Understanding the Concepts and Applications of Aspect-Oriented Programming
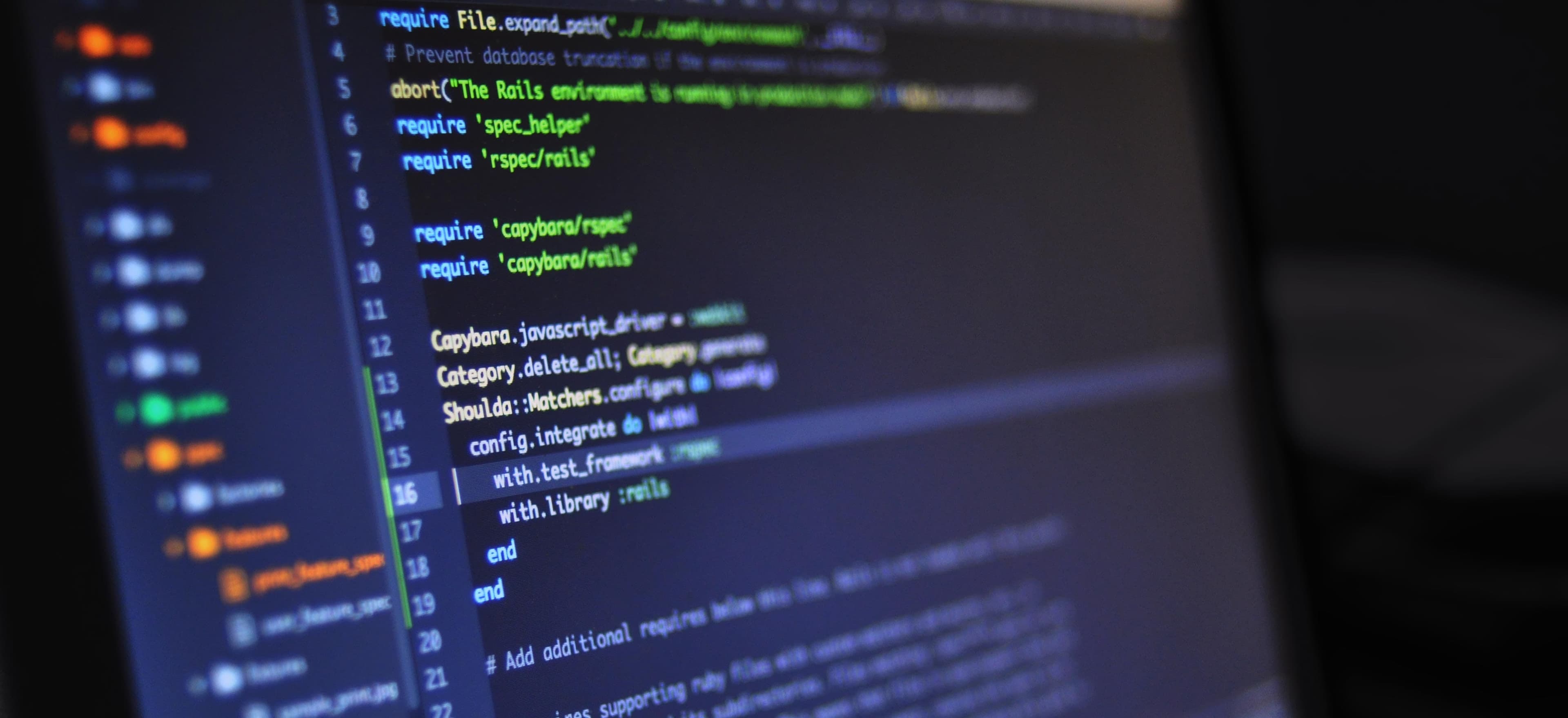
- Published on
Understanding the Concepts and Applications of Aspect-Oriented Programming
In the realm of software development, the need for modularity and maintainability has always been paramount. In response to this, various programming paradigms and methodologies have emerged, one of which is Aspect-Oriented Programming (AOP). AOP aims to address cross-cutting concerns in programming, making it a valuable tool in software development.
