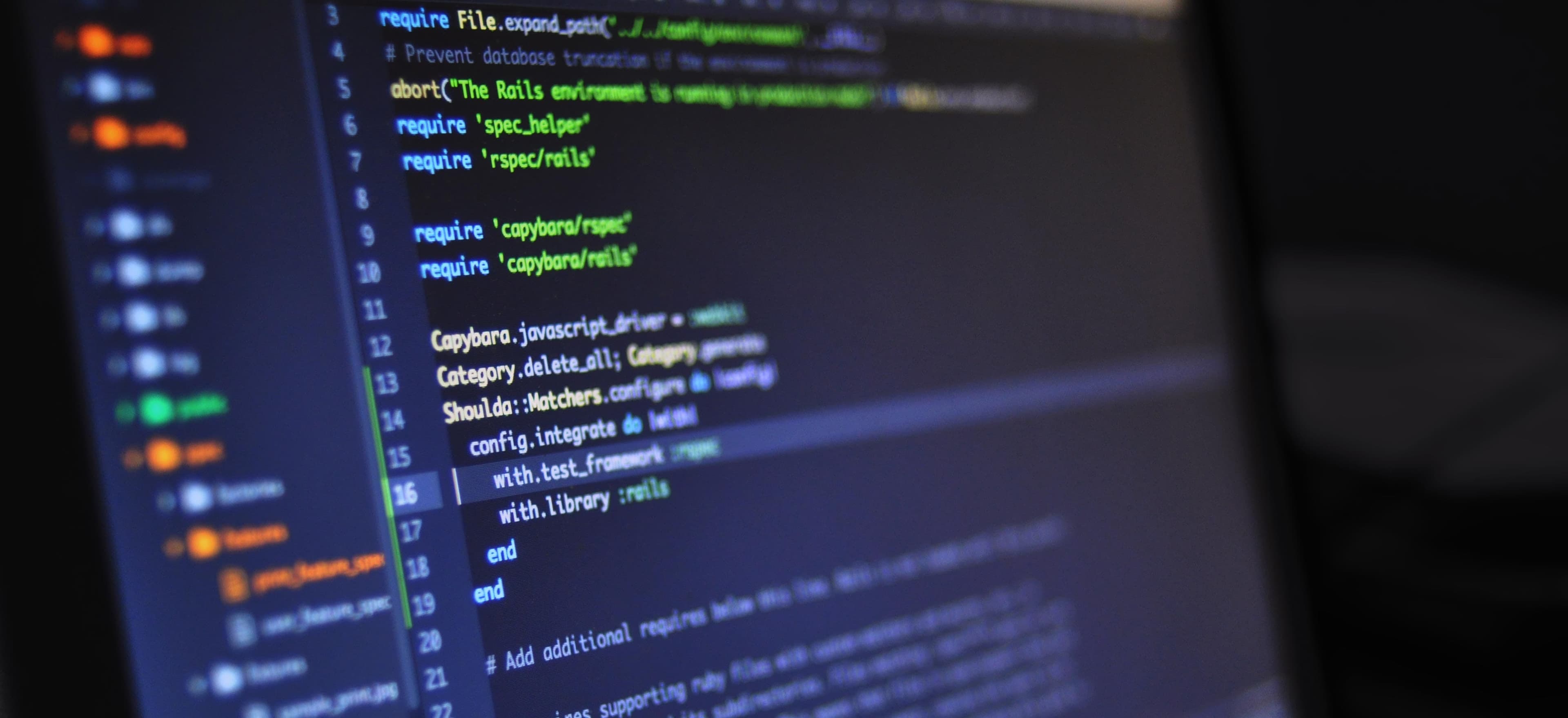
- Published on
Building a "Hello World" Application in Vaadin Dukescript
In this tutorial, we will walk through the process of creating a simple "Hello World" application using Vaadin Dukescript. Vaadin Dukescript is a framework that allows you to build web applications in Java, leveraging the power of HTML and JavaScript. By the end of this tutorial, you will have a basic understanding of how to create a Vaadin Dukescript application and run it in your browser.
Getting Started
Before we begin, make sure you have the following installed:
- Java Development Kit (JDK)
- Maven
To create a new Vaadin Dukescript application, we will use Maven to generate the project structure for us.
Open your terminal and run the following command:
mvn archetype:generate -DarchetypeGroupId=com.dukescript.archetype -DarchetypeArtifactId=knockout4j-archetype -DarchetypeCatalog=http://dukescript.com/maven2
This Maven command uses the knockout4j-archetype
archetype provided by Vaadin Dukescript to generate the project structure for our application.
Project Structure
Once the project is generated, you will see a new directory with the name of your project. Inside, you will find the following structure:
hello-world-app
├── pom.xml
├── src
| └── main
| └── java
| └── com
| └── example
| └── HelloWorldApp.java
Our main Java file, HelloWorldApp.java
, is located inside the src/main/java/com/example
directory. This is where we will write our "Hello World" application code using Vaadin Dukescript.
Writing the Application Code
Open HelloWorldApp.java
with your favorite text editor or integrated development environment (IDE). We will write the code for our "Hello World" application inside this file.
package com.example;
import net.java.html.json.Function;
import net.java.html.json.Model;
import net.java.html.json.Property;
@Model(className = "HelloWorldModel", properties = {
@Property(name = "message", type = String.class),
})
final class HelloWorldApp {
@Function
static void sayHello(HelloWorldModel model) {
model.setMessage("Hello, World!");
}
public static void main(String[] args) throws Exception {
HelloWorldModel model = new HelloWorldModel();
model.applyBindings();
}
}
In this code snippet, we define a model class HelloWorldModel
with a single property message
. We then create a function sayHello
that sets the message
property to "Hello, World!". Finally, in the main
method, we create an instance of HelloWorldModel
and apply the bindings.
Running the Application
To run the application, navigate to the root of your project in the terminal and execute the following Maven command:
mvn test
This command will compile and run the application, and you will see the output "Hello, World!" displayed in your terminal.
Viewing the Application in the Browser
To view the application in your browser, you can open the index.html
file generated in the project's target
directory. This file contains the necessary HTML and JavaScript to render the application in the browser.
Navigate to the target
directory and open index.html
using your preferred web browser. You will see "Hello, World!" displayed on the page, indicating that your Vaadin Dukescript "Hello World" application is up and running.
Final Thoughts
In this tutorial, we covered the process of creating a simple "Hello World" application using Vaadin Dukescript. We used Maven to generate the project structure, wrote the application code in Java, and ran the application to see the output in the browser.
Vaadin Dukescript provides a powerful way to build web applications using Java, and this "Hello World" example serves as a great starting point for exploring its capabilities further.
Now that you have successfully created and run your first Vaadin Dukescript application, you can explore more complex features and build upon this foundation to create sophisticated web applications in Java.
For further exploration, you can delve into Vaadin Dukescript documentation and experiment with more advanced application development.