Understanding Type Functions in Ceylon: A Powerful Tool for Kotlin Developers
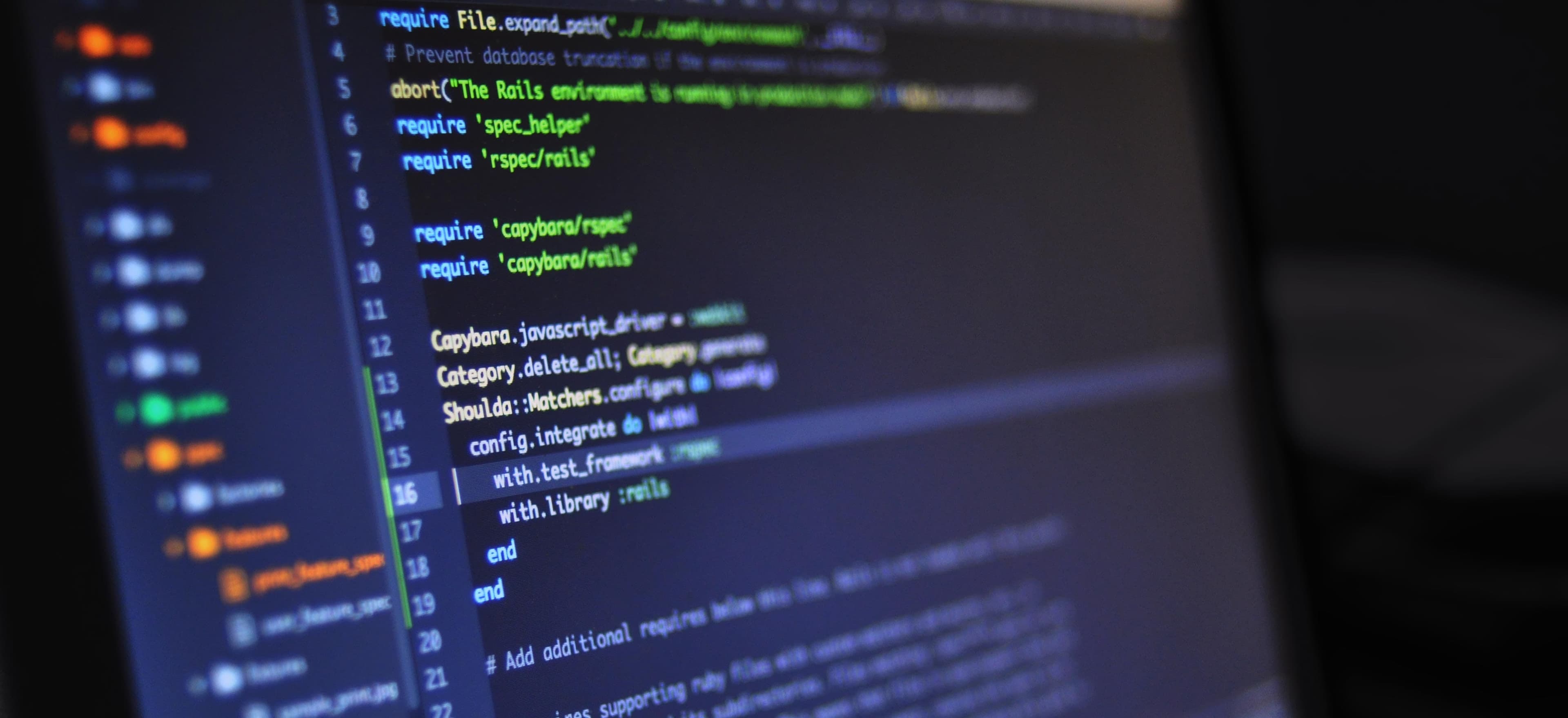
- Published on
Understanding Type Functions in Ceylon: A Powerful Tool for Kotlin Developers
If you're a Kotlin developer looking to extend your understanding of type functions, you'll be pleased to know that Ceylon offers a powerful toolset for implementing and utilizing type functions. In this article, we'll delve into the concept of type functions, explore their implementation in Ceylon, and draw comparisons with similar features in Kotlin.
What are Type Functions?
Type functions, also known as kind polymorphism, are an essential aspect of programming languages that support a high level of type abstraction. They enable developers to define functions at the type level, allowing for manipulation and transformation of types themselves. This level of abstraction is particularly useful in scenarios where operations need to be performed on types to produce new types, effectively allowing for the creation of generic type-level computations.
In languages like Kotlin, type functions can be implemented using higher kinded types and generic constraints. However, Ceylon takes a more explicit and direct approach to type functions, providing a distinct syntax and feature set for defining and working with type functions.
Implementing Type Functions in Ceylon
In Ceylon, type functions are declared using the type
keyword, followed by the name of the type function and its parameterized input types. Let's take a look at a simple example of a type function that applies a generic transformation to a given type:
type Transformer<Type, Result>
given Type satisfies Object {
shared formal Result transform(Type input);
}
In this example, we've defined a type function called Transformer
that takes two input types, Type
and Result
, and declares a transform
function that accepts an input of type Type
and produces a result of type Result
. The given
constraint ensures that the Type
parameter satisfies the Object
type.
To use the Transformer
type function, we can create concrete implementations that specify the actual transformation logic for specific types:
class StringLengthTransformer()
satisfies Transformer<String, Integer> {
shared actual Integer transform(String input) {
return input.size;
}
}
In this example, we've implemented a StringLengthTransformer
that utilizes the Transformer
type function to specify that it operates on String
types and produces Integer
results by returning the length of the input string.
Comparing with Kotlin's Type Functions
In Kotlin, type functions are typically implemented using higher kinded types and generic constraints. Let's consider the equivalent implementation of the Transformer
type function and its concrete implementation in Kotlin:
interface Transformer<Type, Result> {
fun transform(input: Type): Result
}
class StringLengthTransformer : Transformer<String, Int> {
override fun transform(input: String): Int {
return input.length
}
}
In this Kotlin example, we define an interface Transformer
with a type function transform
and implement a StringLengthTransformer
that provides the specific transformation logic for strings.
While the implementation in Kotlin achieves the same outcome as the Ceylon example, the syntax and approach differ, reflecting the unique language features and programming paradigms of each language.
Advantages of Type Functions in Ceylon
The explicit syntax and feature set for type functions in Ceylon offer several advantages for developers, particularly in the context of type-level computations and generic type manipulation:
Readability and Clarity
The explicit type
keyword and syntax for defining type functions in Ceylon enhance code readability and clarity, making it easier to understand and reason about type-level operations within the codebase.
Type Safety and Constraints
Ceylon's type system provides strong support for type safety and constraints, ensuring that type functions are used in a consistent and controlled manner, contributing to overall code robustness and correctness.
Seamless Integration with Ceylon's Module System
Type functions seamlessly integrate with Ceylon's module system, allowing for organized and encapsulated management of type-level computations and transformations within the context of modular applications.
Final Considerations
In this article, we've explored the concept of type functions, delved into their implementation in Ceylon, and compared them with similar features in Kotlin. We've seen how Ceylon's explicit syntax and feature set for type functions provide a powerful toolset for developers to perform type-level computations and generic type manipulation.
As a Kotlin developer, understanding type functions in Ceylon can broaden your perspective on type abstraction and manipulation, offering new insights and approaches to type-level programming. By exploring the unique features and benefits of type functions in Ceylon, you can enrich your skill set and enhance your capabilities as a versatile and proficient developer.
For further information on type functions in Ceylon, you can refer to the official Ceylon documentation for comprehensive insights and examples.
Happy coding!