Solving Image Caching Issues in Android Development
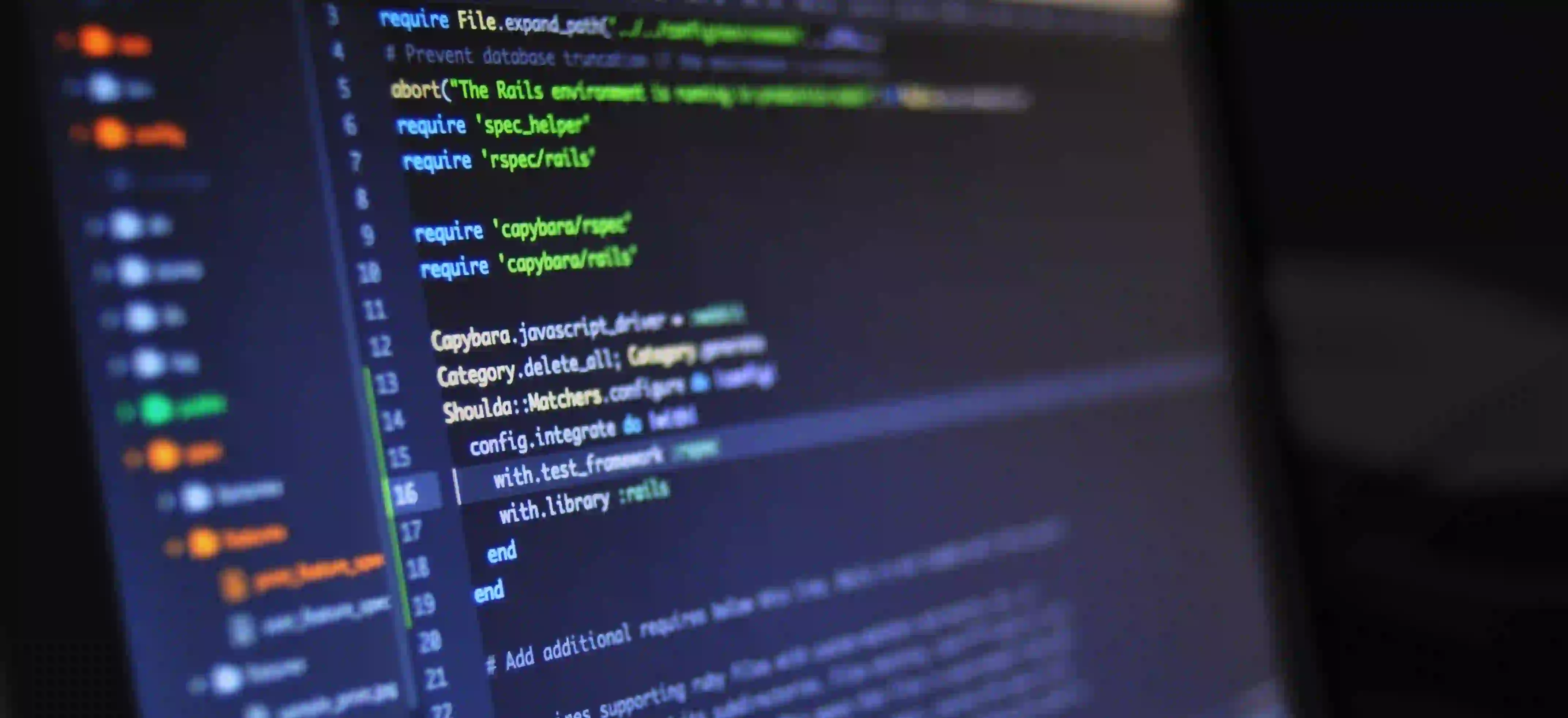
Solving Image Caching Issues in Android Development
Image caching is an essential aspect of Android development. It significantly impacts application performance, user experience, and the amount of data consumed. The goal of this blog post is to provide insights into image caching issues, common pitfalls, and effective strategies for resolving them, making your Android applications more efficient.
Why Image Caching Matters
In the context of Android development, image caching refers to storing images locally on a device, which helps reduce bandwidth usage and loading times. Images often take longer to load due to network latency, heavy file sizes, or inefficient loading strategies. By caching these images, you can improve your application's speed and make it more user-friendly.
