOpen(Session session) {
sessions.add(session);
}
@OnMessage
public void onMessage(String message, Session senderSession) {
for (Session session : sessions) {
if (session != senderSession) {
session.getAsyncRemote().sendText(message);
}
}
}
@OnClose
public void onClose(Session session) {
sessions.remove(session);
}
}
In the updated ChatEndpoint
class, we maintain a synchronized set of active Session
objects representing connected clients. When a message is received, we iterate through the sessions and send the message to all clients except the one that sent the message.
Handling User Authentication and Authorization
In a production environment, it's crucial to implement user authentication and authorization to secure the chat application. This can be achieved by integrating WebSocket endpoints with a security framework such as Spring Security.
We can use Spring Security to enforce authentication and authorization rules for WebSocket endpoints, ensuring that only authenticated users can connect and send messages.
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfig implements WebSocketMessageBrokerConfigurer {
@Override
public void registerStompEndpoints(StompEndpointRegistry registry) {
registry.addEndpoint("/chat").setAllowedOrigins("*").withSockJS();
}
@Override
public void configureClientInboundChannel(ChannelRegistration registration) {
registration.interceptors(new AuthChannelInterceptorAdapter());
}
}
In the above example, we configure WebSocket endpoints using Spring WebSockets and apply a custom AuthChannelInterceptorAdapter
to enforce authentication and authorization rules for incoming messages.
Key Takeaways
In this blog post, we explored how to implement real-time chat functionality in Java using WebSocket. We learned how to create a WebSocket endpoint, integrate it with a frontend application, broadcast messages to connected clients, and handle user authentication and authorization.
By following these best practices and leveraging the power of WebSocket, developers can create robust and secure real-time chat applications in Java.
Implementing real-time chat functionality is a valuable skill for Java developers, enabling them to build interactive and collaborative applications that meet the demands of modern users.
To delve deeper into the topic of WebSocket in Java or understand the integration with frameworks like Spring Security, it is recommended to refer to this guide on WebSocket in Java.
Feel free to experiment with the code snippets provided and incorporate real-time chat functionality into your Java applications!