Composing Multiple Async Results with Java 8
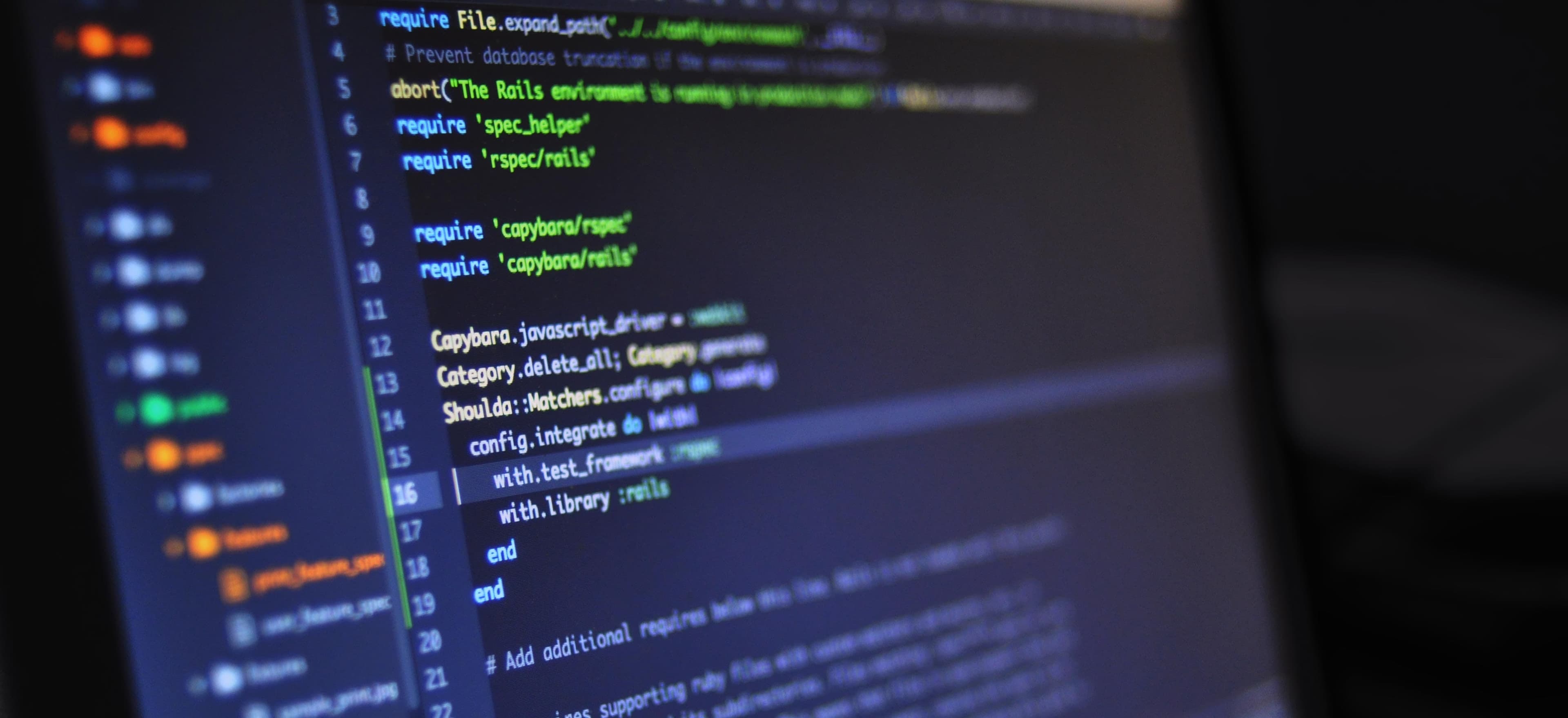
- Published on
Composing Multiple Async Results with Java 8
In today's fast-paced world, asynchronous programming has become increasingly important in the world of software development. Java 8 introduced the CompletableFuture class, which is a powerful tool for managing and composing asynchronous results. In this article, we'll explore how to use CompletableFuture to compose multiple asynchronous results in Java 8.
