Fixing Error Creating Bean: DatasourceAutoConfiguration
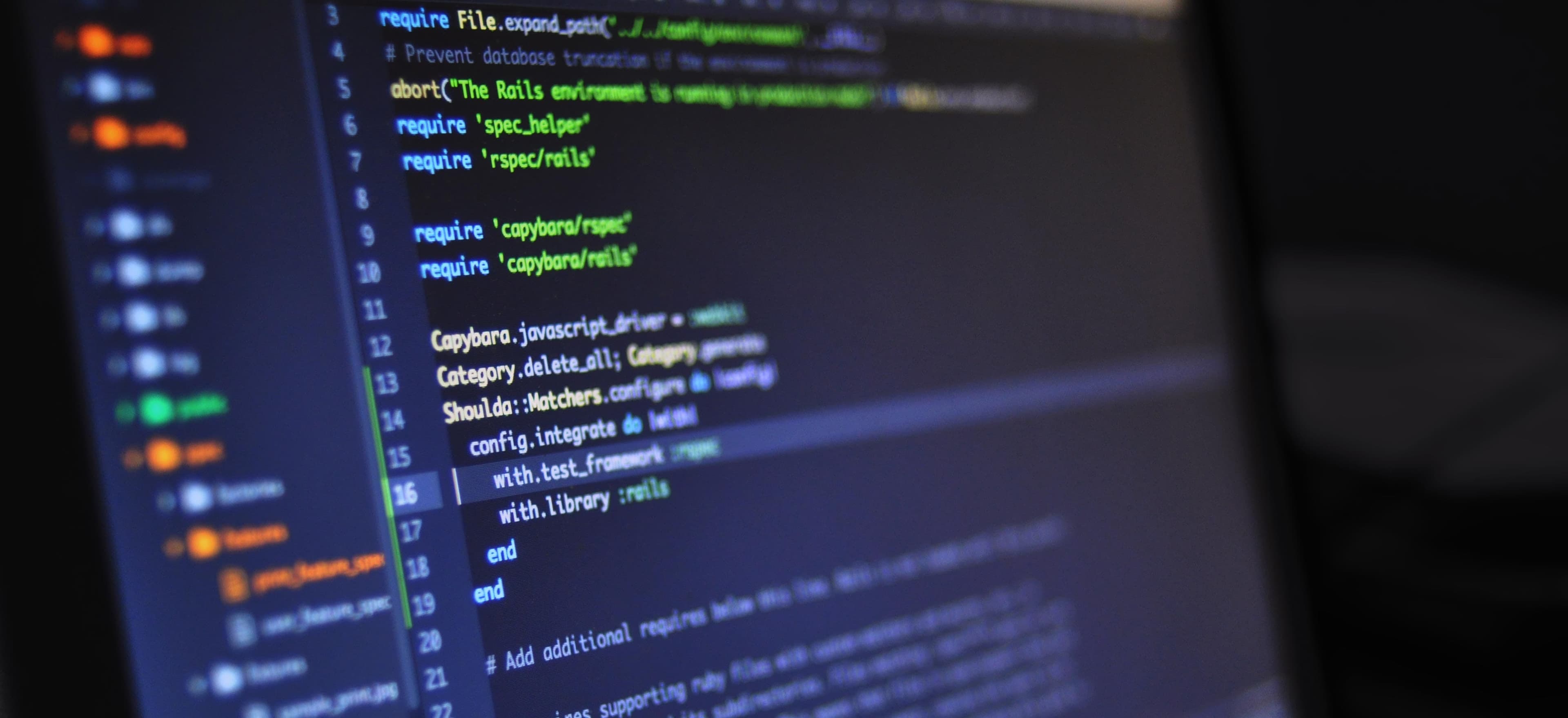
- Published on
Fixing Error Creating Bean: DatasourceAutoConfiguration
In Java Spring applications, you may encounter the "Error creating bean with name 'dataSource' defined in class path resource" error. This can be frustrating, especially if you're new to Spring and are unsure of how to resolve it. In this blog post, we'll explore the potential causes of this error and provide solutions to help you get your Spring application up and running smoothly.
Understanding the Error
The "Error creating bean with name 'dataSource' defined in class path resource" typically occurs when Spring is unable to auto-configure a DataSource bean for your application. This can happen for a variety of reasons, including invalid configuration properties, missing dependencies, or conflicting configurations.
Let's dive into some common causes of this error and how to address them.
Potential Causes and Solutions
Missing Database Dependency
One common reason for this error is a missing database dependency. If your Spring application requires a database connection, ensure that you have included the necessary database driver dependency in your project.
// Example Maven dependency for MySQL database
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
</dependency>
Ensure that the version of the database driver matches the version of the database you are using. Once you have added the appropriate database dependency, re-run your application and check if the error persists.
Incorrect Configuration Properties
Another potential cause of the error is incorrect database configuration properties. Check your application.properties or application.yml file to ensure that the database connection properties are correctly specified.
// Example database configuration properties in application.properties
spring.datasource.url=jdbc:mysql://localhost:3306/mydatabase
spring.datasource.username=root
spring.datasource.password=yourpassword
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
Ensure that the URL, username, password, and driver class name are accurate and match your database configuration. Incorrect properties can lead to the failure of DataSource auto-configuration.
Conflicting Configuration
If your Spring application includes multiple DataSource configurations, it can result in conflicts and lead to the error creating the DataSource bean. Check your codebase for any duplicate or conflicting DataSource configurations and remove or refactor them as needed.
Leveraging Spring Profiles
If your application requires different database configurations for different environments, leveraging Spring profiles can be a powerful way to manage these variations. By using profiles, you can specify separate configuration properties for development, testing, and production environments, ensuring that the DataSource is auto-configured appropriately for each scenario.
// Example Spring profile-specific configuration
@Configuration
@Profile("dev")
public class DevDataSourceConfig {
// Dev database configuration
}
@Configuration
@Profile("prod")
public class ProdDataSourceConfig {
// Production database configuration
}
By utilizing Spring profiles, you can maintain clean and specific configurations for each environment, minimizing the risk of conflicts and errors during DataSource auto-configuration.
Taking Advantage of Spring Boot Auto-Configuration
Spring Boot provides powerful auto-configuration capabilities that can simplify the setup of DataSource beans. By adhering to Spring Boot conventions and best practices, you can often rely on the framework to automatically configure the DataSource based on the dependencies and configurations present in your project.
Ensure that you are following the recommended project structure and naming conventions, as this can significantly reduce the need for explicit DataSource configuration and troubleshooting.
Closing the Chapter
In conclusion, the "Error creating bean with name 'dataSource'" in a Java Spring application can stem from various causes, including missing dependencies, incorrect configuration properties, conflicting configurations, or the absence of Spring profiles and conventions.
By carefully examining your project's dependencies, configurations, and environment-specific requirements, you can address these issues and ensure successful DataSource auto-configuration in your Spring application.
Remember to double-check your database dependencies, review and correct your configuration properties, resolve any conflicting configurations, and leverage Spring profiles and Spring Boot's auto-configuration capabilities to streamline the process.
With these insights and solutions at your disposal, you can effectively resolve the "Error creating bean with name 'dataSource' defined in class path resource" and propel your Spring application towards seamless execution. Happy coding!
For more in-depth information on Spring Boot auto-configuration, check out the official Spring documentation and Spring Boot auto-configuration guide.
Checkout our other articles