Mastering MVC 1.0 Routing: Find the Best Approach
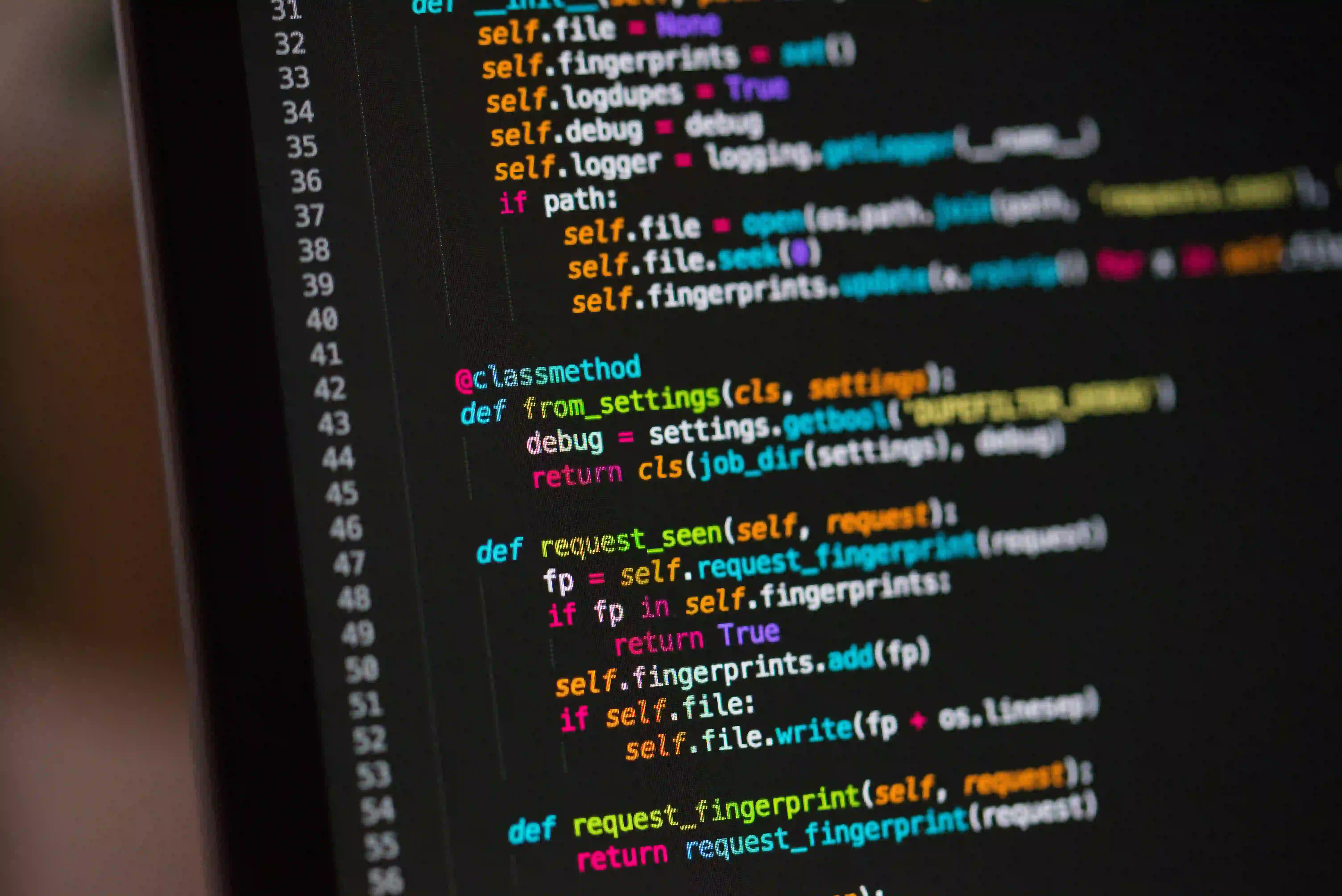
Mastering MVC 1.0 Routing: Find the Best Approach
Search engine optimization (SEO) is the backbone of any successful online content. However, achieving high search engine rankings often relies on more than just keyword optimization and quality content. The way content is structured and presented can also have a significant impact on its search visibility. In this blog post, we will explore the key concepts of MVC 1.0 routing in Java and discuss the best approaches to maximize SEO benefits while leveraging this powerful feature.
Understanding MVC 1.0 Routing
What is MVC?
MVC (Model-View-Controller) is a software architectural design pattern commonly used for developing user interfaces. It separates the application into three interconnected components - the model (data), the view (UI), and the controller (handling user input).
Setting the Stage to MVC 1.0 Routing
MVC 1.0 routing is a feature that allows developers to map URLs to specific controller actions. This enables the creation of clean, readable URLs and provides a means to organize the application's functionality.
