Handling JSON Requests and Responses in Spring MVC
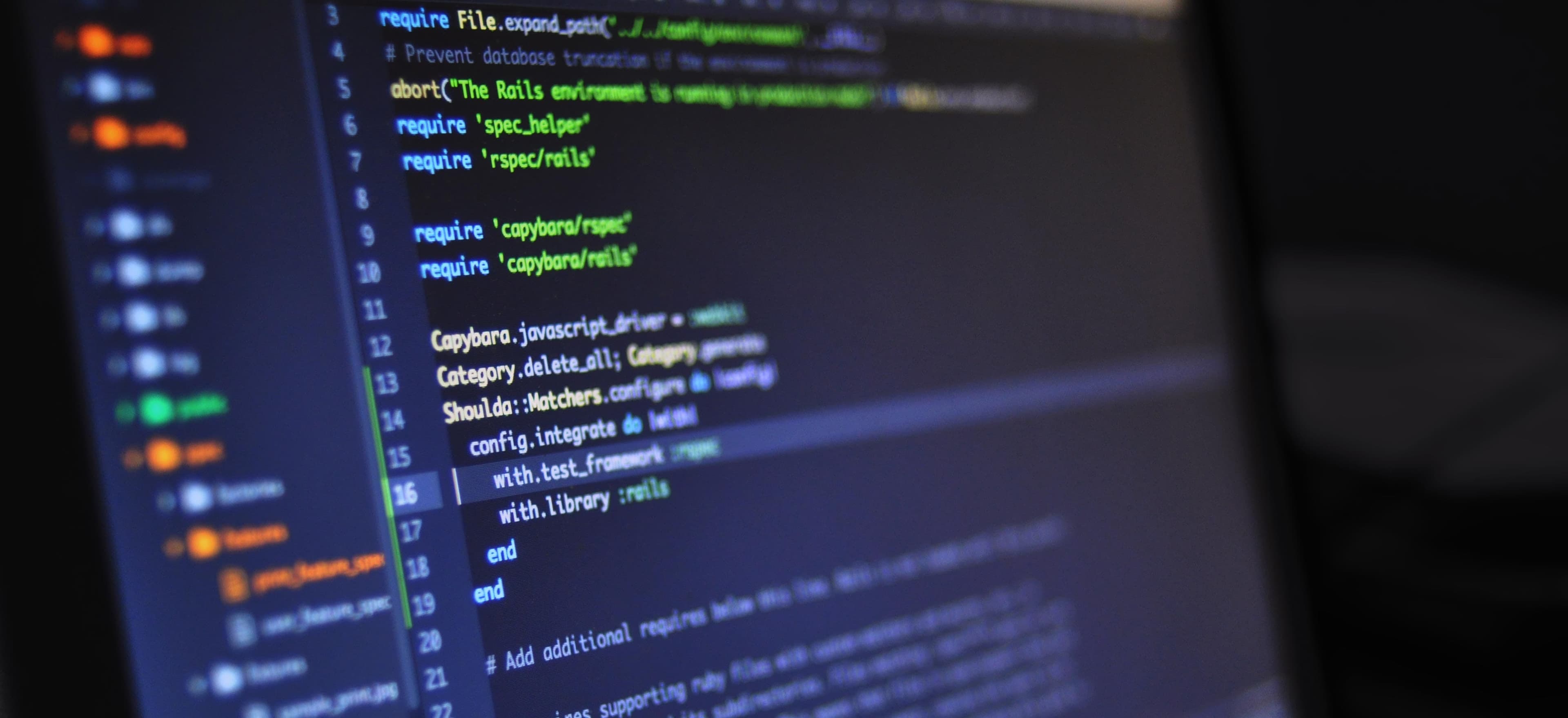
- Published on
Handling JSON Requests and Responses in Spring MVC
The Opening Bytes
In modern web development, handling JSON (JavaScript Object Notation) has become a fundamental part of building RESTful APIs. When working with Java, Spring MVC provides easy and powerful tools to handle JSON requests and responses. In this blog post, we'll explore how to handle JSON requests and responses in Spring MVC, covering topics such as serialization, deserialization, and error handling.
