Troubleshooting OAuth2 Configuration in Spring Boot
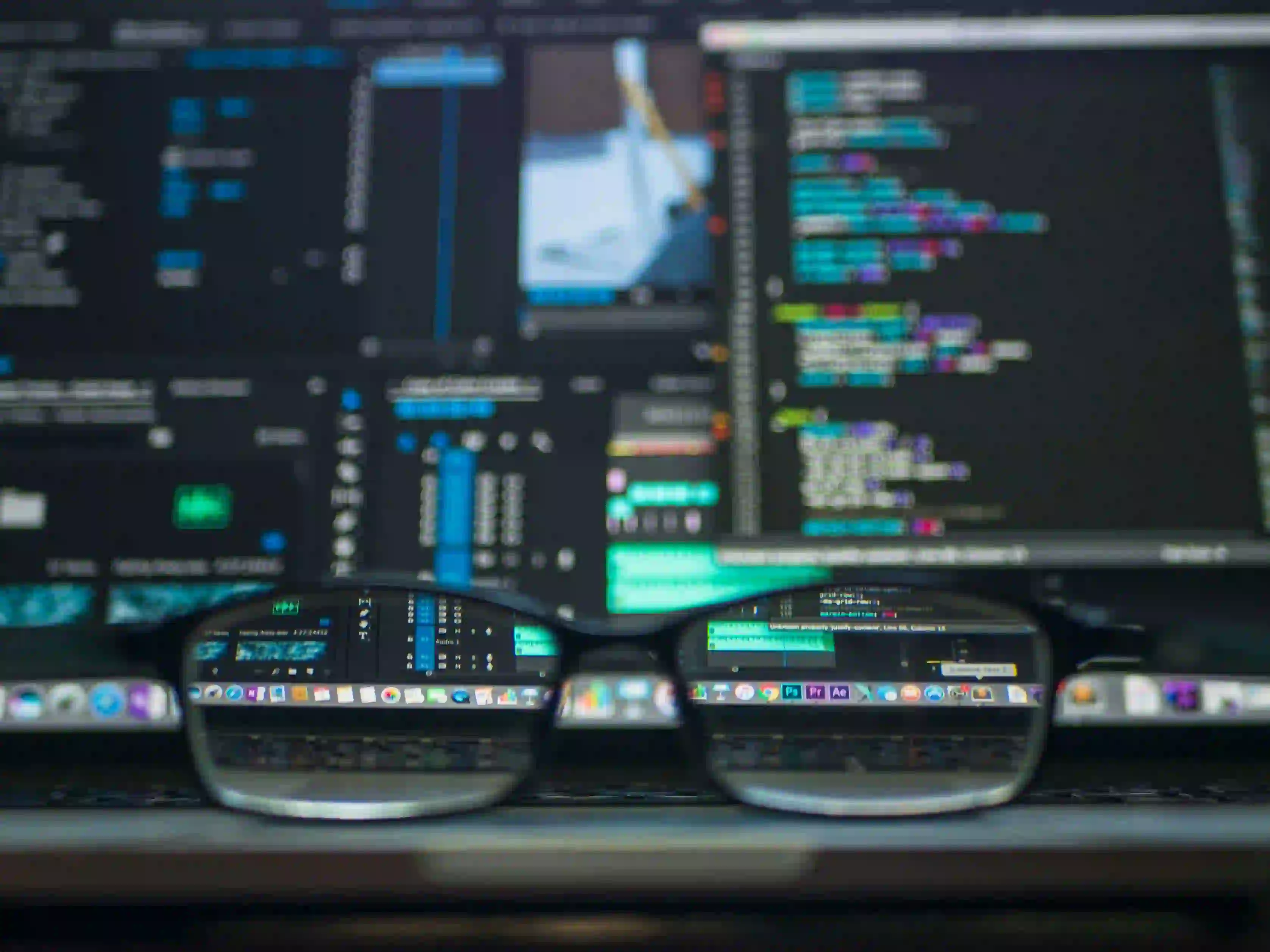
Troubleshooting OAuth2 Configuration in Spring Boot
OAuth2 is a widely used standard for secure authorization, and integrating it into a Spring Boot application is a common task for many developers. However, like any technology, issues may arise during the configuration process. In this article, we will explore some common problems that developers encounter when configuring OAuth2 in a Spring Boot application and provide solutions to address them.