Importance of SEO in Web Development
Why SEO Matters
Search engine optimization (SEO) is crucial for ensuring that your web content is discoverable and ranks well in search engine results. It encompasses various techniques and strategies aimed at enhancing a website's visibility to search engines and, ultimately, to users.
SEO-friendly URLs
One of the fundamental elements of SEO is the URL structure. Search engines, like Google, prefer URLs that are descriptive, readable, and contain relevant keywords. Clean and structured URLs improve the user experience and make it easier for search engine crawlers to index the content.
Optimizing MVC 1.0 Routing for SEO
Choosing Descriptive URLs
When designing routes in MVC 1.0, it's essential to prioritize clear and meaningful URLs. This not only makes the URLs more user-friendly but also aligns with SEO best practices. For example:
@Path("/articles/{id}/{title}")
public class ArticleController {
@GET
public String viewArticle(@PathParam("id") int id, @PathParam("title") String title) {
// Implementation
}
}
In the above code snippet, the URL pattern /articles/{id}/{title}
is far more descriptive and SEO-friendly compared to generic patterns.
Utilizing Keywords in Routes
Incorporating relevant keywords into route patterns can have a positive impact on SEO. It's essential to consider the words users are likely to search for and integrate them into the URLs where appropriate.
@Path("/java-development/{topic}")
public class JavaController {
@GET
public String viewTopic(@PathParam("topic") String topic) {
// Implementation
}
}
By including the keyword "java-development" in the route, the URL becomes more targeted and relevant to users interested in Java development topics.
Canonical URLs
Canonical URLs help consolidate the indexing properties of sets of URLs into a single authoritative URL. When implementing MVC 1.0 routing, it's crucial to ensure that each piece of content has a canonical URL, thus avoiding duplicate content issues.
@RequestPath("/products")
public class ProductController {
@GET
public String viewProductList() {
// Implementation
}
@GET
@Path("/{id}/{name}")
public String viewProductDetails(@PathParam("id") int id, @PathParam("name") String name) {
// Implementation
}
}
In the above example, setting the canonical tag within the HTML head for each product detail page can help search engines understand the preferred URL for indexing.
Creating SEO-friendly Redirects
Implementing redirects is a common requirement in web applications. When it comes to SEO, it's important to choose the right type of redirect and handle them properly within MVC 1.0 routing.
Using 301 Redirects
A 301 redirect is a permanent redirect that passes the SEO value from the old URL to the new one. It is essential for preserving search engine rankings when migrating content or making changes to URL structures.
@Path("/old-url")
public class RedirectController {
@GET
public Response redirect() {
return Response.seeOther(URI.create("/new-url")).status(301).build();
}
}
By using a 301 redirect, any SEO value associated with the old URL will be transferred to the new URL, ensuring minimal impact on search rankings.
Handling Dynamic Routes and Pagination
Dynamic routes and pagination are common features in web applications, especially when dealing with large sets of data. It's important to handle dynamic routes and pagination in a way that is both SEO-friendly and user-friendly.
Dynamic Route Handling
When dealing with dynamic routes, such as category pages or user profiles, it's crucial to ensure that the URLs are structured in a logical and predictable manner.
@Path("/users/{username}")
public class UserController {
@GET
public String viewUserProfile(@PathParam("username") String username) {
// Implementation
}
}
By maintaining a clear and consistent URL structure for dynamic routes, both users and search engines can better understand and navigate the content.
Pagination and SEO
Pagination can present challenges for SEO, especially when managing large sets of paginated content. Employing best practices, such as using rel="prev" and rel="next" link tags and implementing canonical URLs, is essential for signaling the relationship between paginated pages and consolidating their SEO signals.
@Path("/articles")
public class ArticleController {
@GET
public String viewArticleList(@QueryParam("page") int page) {
// Implementation
}
}
Adhering to best practices ensures that search engines understand the structure of paginated content and can attribute SEO value appropriately.
Closing Remarks
In conclusion, mastering MVC 1.0 routing for SEO involves designing clear, descriptive URLs, incorporating relevant keywords, handling redirects properly, and addressing the challenges of dynamic routes and pagination. By adhering to best practices and considering the impact of routing on SEO, developers can optimize their web applications for improved search engine visibility and user experience.
By leveraging the power of MVC 1.0 routing and aligning it with SEO best practices, developers can create web applications that are not only functionally robust but also readily discoverable and user-friendly.
For more in-depth understanding of SEO best practices for Java web development, consider exploring resources from reputable sources such as Google's Search Engine Optimization Starter Guide.
Thank you for reading! If you have any questions or insights to share, feel free to leave a comment below.