Optimizing Spring Data JPA Performance with MySQL
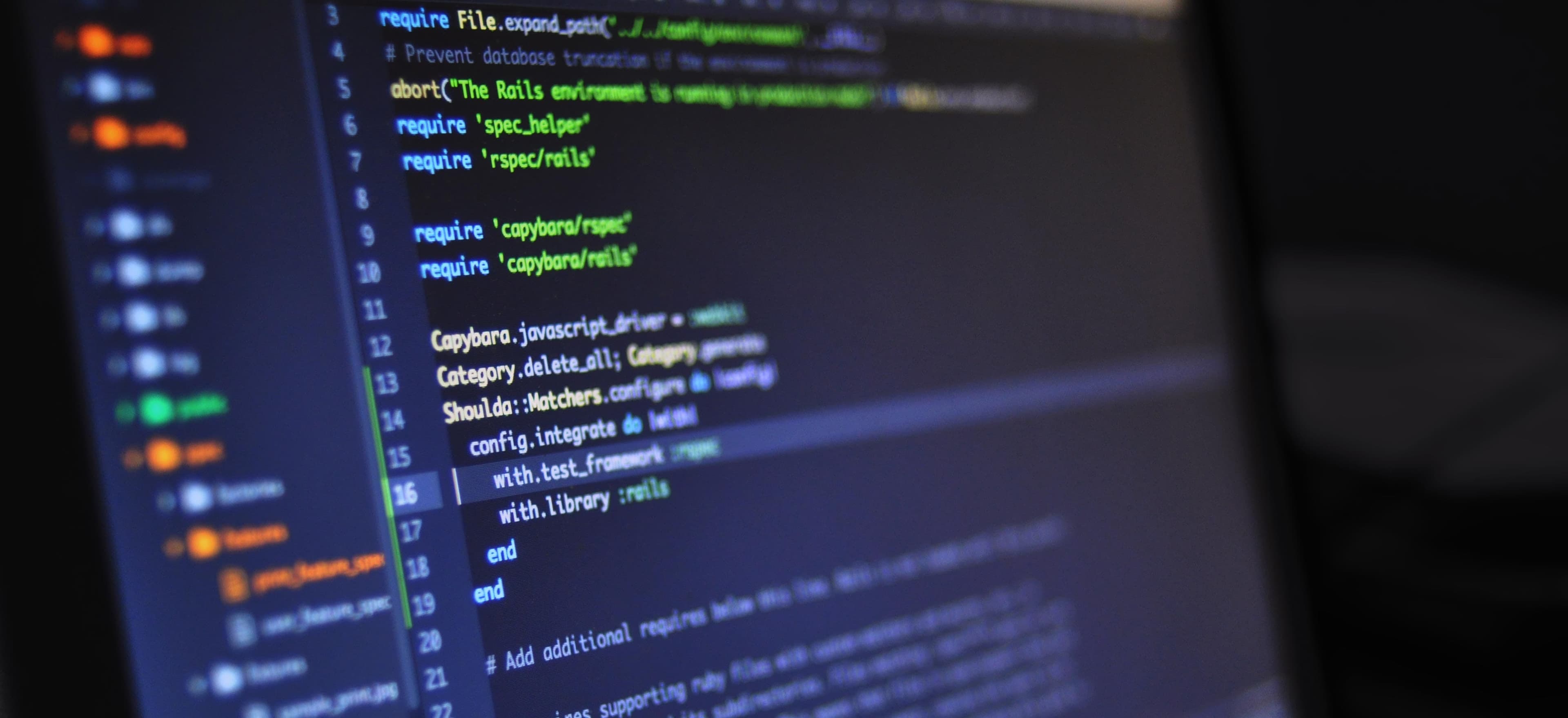
- Published on
Optimizing Spring Data JPA Performance with MySQL
When building applications with Spring Data JPA and MySQL, optimizing performance becomes crucial for achieving scalable and efficient data operations. In this article, we will explore various techniques and best practices to optimize the performance of Spring Data JPA applications using MySQL as the underlying database.
1. Understanding the Importance of Performance Optimization
Performance optimization is essential for ensuring that an application can handle a growing user base and increasing data volume without compromising on responsiveness and scalability. By optimizing the performance of Spring Data JPA applications with MySQL, developers can minimize query execution times, reduce resource utilization, and deliver a seamless user experience.
