Troubleshooting High CPU Usage in JVM
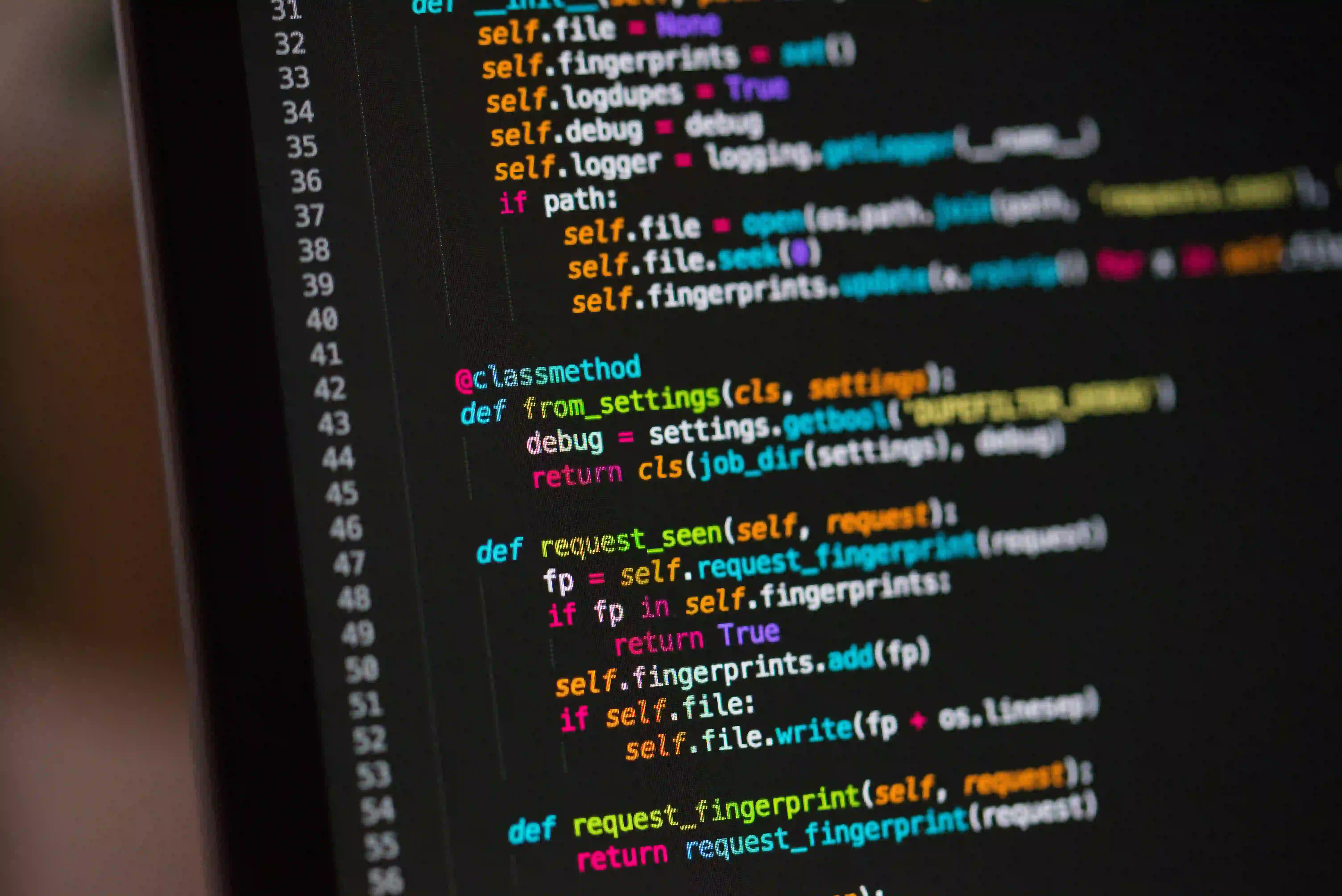
Troubleshooting High CPU Usage in JVM
When it comes to Java applications, dealing with high CPU usage can be a challenging task. High CPU usage can lead to performance issues, degraded user experience, and increased infrastructure costs. In this blog post, we will explore some common causes of high CPU usage in JVM-based applications and discuss troubleshooting techniques to identify and resolve these issues.
Understanding High CPU Usage in JVM
High CPU usage in JVM-based applications can be caused by a variety of factors such as inefficient code, excessive garbage collection, suboptimal thread management, and resource contention. It is essential to understand the underlying reasons for high CPU usage to effectively troubleshoot and resolve the issue.
Profiling the Application
Profiling the application is a crucial step in identifying the source of high CPU usage. Tools like VisualVM, YourKit, and JProfiler can provide valuable insights into the application's behavior, including method-level CPU usage, memory allocation, and thread activity.
Example using VisualVM:
public class ProfilingExample {
public static void main(String[] args) {
// Code to be profiled
}
}
When profiling the application, focus on identifying hotspots in the code, inefficient algorithms, and excessive object creation that contribute to high CPU usage.
Analyzing Garbage Collection
Inefficient garbage collection can significantly impact CPU usage in Java applications. Monitoring and analyzing garbage collection logs can help identify issues such as frequent Full GC, long garbage collection pauses, and excessive memory churn.
Example of GC logging:
java -Xlog:gc:gc.log -jar my-application.jar
Analyzing GC logs can reveal insights into heap utilization, garbage collection pauses, and memory allocation patterns, helping to optimize JVM garbage collection settings for improved performance.
Thread Dump Analysis
Thread management issues, such as thread contention, deadlock, and excessive thread creation, can lead to high CPU usage. Analyzing thread dumps using tools like jstack and Thread Dump Analyzer can help identify problematic threads and synchronization issues.
Example using jstack:
jstack <pid> > threaddump.txt
Analyzing thread dumps can provide visibility into thread states, locks, and potential bottlenecks, enabling effective resolution of thread-related CPU usage issues.
Utilizing Performance Monitoring Tools
Utilizing performance monitoring tools like Java Mission Control (JMC) and Prometheus with Grafana can provide real-time insights into CPU usage, memory consumption, and application metrics. These tools enable proactive monitoring and alerting for abnormal CPU usage patterns.
Optimizing Code and Algorithms
Optimizing code and algorithms is essential for reducing CPU usage in Java applications. Utilize efficient data structures, minimize object creation, and refactor complex algorithms to improve overall performance and reduce CPU overhead.
Example of optimizing code:
// Before optimization
public void processList(List<String> data) {
for (String str : data) {
// Processing logic
}
}
// After optimization
public void processList(List<String> data) {
data.forEach(str -> {
// Optimized processing logic
});
}
Optimizing code can lead to significant reductions in CPU usage and improved application performance.
My Closing Thoughts on the Matter
High CPU usage in JVM-based applications can have detrimental effects on performance and user experience. By leveraging profiling tools, analyzing garbage collection, monitoring thread activity, utilizing performance monitoring tools, and optimizing code, developers can effectively troubleshoot and mitigate high CPU usage issues.
Identifying and resolving high CPU usage in Java applications requires a comprehensive understanding of the application's behavior and performance characteristics. By employing the discussed troubleshooting techniques and best practices, developers can optimize application performance and deliver a seamless user experience.
Remember that troubleshooting high CPU usage in JVM is an iterative process, requiring continuous monitoring and optimization to ensure optimal performance and resource utilization.
For further reading, check out Oracle's Java Performance Tuning Guide and Understanding Java Garbage Collection.