Overcoming Common Challenges in JavaServer Faces (JSF)
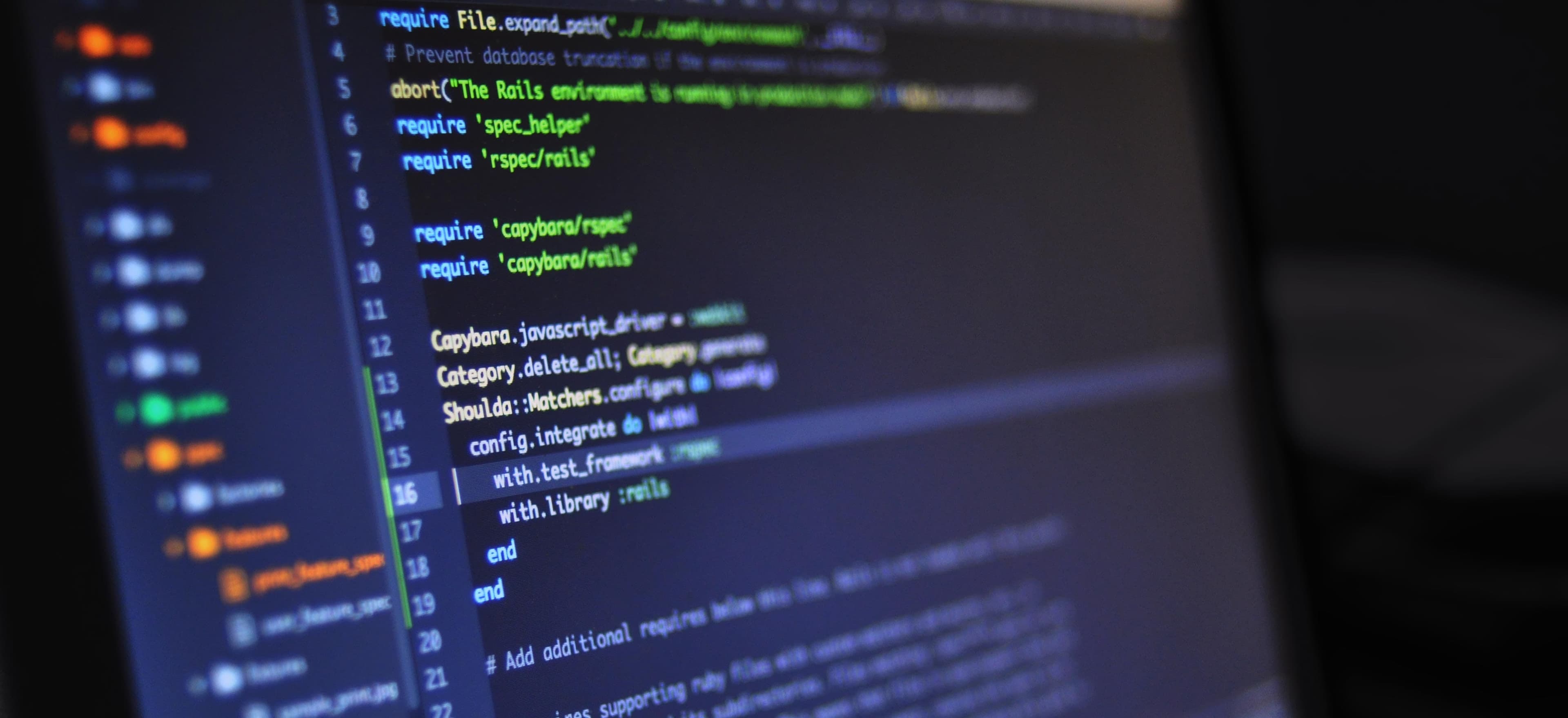
- Published on
Overcoming Common Challenges in JavaServer Faces (JSF)
JavaServer Faces (JSF) is a powerful framework for building web applications in Java. While JSF provides a robust set of tools for creating user interfaces, developers often face challenges when working with it. In this post, we'll explore some common issues encountered when using JSF and discuss strategies for overcoming them.
1. Understanding the JSF Lifecycle
One of the fundamental challenges in JSF development is understanding the lifecycle of a JSF application. The lifecycle encompasses the various phases from the initial request to the final response, and understanding it is crucial for managing component state and handling user input effectively.