Understanding CompletableFuture
Before we dive into composing multiple asynchronous results, let's first understand the basics of CompletableFuture. CompletableFuture is a class that represents a future result of an asynchronous computation. It allows you to chain multiple asynchronous operations together and provides various methods for handling their results.
One of the key features of CompletableFuture is its ability to combine the results of multiple asynchronous computations. This is often useful in scenarios where you need to perform multiple independent asynchronous tasks and then combine their results to produce a final outcome.
Composing Multiple Async Results
Running Async Tasks in Parallel
To demonstrate composing multiple async results, let's consider a scenario where we need to fetch data from two different external APIs and then combine their results. We can use CompletableFuture to run these two tasks in parallel and then combine their results once both tasks are completed.
CompletableFuture<String> task1 = CompletableFuture.supplyAsync(() -> fetchDataFromApi1());
CompletableFuture<String> task2 = CompletableFuture.supplyAsync(() -> fetchDataFromApi2());
CompletableFuture<Void> combinedTask = CompletableFuture.allOf(task1, task2);
combinedTask.thenRun(() -> {
String result1 = task1.join();
String result2 = task2.join();
String combinedResult = combineResults(result1, result2);
System.out.println(combinedResult);
});
In the above example, we use supplyAsync
method to run the tasks asynchronously. We then use allOf
method to create a new CompletableFuture that completes when all of the given CompletableFutures complete. Finally, we use the thenRun
method to specify a callback to be executed once both tasks are completed, where we combine the results and print the combined result.
Handling Errors
When composing multiple async results, it's important to handle any potential errors that may occur during the asynchronous computations. CompletableFuture provides methods for handling errors, such as exceptionally
and handle
, which allow you to gracefully manage any exceptions that may occur during the execution of the asynchronous tasks.
CompletableFuture<String> task1 = CompletableFuture.supplyAsync(() -> fetchDataFromApi1())
.exceptionally(ex -> "Error fetching data from API1");
CompletableFuture<String> task2 = CompletableFuture.supplyAsync(() -> fetchDataFromApi2())
.exceptionally(ex -> "Error fetching data from API2");
CompletableFuture<Void> combinedTask = CompletableFuture.allOf(task1, task2);
combinedTask.thenRun(() -> {
if (!task1.isCompletedExceptionally() && !task2.isCompletedExceptionally()) {
String result1 = task1.join();
String result2 = task2.join();
String combinedResult = combineResults(result1, result2);
System.out.println(combinedResult);
} else {
System.out.println("One or both tasks failed");
}
});
In the above example, we use the exceptionally
method to handle any exceptions that may occur during the execution of the asynchronous tasks. We then check if any of the tasks completed exceptionally before combining their results.
Combining Results with Further Processing
Sometimes, you may need to combine the results of multiple async tasks and then perform further processing on the combined result. CompletableFuture provides methods like thenApply
, thenCompose
, and thenCombine
for composing the results of multiple CompletableFutures and performing additional processing on the combined result.
CompletableFuture<String> task1 = CompletableFuture.supplyAsync(() -> fetchDataFromApi1());
CompletableFuture<String> task2 = CompletableFuture.supplyAsync(() -> fetchDataFromApi2());
CompletableFuture<String> combinedTask = task1.thenCombine(task2, (result1, result2) -> combineResults(result1, result2))
.thenApply(finalResult -> "Processed result: " + finalResult);
System.out.println(combinedTask.get());
In the above example, we use the thenCombine
method to combine the results of the two tasks and apply a function to the combined results. We then use the thenApply
method to further process the combined result before finally printing the processed result.
Closing the Chapter
Composing multiple asynchronous results with CompletableFuture in Java 8 provides a powerful way to manage and combine the results of independent asynchronous tasks. It allows for efficient utilization of resources by running tasks in parallel and handling their results in a flexible and expressive manner. By understanding and leveraging the capabilities of CompletableFuture, developers can create robust and responsive asynchronous applications.
In this post, we've only scratched the surface of what CompletableFuture can do. For more in-depth information, you can refer to the official Java documentation on CompletableFuture.
In conclusion, CompletableFuture represents a significant enhancement to Java's concurrency model, offering a wide array of functionality for asynchronous programming, and composing multiple async results is just one of its many powerful features.