How to Efficiently Use MySQL Stored Procedures
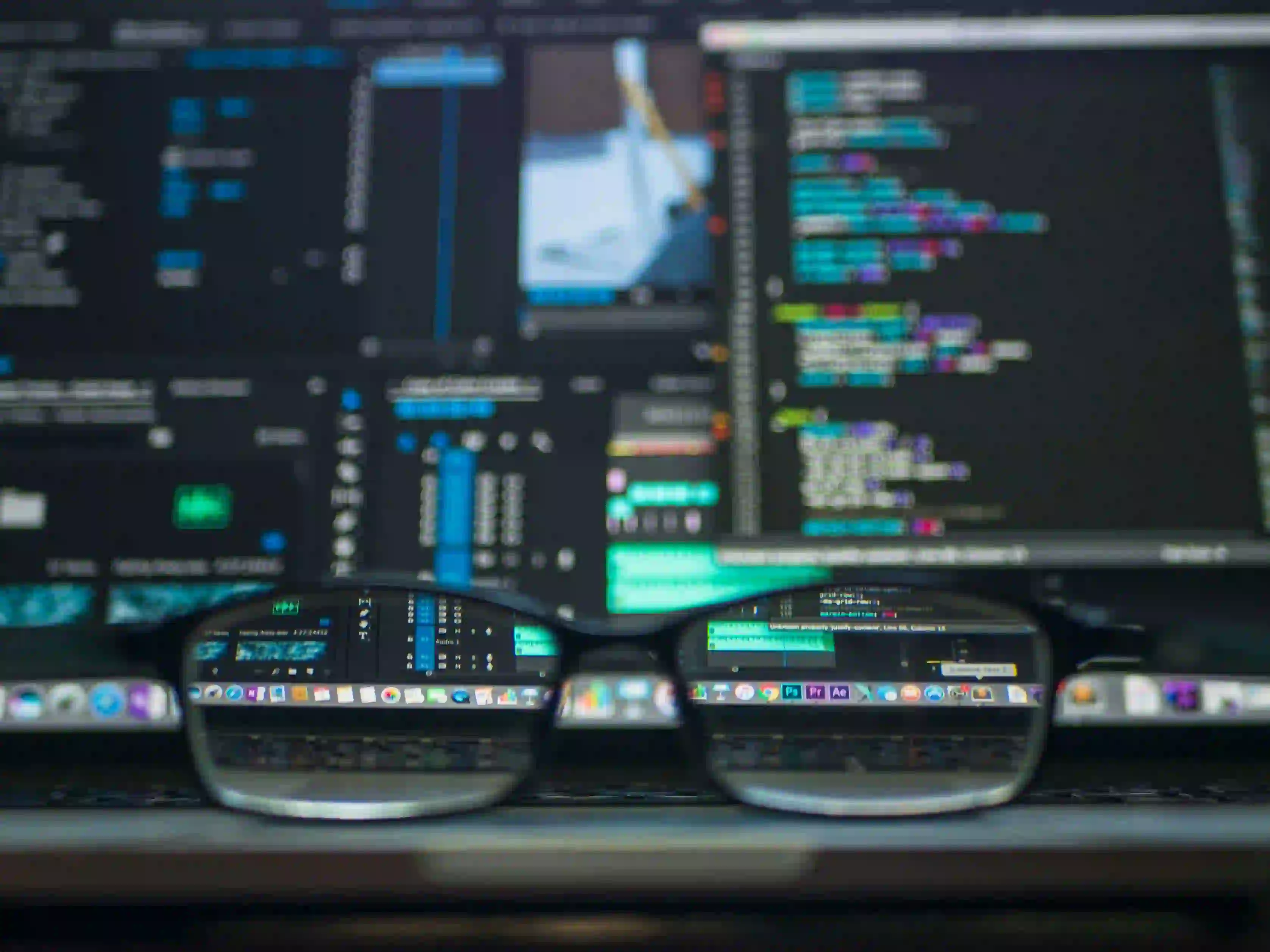
Maximizing Database Efficiency with MySQL Stored Procedures
In the world of database management, optimizing performance is key to ensuring seamless and responsive applications. MySQL stored procedures are a powerful tool that can significantly enhance database efficiency, reduce network traffic, and improve code reusability. In this article, we will delve into the benefits of using stored procedures, explore best practices for their implementation, and provide practical examples in Java.