For example, when a user interacts with a JSF page, the framework goes through a series of phases such as Restore View, Apply Request Values, Process Validations, Update Model Values, Invoke Application, and Render Response. Each phase has its own significance and understanding this lifecycle is essential for writing efficient JSF applications.
// Example of JSF lifecycle phases
@ManagedBean
public class UserBean {
private String username;
public String submit() {
// Process user input
return "result";
}
}
Developers should familiarize themselves with the JSF lifecycle as it forms the backbone of JSF development.
2. Managing Component IDs
JSF dynamically generates IDs for components, which can lead to challenges when referencing these components in client-side JavaScript or CSS. This can be especially troublesome when working with complex component trees or when integrating third-party libraries that require specific IDs for interaction.
To address this challenge, developers can use the prependId
attribute in the h:form
tag to prepend the parent form's ID to all child component IDs. Additionally, libraries such as PrimeFaces provide a widgetVar
attribute to assign a specific client-side identifier to components.
<!-- Using prependId attribute to manage component IDs -->
<h:form prependId="false">
<h:inputText id="username" />
<h:commandButton id="submitBtn" />
</h:form>
By understanding how to manage component IDs, developers can ensure seamless integration with client-side technologies.
3. Handling Navigation
Navigation in JSF is often a pain point for developers, especially when dealing with complex navigation rules and conditional redirects. Understanding the navigation model in JSF and effectively managing page transitions is crucial for building a smooth user experience.
JSF offers navigation handling through XML navigation rules or annotations (@ManagedBean
and @ManagedProperty
). Additionally, the <h:link>
and <h:button>
tags provide ways to create links and buttons for navigation within JSF applications.
// Example of conditional navigation in JSF
public String submit() {
if (isValidUser) {
return "success";
} else {
return "error";
}
}
By mastering navigation handling, developers can ensure that users can seamlessly move through an application's different views.
4. Validation and Error Handling
Validating user input and effectively handling errors are essential aspects of building robust web applications. In JSF, validating user input and providing meaningful error messages can be challenging, especially when working with complex form submissions.
JSF provides a rich set of validation tags such as <f:validateLength>
, <f:validateRegex>
, and managing error messages using <h:message>
and <h:messages>
. Additionally, custom validation logic can be implemented by creating custom validators and attaching them to input components.
<!-- Example of input validation and error handling -->
<h:inputText id="username">
<f:validateLength minimum="3" maximum="20" />
</h:inputText>
<h:message for="username" />
Effective validation and error handling are crucial for enhancing the user experience and maintaining data integrity.
5. Managing State and Performance
As JSF is component-based, managing the state of components and ensuring optimal performance can be a challenge, especially in applications with complex views and large component trees.
To address this, developers can employ strategies such as optimizing managed bean scopes, using AJAX to update components selectively, and implementing client-side validation to reduce server round-trips. Additionally, JSF libraries like OmniFaces provide utilities for better state saving.
// Example of using AJAX for partial component updates
<h:commandButton value="Update" action="#{userBean.update}">
<f:ajax render="outputPanel" />
</h:commandButton>
<h:outputPanel id="outputPanel">
<!-- Content to be updated -->
</h:outputPanel>
By effectively managing state and focusing on performance optimizations, developers can ensure responsive and efficient JSF applications.
Lessons Learned
JavaServer Faces (JSF) is a powerful framework for building web applications in Java, but it comes with its own set of challenges. By understanding and addressing common issues related to the JSF lifecycle, component IDs, navigation, validation, error handling, and performance, developers can build robust and responsive web applications with JSF. Mastering these challenges will enable developers to harness the full potential of JSF and deliver exceptional user experiences.
In conclusion, despite its challenges, JSF remains a viable option for building Java web applications, and by overcoming these obstacles, developers can fully leverage its capabilities for creating feature-rich and interactive web applications.
By addressing these challenges head-on, developers can unlock the full potential of JSF and deliver exceptional web applications with a remarkable user experience.
References:
- JSF Lifecycle
- JSF Component IDs
- JSF Navigation
- JSF Validation
- JSF State Management
Checkout our other articles