What is Aspect-Oriented Programming?
At its core, Aspect-Oriented Programming (AOP) is a programming paradigm that aims to increase modularity by allowing the separation of cross-cutting concerns. In traditional programming, concerns such as logging, security, and transaction management tend to be interspersed throughout the code base, leading to a lack of modularity and code tangling. AOP seeks to address this issue by providing a way to modularize these concerns and apply them to the appropriate parts of the codebase.
How Does Aspect-Oriented Programming Work?
AOP achieves its goal by introducing the concept of an "aspect," which encapsulates cross-cutting concerns. It allows developers to define aspects separately from the main business logic of the application. These aspects can then be applied to the code at specific join points, which are points in the execution of the code such as method invocations, field accesses, or object instantiations.
Key Concepts in Aspect-Oriented Programming
1. Join Points
Join points are specific points in the execution of the program where the aspect's behavior can be applied. These points can include method calls, object instantiations, or variable access.
2. Pointcuts
Pointcuts are expressions that define a set of join points where the aspect should be applied. They allow developers to specify the conditions under which the aspect's behavior should be executed.
3. Advice
Advice is the actual action taken by the aspect at a particular join point. It can be executed before, after, or around the join point.
4. Weaving
Weaving is the process of applying aspects to the appropriate join points in the code. This can be done at compile time, load time, or runtime.
Example of Aspect-Oriented Programming in Java
Let's consider a simple logging example to illustrate the use of AOP in Java. Suppose we have a Calculator
class with a divide
method, and we want to log the parameters and result of the method execution.
First, we define a pointcut that captures the execution of the divide
method:
pointcut divideExecution(): execution(* Calculator.divide(int, int));
Next, we define the advice that performs the logging:
before(): divideExecution() {
System.out.println("Logging the division operation");
}
We then weave the aspect into our Calculator
class using the defined pointcut and advice.
Calculator calculator = new Calculator();
calculator.divide(10, 2); // This will trigger the logging advice
Benefits of Aspect-Oriented Programming
1. Modularity
AOP promotes modularity by separating cross-cutting concerns from the main business logic. This leads to cleaner, more maintainable code.
2. Reusability
Aspects can be applied to multiple parts of the codebase, promoting code reuse and reducing duplication of concern-related code.
3. Encapsulation of Cross-Cutting Concerns
AOP allows for the encapsulation of cross-cutting concerns, making it easier to maintain and evolve the codebase without affecting the core business logic.
4. Improved Readability
By separating cross-cutting concerns from the main logic, AOP can lead to improved code readability and understanding.
Considerations and Best Practices for Aspect-Oriented Programming
1. Careful Identification of Cross-Cutting Concerns
It's important to carefully identify and define cross-cutting concerns to avoid over-engineering and unnecessary abstraction.
2. Avoiding Overuse
While AOP can be a powerful tool, overuse of aspects can lead to code that is difficult to understand and maintain. It's important to use AOP judiciously.
3. Testing
Aspects can introduce complexities into testing and debugging, so it's important to consider the impact of aspects on testing strategies.
4. Tooling and Support
Choose the right AOP framework and tooling for the specific requirements of the project. Spring AOP and AspectJ are popular choices for AOP in Java.
My Closing Thoughts on the Matter
Aspect-Oriented Programming is a valuable paradigm in the software development toolkit. By addressing cross-cutting concerns and promoting modularity, AOP can contribute to cleaner, more maintainable codebases. However, it's essential to wield AOP judiciously and understand its implications on code maintainability and testing. When used appropriately, Aspect-Oriented Programming can be a powerful ally in the pursuit of clean, modular code.
With a clear understanding of AOP and its applications, developers can leverage its benefits to build robust and maintainable software systems.
By mastering the concepts of AOP, developers can elevate their programming skills and contribute to the creation of more efficient and maintainable software.
In conclusion, Aspect-Oriented Programming opens a world of possibilities for cleaner, more modular code, and it is a tool well worth adding to the arsenal of any software developer.