Benefits of Proper Caching
- Reduced Load Times: Caching images can significantly speed up subsequent image loads, making the application feel snappier.
- Lower Data Usage: Users can save on data costs when images are loaded from local storage instead of being repeatedly downloaded.
- Enhanced Performance: Efficient caching strategies reduce the burden on the server and enhance the overall performance of the application.
Understanding Image Caching Mechanisms
There are several methods for caching images in Android:
- Memory Caching
- Disk Caching
- Network Caching
Memory Caching vs. Disk Caching
Memory caching stores images in RAM for fast access. While it provides quick retrieval, it is limited by the device's memory. On the other hand, disk caching stores images on storage, which is slower but can handle larger datasets.
It's crucial to utilize both methods effectively to maximize performance. A well-integrated caching solution can ensure smooth image loading.
Popular Libraries for Image Caching
When working with image caching in Android, several libraries can simplify the process. Here are a few notable libraries:
Each of these libraries has its advantages, but generally, they all offer appropriate caching strategies.
Common Caching Issues
While implementing image caching, you may encounter several issues:
- Outdated Cached Images: The cached images may become stale, showing older versions of the images.
- Increased Cache Size: Over time, cached images can consume considerable disk or memory space.
- Concurrency Problems: Concurrency issues can arise when multiple threads attempt to access or modify the cache simultaneously.
- Network Latency: Even with caching, network issues can sometimes hinder image loading performance.
1. Outdated Cached Images
To handle outdated cached images, consider adding cache versioning or using HTTP headers. This will instruct the cache to refresh when needed. Using libraries like Glide and Picasso can often help manage cache expiration seamlessly.
Example with Glide:
Glide.with(context)
.load(imageUrl)
.diskCacheStrategy(DiskCacheStrategy.RESOURCE) // cache freshly downloaded images
.into(imageView);
In the above example, the diskCacheStrategy
dictates how Glide handles caching. The RESOURCE
strategy allows it to refresh the cache when there's an updated version of the image.
2. Increased Cache Size
Monitor the cache size and implement a strategy to clear it periodically or when specific conditions are met. You may employ an LRU (Least Recently Used) caching mechanism.
Example of Limiting Cache Size:
Picasso picasso = new Picasso.Builder(context)
.downloader(new OkHttp3Downloader(context, cacheSize))
.build();
In this case, cacheSize
defines the maximum size of the disk cache in bytes. Setting this size appropriately prevents your cache from growing indefinitely.
3. Concurrency Problems
Concurrency issues can arise when multiple threads attempt to access or update the cache concurrently. It’s essential to use thread-safe methods or synchronize access to cache.
Example Using synchronized:
private final Object cacheLock = new Object();
public Bitmap getImageFromCache(String url) {
synchronized (cacheLock) {
return cache.get(url); // thread-safe access
}
}
Using synchronization ensures that only one thread accesses the cache at a time, preventing potential data inconsistencies.
4. Network Latency
Even with caching, network latency can still occur, especially if your application relies on a remote server for images. To mitigate this, consider implementing a local fallback mechanism.
Picasso.get()
.load(imageUrl)
.error(R.drawable.error_image) // Fallback image in case of failure
.into(imageView);
In this example, an error drawable appears if loading fails, enhancing user experience.
Best Practices for Image Caching
- Choose the Right Library: Select a library that meets your application’s needs while providing efficient caching methods.
- Set Appropriate Cache Limits: Establish both memory and disk cache limits to manage the resources effectively.
- Monitor Cache States: Log cache states and sizes during development for optimization.
- Implement Cache Versioning: Consider using versioning techniques to manage outdated images.
- Test Thoroughly: Make sure to test image caching under various network conditions and app states.
Summary
In Android development, effective image caching is vital for building high-performance applications. By understanding caching mechanisms, potential issues, and common practices, you can improve user experience and resource management within your application.
For more advanced scenarios, consider diving into custom caching solutions or leverage existing libraries further, tapping into their extensive capabilities.
Additional Resources
By following these guidelines and integrating the suggested practices into your application, you’ll be well on your way to efficiently handling image caching issues in Android development. Remember, performance and user experience are key to a successful app. Happy coding!