Setting Up the Project
Before diving into the specifics of handling JSON in Spring MVC, let's set up a basic Spring MVC project. If you're not familiar with the process of setting up a Spring MVC project, you can follow the steps outlined in the official Spring Framework documentation.
Handling JSON Requests
When dealing with JSON requests, Spring MVC provides the @RequestBody
annotation to automatically deserialize the JSON payload into a Java object. Let's consider an example where we want to handle a JSON request containing user information.
Example 1: Handling a JSON Request
@RestController
@RequestMapping("/users")
public class UserController {
@PostMapping
public ResponseEntity<String> createUser(@RequestBody User user) {
// Process the user object
return ResponseEntity.ok("User created successfully");
}
}
In this example, the @PostMapping
annotation signifies that the createUser
method handles POST requests. The @RequestBody
annotation tells Spring MVC to deserialize the JSON payload into the User
object.
Why It Matters
Using the @RequestBody
annotation allows for seamless integration of JSON requests in Spring MVC, providing a clean and concise way to work with incoming JSON data.
Handling JSON Responses
Similarly, when returning JSON responses from Spring MVC controllers, we can use the @ResponseBody
annotation to automatically serialize the response object into JSON. Let's explore this with an example where we return user information as JSON.
Example 2: Handling a JSON Response
@RestController
@RequestMapping("/users")
public class UserController {
@GetMapping("/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
User user = // Retrieve user from database
return ResponseEntity.ok(user);
}
}
In this example, the getUserById
method returns a ResponseEntity
containing the user object. Spring MVC automatically serializes the User
object into JSON due to the @ResponseBody
annotation on the method.
Why It Matters
By using the @ResponseBody
annotation, we simplify the process of returning JSON responses, enhancing the readability and maintainability of our code.
Error Handling
Handling errors when working with JSON in Spring MVC is crucial for building robust APIs. Spring MVC provides ways to handle error responses in a structured and consistent manner. Let's consider an example where we handle a custom exception and return an error response as JSON.
Example 3: Handling JSON Error Response
@RestControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<String> handleUserNotFoundException(UserNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage());
}
}
In this example, the GlobalExceptionHandler
class uses the @RestControllerAdvice
annotation to globally handle exceptions. The @ExceptionHandler
annotation is used to handle the UserNotFoundException
and return a JSON response with an appropriate HTTP status code.
Why It Matters
Proper error handling ensures that clients receive meaningful error messages in JSON format, contributing to a better user experience and easier debugging.
Customizing JSON Serialization and Deserialization
While Spring MVC's default JSON serialization and deserialization work well for most cases, there are scenarios where customization is necessary. This can include handling date formats, ignoring certain fields, or applying specific naming conventions. In such cases, we can use Jackson annotations to customize the JSON mapping behavior.
Example 4: Customizing JSON Serialization
public class User {
@JsonFormat(shape = JsonFormat.Shape.STRING, pattern = "yyyy-MM-dd")
private Date birthDate;
// Getter and setter methods
}
In this example, the @JsonFormat
annotation from the Jackson library is used to specify the date format for the birthDate
field. This ensures that the birthDate
is serialized into the specified date format in the JSON response.
Why It Matters
Customizing JSON serialization and deserialization allows us to tailor the JSON representation of our objects to specific requirements, ensuring compatibility with client-side expectations.
To Wrap Things Up
In this blog post, we've explored the key aspects of handling JSON requests and responses in Spring MVC. We discussed how to effortlessly handle JSON requests using the @RequestBody
annotation, return JSON responses with the @ResponseBody
annotation, handle errors in a structured manner, and customize JSON serialization and deserialization. With these tools and techniques at your disposal, you can effectively work with JSON in Spring MVC, building robust and efficient RESTful APIs.
If you found this blog post helpful, feel free to share it with your peers and colleagues.
Happy coding!