Securing Mobile Apps with OIDC in Ionic
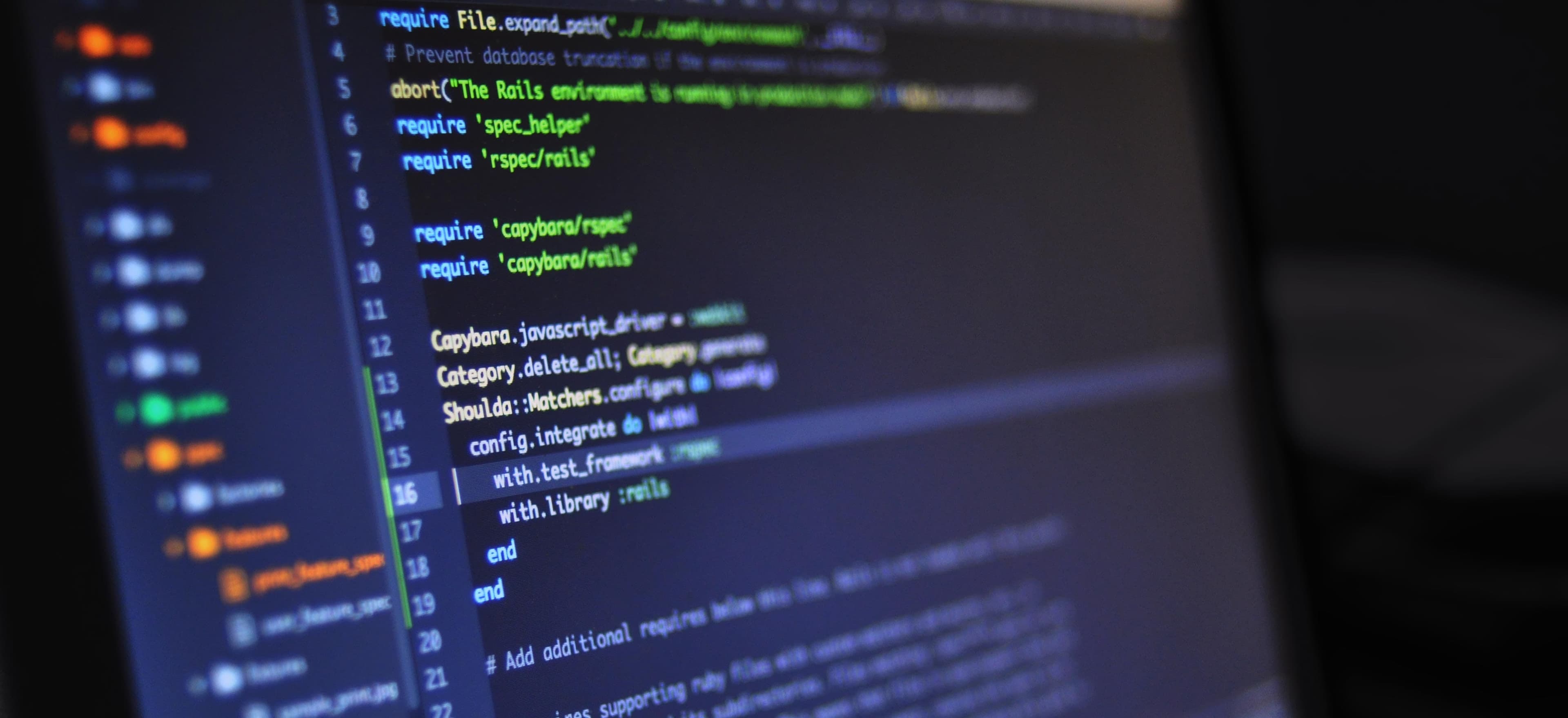
- Published on
Securing Mobile Apps with OpenID Connect (OIDC) in Ionic
In the digital age, mobile applications have become an integral part of our daily lives. As the usage of mobile apps continues to soar, the need for ensuring the security of these apps has become paramount. OpenID Connect (OIDC) has emerged as a powerful authentication and authorization protocol that can be leveraged to secure mobile apps. This blog post will delve into securing mobile apps with OIDC in the context of Ionic, a popular framework for building cross-platform mobile applications using web technologies.
