Integrating Jython with Java Codebase
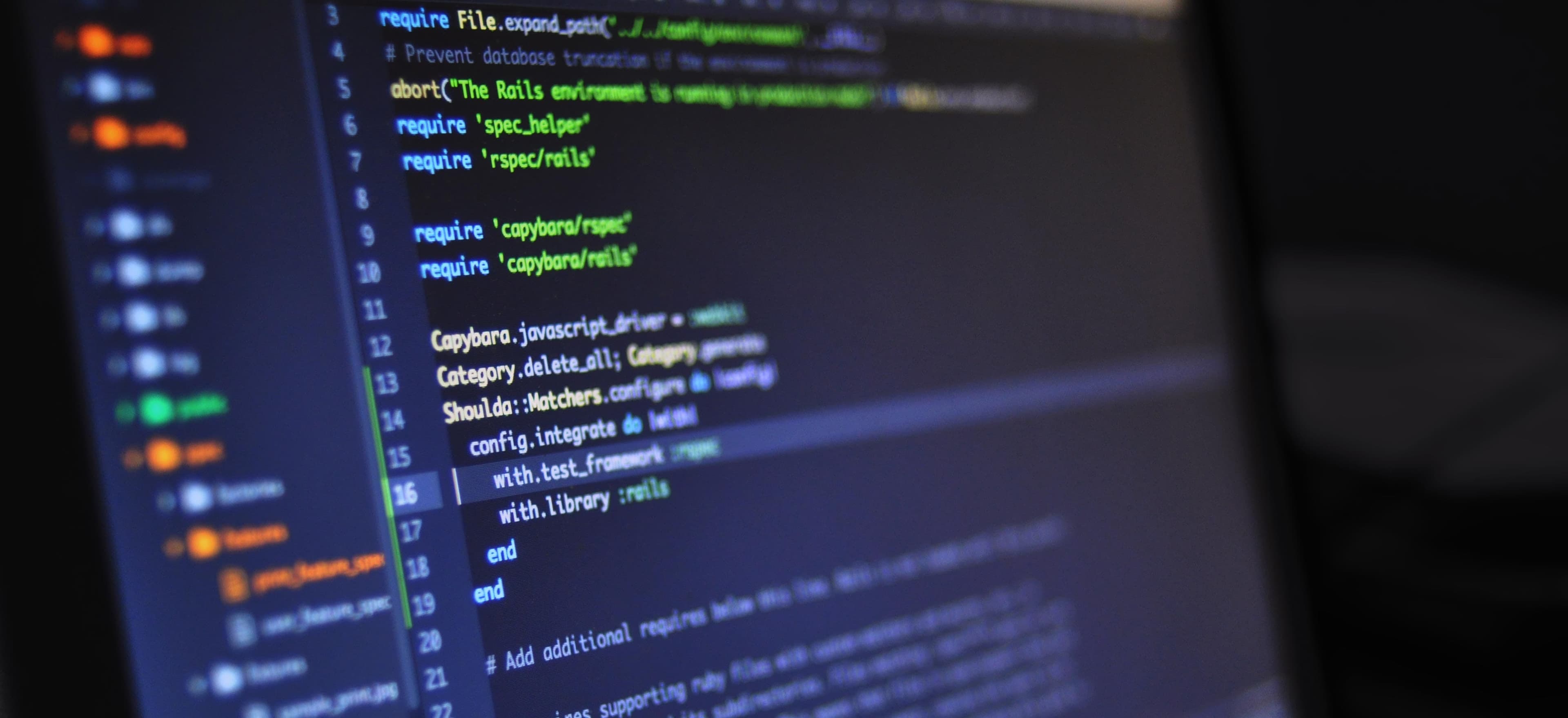
- Published on
Integrating Jython with Java Codebase
Developers working on Java codebases often encounter the need to incorporate scripting languages in their projects. Integrating Jython, the Python implementation in Java, can be a powerful tool to execute Python code within a Java application. In this blog post, we will explore the steps to integrate Jython with a Java codebase, its benefits, and how it can be utilized effectively.
What is Jython?
Jython is an implementation of the Python programming language designed to run on the Java platform. It enables Python code to seamlessly interact with Java code, allowing developers to leverage the strengths of both languages within a single application. By integrating Jython with a Java codebase, developers can achieve greater flexibility, rapid development, and access to a wide range of Python libraries.
Benefits of Integrating Jython with Java
-
Leveraging Python's Rich Ecosystem: Python has a vast ecosystem of libraries for various purposes, such as data analysis, machine learning, and web development. By integrating Jython, Java developers can tap into this extensive collection of Python libraries without having to rewrite them in Java.
-
Rapid Prototyping and Development: Python is known for its concise and expressive syntax, which allows for rapid prototyping and development. Integrating Jython with Java enables developers to write quick scripts and prototypes in Python, utilizing its simplicity and then seamlessly integrate them into the Java codebase.
-
Interoperability: Jython facilitates seamless interaction between Python and Java code, allowing for the exchange of data, objects, and method calls between the two languages. This interoperability can be particularly beneficial when integrating existing Python code or when certain functionalities are better implemented in Python.
Integrating Jython with Java
Step 1: Adding Jython Dependency
The first step is to add the Jython dependency to your Java project. This can be achieved by including the Jython JAR file in the project's classpath. You can download the Jython JAR file from the official website or include it as a Maven or Gradle dependency.
Step 2: Calling Python Code from Java
import org.python.util.PythonInterpreter;
import org.python.core.PyObject;
public class JythonIntegration {
public static void main(String[] args) {
PythonInterpreter pythonInterpreter = new PythonInterpreter();
pythonInterpreter.exec("print('Hello from Python!')");
}
}
In this example, we create a PythonInterpreter
instance and execute a simple Python code snippet to print "Hello from Python!". This demonstrates the seamless integration of Python code within a Java application using Jython.
Step 3: Interacting with Java Objects
import org.python.util.PythonInterpreter;
import org.python.core.PyObject;
public class JythonIntegration {
public static void main(String[] args) {
PythonInterpreter pythonInterpreter = new PythonInterpreter();
pythonInterpreter.exec("from java.util import ArrayList");
pythonInterpreter.exec("list = ArrayList()");
pythonInterpreter.exec("list.add('Item 1')");
pythonInterpreter.exec("list.add('Item 2')");
PyObject pyObject = pythonInterpreter.get("list");
System.out.println(pyObject);
}
}
In this code snippet, we demonstrate how to interact with Java objects from Python code. We create a Java ArrayList
within the Python interpreter, add items to it, and then retrieve the ArrayList
object back into the Java code for further manipulation or processing.
Lessons Learned
Integrating Jython with a Java codebase can significantly enhance the capabilities of a Java application by incorporating the strengths of Python. It enables developers to harness Python's rich ecosystem, rapid prototyping capabilities, and seamless interoperability with Java. By following the outlined steps, developers can seamlessly integrate Jython into their Java projects and reap the benefits of both languages within a single codebase.
In conclusion, integrating Jython with a Java codebase offers a powerful combination of Python's simplicity and Java's robustness, opening up new possibilities for development and code reuse.
To delve deeper into Jython and its integration with Java, you can explore the official Jython documentation.
Happy coding!