Optimizing Performance with Sorted Sets
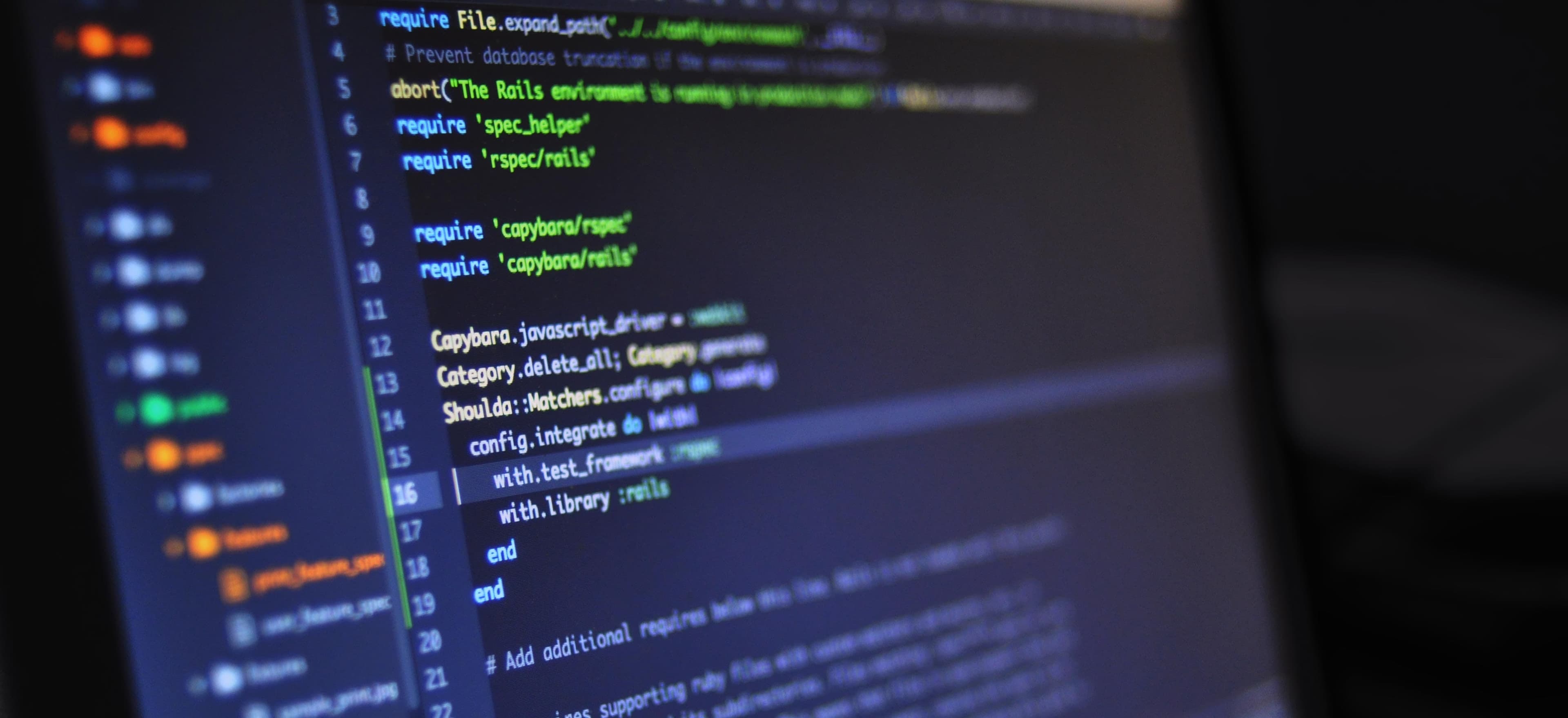
- Published on
How to Optimize Performance with Sorted Sets in Java
In Java, sorted sets are a popular data structure that provides a sorted collection of elements. They are often used to store and manipulate data in a sorted order, which makes them a valuable tool for various programming tasks. However, as with any data structure, it is essential to optimize their performance to ensure efficient operations.
In this article, we will explore how to optimize performance with sorted sets in Java. We will discuss various techniques and best practices that can be used to improve the efficiency of sorted set operations. By the end of this article, you will have a solid understanding of how to leverage sorted sets effectively in your Java applications.