Problem: Unable to Obtain Access Token
One common issue when working with OAuth2 in Spring Boot is being unable to obtain an access token from the authorization server. This can occur due to misconfigured client credentials or improper configuration of the AuthorizationServerConfigurerAdapter
.
One possible cause for this issue is an incorrect client registration in the application properties file. Ensure that the client-id
and client-secret
are correctly specified.
spring:
security:
oauth2:
client:
registration:
your-client-id:
client-id: your-client-id
client-secret: your-client-secret
client-authentication-method: basic
scope: openid,profile,email
Additionally, double-check the configuration of the AuthorizationServerConfigurerAdapter
to ensure that it properly exposes the token endpoint and configures the token store.
@EnableAuthorizationServer
@Configuration
public class AuthorizationServerConfig extends AuthorizationServerConfigurerAdapter {
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients
.inMemory()
.withClient("your-client-id")
.secret("{noop}your-client-secret")
.authorizedGrantTypes("authorization_code", "refresh_token")
.scopes("openid", "profile", "email");
}
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) throws Exception {
endpoints.tokenStore(tokenStore);
}
}
Problem: Invalid Redirect URI
Another common issue is encountering an "invalid redirect URI" error when attempting to authenticate with the OAuth2 provider. This problem often occurs due to a mismatch between the configured redirect URI in the client registration and the actual redirect URI used in the application.
spring:
security:
oauth2:
client:
registration:
your-client-id:
client-id: your-client-id
client-secret: your-client-secret
redirect-uri: "{baseUrl}/login/oauth2/code/{registrationId}"
client-authentication-method: basic
scope: openid,profile,email
Ensure that the redirect-uri
matches the actual URL used for the OAuth2 callback in your application. For example, if your application is running on http://localhost:8080
, the redirect URI should be http://localhost:8080/login/oauth2/code/{registrationId}
.
Problem: Missing User Data After Successful Authentication
After successful authentication using OAuth2, you may encounter issues with missing user data such as the user's email, name, or profile information. This is often caused by incomplete or incorrect configuration of the user info endpoint.
@EnableOAuth2Sso
@Configuration
public class OAuth2SsoConfig extends WebSecurityConfigurerAdapter {
@Override
public void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/login/**", "/error**")
.permitAll()
.anyRequest()
.authenticated()
.and()
.oauth2Login()
.userInfoEndpoint()
.userService(oAuth2UserService);
}
}
In the configuration above, ensure that the userService
provided to the userInfoEndpoint
correctly maps the user attributes from the OAuth2 provider to the application's user details.
Problem: CSRF Token Validation Failure
When integrating OAuth2 with Spring Security, you may encounter CSRF token validation failures during the authentication process. This often happens when the application's security configuration does not exclude the OAuth2 endpoints from CSRF protection.
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/oauth2/**", "/login/**", "/error/**")
.permitAll()
.anyRequest()
.authenticated()
.and()
.oauth2Login()
.and()
.csrf()
.ignoringAntMatchers("/oauth2/**");
}
}
In the above example, we have configured the OAuth2 endpoints to be excluded from CSRF protection using .ignoringAntMatchers("/oauth2/**")
. This ensures that CSRF validation does not interfere with the OAuth2 authentication flow.
Closing Remarks
In this article, we have discussed common issues that developers may encounter when configuring OAuth2 in a Spring Boot application and provided solutions to address these problems. By carefully reviewing and troubleshooting the configuration of client credentials, redirect URIs, user data retrieval, and CSRF token validation, developers can ensure a smooth integration of OAuth2 authentication in their applications.
Remember, troubleshooting OAuth2 issues often requires close attention to detail, including the correct configuration of client properties, endpoint URLs, and security settings. With a solid understanding of these common problems and their solutions, developers can effectively diagnose and resolve OAuth2 configuration issues in their Spring Boot applications.
For further information on OAuth2 configuration in Spring Boot, you may find it useful to refer to the official documentation for detailed guidance and best practices.
Now, armed with these troubleshooting strategies, you can confidently tackle OAuth2 configuration challenges in your Spring Boot applications. Happy coding!