Understanding MySQL Stored Procedures
What are Stored Procedures?
A stored procedure is a set of SQL statements that have been pre-compiled and stored in the database. These procedures can be invoked through a simple call from within an application, reducing the need to send multiple SQL statements over the network.
Advantages of Using Stored Procedures
- Improved Performance: By pre-compiling the SQL statements, stored procedures minimize the overhead of parsing and optimizing the queries each time they are executed.
- Enhanced Security: Stored procedures can restrict direct access to the tables and provide controlled access to data through parameterized calls, reducing the risk of SQL injection attacks.
- Code Reusability: They encapsulate frequently used logic within the database, enabling multiple applications to call the same procedure, thereby promoting code reusability and maintainability.
Best Practices for Using Stored Procedures
- Parameterized Queries: Always use parameterized queries within stored procedures to prevent SQL injection and improve performance by leveraging query caching.
- Modular Design: Break down complex operations into smaller, reusable stored procedures, promoting maintainability and facilitating code reuse.
- Error Handling: Implement robust error handling within stored procedures to gracefully manage exceptions and ensure data integrity.
Utilizing MySQL Stored Procedures in Java Applications
Connecting to MySQL Database
Before we can invoke a stored procedure from a Java application, we need to establish a connection to the MySQL database. The following code snippet demonstrates how to connect to a MySQL database using JDBC (Java Database Connectivity).
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class MySQLDatabaseConnection {
private static final String URL = "jdbc:mysql://localhost:3306/mydatabase";
private static final String USER = "username";
private static final String PASSWORD = "password";
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(URL, USER, PASSWORD);
}
}
In this example, we utilize the DriverManager
class to establish a connection to the MySQL database by providing the database URL, username, and password.
Invoking a Stored Procedure
Once the database connection is established, we can proceed to invoke a stored procedure from our Java application. Let's consider a scenario where we have a stored procedure named getEmployeeDetails
that retrieves the details of an employee based on their unique identifier.
import java.sql.*;
public class EmployeeDetailsRetriever {
public void getEmployeeDetails(int employeeId) {
try (Connection connection = MySQLDatabaseConnection.getConnection();
CallableStatement callableStatement = connection.prepareCall("{call getEmployeeDetails(?)}")) {
callableStatement.setInt(1, employeeId);
ResultSet resultSet = callableStatement.executeQuery();
// Process the retrieved employee details
while (resultSet.next()) {
// Retrieve and process the employee details
}
} catch (SQLException e) {
// Handle any SQL exceptions
}
}
}
In this example, we use the CallableStatement
interface to prepare and execute the stored procedure getEmployeeDetails
with a parameterized input for the employee ID. The retrieved result set can be iterated over to process the employee details within the Java application.
Why Use Stored Procedures in Java Applications?
- Reduced Network Traffic: By invoking a stored procedure, only the procedure call and its parameters are transmitted over the network, minimizing network traffic compared to sending individual SQL statements.
- Enhanced Scalability: Stored procedures enable the database logic to evolve independently, allowing for optimizations without impacting the application code.
- Improved Security: They provide an additional layer of security by restricting direct access to tables, minimizing the risk of unauthorized data access.
Final Thoughts
Incorporating MySQL stored procedures into Java applications can significantly enhance database efficiency, promote reusability, and strengthen security measures. By leveraging the benefits of stored procedures and adhering to best practices, developers can streamline database interactions and improve overall application performance.
In summary, stored procedures empower developers to encapsulate database logic, minimize network overhead, and enhance code reusability, ultimately contributing to the seamless operation of Java applications backed by MySQL databases.
By harnessing the power of MySQL stored procedures, developers can optimize database performance and foster a robust and efficient application ecosystem.
Remember, efficient database management is the backbone of responsive and scalable applications. Utilizing stored procedures effectively can pave the way for streamlined database interactions and overall application efficiency.
So, why wait? Embrace the power of MySQL stored procedures and propel your Java applications to new heights of efficiency and performance!