Understanding Sorted Sets
Before we dive into performance optimization, let's first understand what sorted sets are and how they work in Java. Sorted sets, such as TreeSet
and NavigableSet
, are implementations of the SortedSet
interface, which extends the Set
interface. They maintain their elements in a sorted order, which allows for efficient retrieval and manipulation based on the natural ordering of the elements or a custom comparator.
Example: Creating a Sorted Set in Java
// Creating a TreeSet of Strings
SortedSet<String> sortedSet = new TreeSet<>();
sortedSet.add("apple");
sortedSet.add("banana");
sortedSet.add("orange");
System.out.println(sortedSet); // Output: [apple, banana, orange]
In the example above, we create a TreeSet
to store a collection of strings. The elements are automatically sorted in ascending order based on their natural ordering.
Performance Optimization Techniques
Now that we have a basic understanding of sorted sets, let's explore some performance optimization techniques that can be applied to improve the efficiency of sorted set operations in Java.
1. Bulk Operations
Sorted sets provide bulk operations that allow multiple elements to be added, removed, or checked for containment in a single operation. These bulk operations can be more efficient than iterating over individual elements, especially for large sets of data.
// Adding multiple elements to a TreeSet
SortedSet<String> fruits = new TreeSet<>();
List<String> newFruits = Arrays.asList("mango", "pear", "grape");
fruits.addAll(newFruits);
By using the addAll
method, we can add multiple elements to the sorted set in a single operation, which can be more efficient than adding each element individually.
2. Custom Comparators
In some cases, the natural ordering of elements may not suit the specific requirements of a sorted set. By providing a custom comparator, we can define a different ordering that better aligns with the application's needs.
// Creating a TreeSet with a custom comparator
SortedSet<String> descendingSet = new TreeSet<>(Collections.reverseOrder());
descendingSet.addAll(fruits);
In the example above, we create a new TreeSet
with a custom comparator to maintain the elements in descending order. This allows us to define a different sorting behavior tailored to our requirements.
3. Immutable Sorted Sets
Immutable sorted sets, such as those provided by the Collections.unmodifiableSortedSet
method, offer the advantage of being inherently thread-safe. This can eliminate the need for synchronization and provide a performance boost in multi-threaded environments.
// Creating an immutable sorted set
SortedSet<String> immutableSet = Collections.unmodifiableSortedSet(sortedSet);
By creating an immutable sorted set, we ensure that the set cannot be modified, making it safe to share across multiple threads without the overhead of synchronization.
4. Subsets and Range Operations
Sorted sets support operations to obtain subsets of the set or elements within a specific range. These operations can be used to efficiently retrieve a portion of the set that meets certain criteria.
// Obtaining a subset of the sorted set
SortedSet<String> subset = fruits.subSet("banana", "mango");
In the example above, we obtain a subset of the fruits
set that includes elements from "banana" (inclusive) to "mango" (exclusive). This allows us to work with a specific range of elements without iterating over the entire set.
5. Data Structures Integration
In some scenarios, integrating sorted sets with other data structures, such as maps or queues, can lead to performance improvements. For example, combining a sorted set with a map can facilitate efficient lookups and retrievals based on the sorted set's ordering.
// Integrating a sorted set with a map for efficient lookups
SortedSet<Integer> scores = new TreeSet<>();
Map<Integer, String> scoreToPlayerMap = new HashMap<>();
// ... (populate the sets and map)
int lowestScore = scores.first();
String lowestScoringPlayer = scoreToPlayerMap.get(lowestScore);
By integrating a sorted set with a map, we can efficiently retrieve elements based on their ordering, which can be beneficial for applications that require sorting and lookups.
Final Considerations
In this article, we have explored various techniques for optimizing performance with sorted sets in Java. By leveraging bulk operations, custom comparators, immutable sets, subset operations, and data structure integration, we can enhance the efficiency of sorted set operations in our applications. It is essential to carefully consider the specific requirements of the application and choose the appropriate optimization techniques to ensure optimal performance.
By implementing these optimization strategies, you can effectively harness the power of sorted sets in Java and build high-performance applications that require efficient sorting and manipulation of data.
To further enhance your understanding of sorted sets and performance optimization in Java, consider exploring the official documentation for SortedSet and TreeSet.
Remember, optimization is a continuous process, and it's crucial to profile your code and measure the impact of optimizations to ensure they align with your performance goals.