Understanding OpenID Connect (OIDC)
OIDC is an identity layer built on top of the OAuth 2.0 protocol. It provides a secure way for clients to verify the identity of end-users based on the authentication performed by an authorization server. OIDC introduces the concept of identity tokens and UserInfo endpoint, allowing clients to obtain information about the authenticated user. By leveraging OIDC, mobile app developers can implement robust authentication and authorization mechanisms, enhancing the overall security posture of their applications.
Why OIDC in Mobile Apps?
Mobile apps often deal with sensitive user data and perform actions on behalf of the user. As such, ensuring that the user accessing the app is indeed who they claim to be is crucial. OIDC provides a standardized and secure way to accomplish this, offering features such as single sign-on, token-based authentication, and access control. By integrating OIDC into mobile apps, developers can mitigate the risks associated with unauthorized access and data breaches.
Securing Ionic Mobile Apps with OIDC
Ionic provides a comprehensive platform for building high-quality mobile apps using familiar web technologies such as HTML, CSS, and JavaScript. By incorporating OIDC into Ionic apps, developers can implement robust authentication mechanisms and ensure that user identities are verifiable. Let's explore the steps to integrate OIDC into an Ionic mobile app.
Step 1: Setting Up the OIDC Provider
Before integrating OIDC into an Ionic app, developers need to set up an OIDC provider. There are several OIDC providers available, such as Okta, Auth0, and Keycloak, that offer comprehensive identity management solutions. For the purpose of this demonstration, let's consider using Okta as the OIDC provider.
Step 2: Configuring OIDC in Ionic App
In the Ionic app, developers need to configure the OIDC settings such as client ID, client secret, authorization endpoint, token endpoint, and redirect URI. This configuration allows the app to communicate with the OIDC provider for authentication and authorization purposes. The @ionic-native/app-auth
plugin can be utilized to handle the OIDC workflow within the Ionic app.
import { AppAuth } from '@ionic-native/app-auth/ngx';
...
constructor(private appAuth: AppAuth) {}
...
// OIDC configuration
const config = {
clientId: 'YOUR_CLIENT_ID',
issuer: 'YOUR_ISSUER_URL',
redirectUrl: 'YOUR_REDIRECT_URI',
scopes: 'openid profile email',
tokenValidationHandler: HttpTokenValidationHandler
};
// Perform OIDC authentication
this.appAuth
.signIn(config)
.then(response => {
// Handle successful authentication
})
.catch(error => {
// Handle authentication failure
});
In the above code snippet, the AppAuth
plugin is used to initiate the OIDC authentication process. The clientId
, issuer
, redirectUrl
, and scopes
are configured to establish the connection with the OIDC provider. Upon successful authentication, the app receives an identity token and an access token, which can be used to validate the user's identity and access protected resources.
Step 3: Accessing Protected Resources
Once the user is authenticated through OIDC, the app may need to access protected resources, such as user information or secure APIs. The obtained access token can be included in the HTTP requests to the resources, allowing the app to perform authorized actions on behalf of the user.
import { HttpClient, HttpHeaders } from '@angular/common/http';
...
// Include access token in HTTP request
const headers = new HttpHeaders({
'Authorization': 'Bearer ' + accessToken
});
// Access protected resource
this.http.get('https://api.example.com/protected-resource', { headers })
.subscribe(data => {
// Handle the retrieved data
});
In the above code snippet, the access token obtained through OIDC authentication is included in the HTTP request headers. This ensures that the app is authorized to access the protected resource, and the server can validate the token to authorize the requested action.
Closing Remarks
Incorporating OIDC into Ionic mobile apps offers a robust and standardized approach to securing user authentication and authorization. By following the steps outlined in this post, developers can enhance the security of their mobile apps while providing a seamless and secure user experience. Leveraging OIDC in combination with the powerful capabilities of Ionic empowers developers to build secure and trusted mobile applications in today's digital landscape.
By adopting OIDC for mobile app security, developers can instill confidence in their users, mitigate the risks of unauthorized access, and safeguard sensitive user data. With the continuous evolution of mobile app technologies, the integration of OIDC stands as a fundamental requirement for ensuring the security and trustworthiness of mobile applications.
References
In conclusion, as the prevalence of mobile apps continues to rise, it is essential to prioritize security and user trust. Integrating OIDC into Ionic mobile apps offers a potent solution to address these concerns, providing a standardized and robust framework for authentication and authorization. By following the principles of OIDC and harnessing the capabilities of the Ionic framework, developers can build mobile apps that not only deliver exceptional user experiences but also uphold the highest standards of security and privacy.