2. Efficient Entity Design
One of the fundamental aspects of optimizing performance in Spring Data JPA is designing efficient entities. When defining JPA entities, it's essential to consider the relationships between entities, use appropriate data types, and apply indexing where necessary.
Example Entity Design
@Entity
@Table(name = "products")
public class Product {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String name;
@Column(nullable = false)
private BigDecimal price;
// Other fields, getters, and setters
}
In the above example, the @Entity
annotation defines a JPA entity, and the @Table
annotation specifies the corresponding database table. By carefully designing entities and their relationships, developers can avoid unnecessary joins and reduce data retrieval overhead.
3. Fetching Strategies
In Spring Data JPA, the fetching strategy determines how related entities are loaded from the database. Selecting an appropriate fetching strategy can significantly impact the performance of data retrieval operations.
Lazy Loading
@Entity
public class Order {
// Other fields
@OneToMany(mappedBy = "order", fetch = FetchType.LAZY)
private List<OrderItem> items;
}
When using lazy loading (FetchType.LAZY
), related entities are fetched from the database only when accessed, which can prevent unnecessary data retrieval and improve performance. However, developers should carefully manage lazy loading to avoid potential issues such as N+1 queries.
4. Indexing for Query Performance
Indexing plays a crucial role in optimizing query performance, especially when dealing with large datasets. By creating appropriate indexes on columns frequently used in queries, developers can enhance data retrieval speed and overall application responsiveness.
Adding Indexes
@Entity
public class Product {
// Other fields
@Column(nullable = false)
@Index(name = "idx_name")
private String name;
@Column(nullable = false)
private BigDecimal price;
// Other fields
}
In the above example, the @Index
annotation is used to specify an index on the name
column. By adding indexes to frequently queried columns, developers can reduce the time taken to fetch data from the database.
5. Query Optimization
Efficiently crafted queries are vital for improving the performance of Spring Data JPA applications. Developers should leverage query optimization techniques such as proper resource allocation, query caching, and using query hints when necessary.
Example Query Optimization
@Repository
public interface ProductRepository extends JpaRepository<Product, Long> {
@QueryHints(value = @QueryHint(name = HINT_CACHEABLE, value = "true"))
List<Product> findByName(String name);
}
In the above example, the @QueryHint
annotation is used to enable caching for the findByName
query. By caching frequently executed queries, developers can reduce database load and improve overall application performance.
6. Connection Pooling
Managing database connections efficiently is crucial for optimal performance. With MySQL, utilizing a connection pool helps mitigate the overhead of creating and destroying database connections for each user request.
Configuring Connection Pooling with HikariCP
To configure HikariCP as the connection pool in a Spring Boot application, the following dependency can be added to the pom.xml
:
<dependency>
<groupId>com.zaxxer</groupId>
<artifactId>HikariCP</artifactId>
</dependency>
Additionally, the pool properties can be configured in the application.properties
file:
spring.datasource.hikari.maximum-pool-size=10
spring.datasource.hikari.connection-timeout=30000
By efficiently configuring the connection pool, developers can manage database connections effectively and enhance the responsiveness of Spring Data JPA applications.
Key Takeaways
In conclusion, optimizing the performance of Spring Data JPA applications with MySQL involves various strategies such as efficient entity design, fetching strategies, indexing, query optimization, and connection pooling. By implementing these best practices, developers can achieve enhanced data access performance, minimize latency, and deliver a highly responsive application experience.
By understanding the intricacies of Spring Data JPA and MySQL, developers can drive significant improvements in application performance, enabling their systems to handle large workloads and scaling efficiently.
Incorporating these best practices into Spring Data JPA applications will not only lead to improved performance but also set the foundation for a robust and scalable application architecture.
Optimizing the performance of Spring Data JPA applications with MySQL is an ongoing process, and developers should continuously monitor and fine-tune their database interactions to ensure optimal performance as the application evolves and scales.
By focusing on efficient entity design, leveraging fetching strategies, optimizing queries, and effectively managing database connections, developers can unlock the full potential of their Spring Data JPA applications with MySQL.
For further insights into Spring Data JPA optimization and MySQL performance tuning, refer to the following resources:
- Spring Data JPA Documentation
- MySQL Performance Tuning and Optimization
Incorporating these best practices and continued learning will enable developers to build high-performance Spring Data JPA applications that thrive in real-world scenarios.
Remember, the key to effective optimization lies in understanding the interplay between Spring Data JPA and MySQL and implementing targeted strategies to address performance challenges.