Preventing OutOfMemoryError in Java Applications
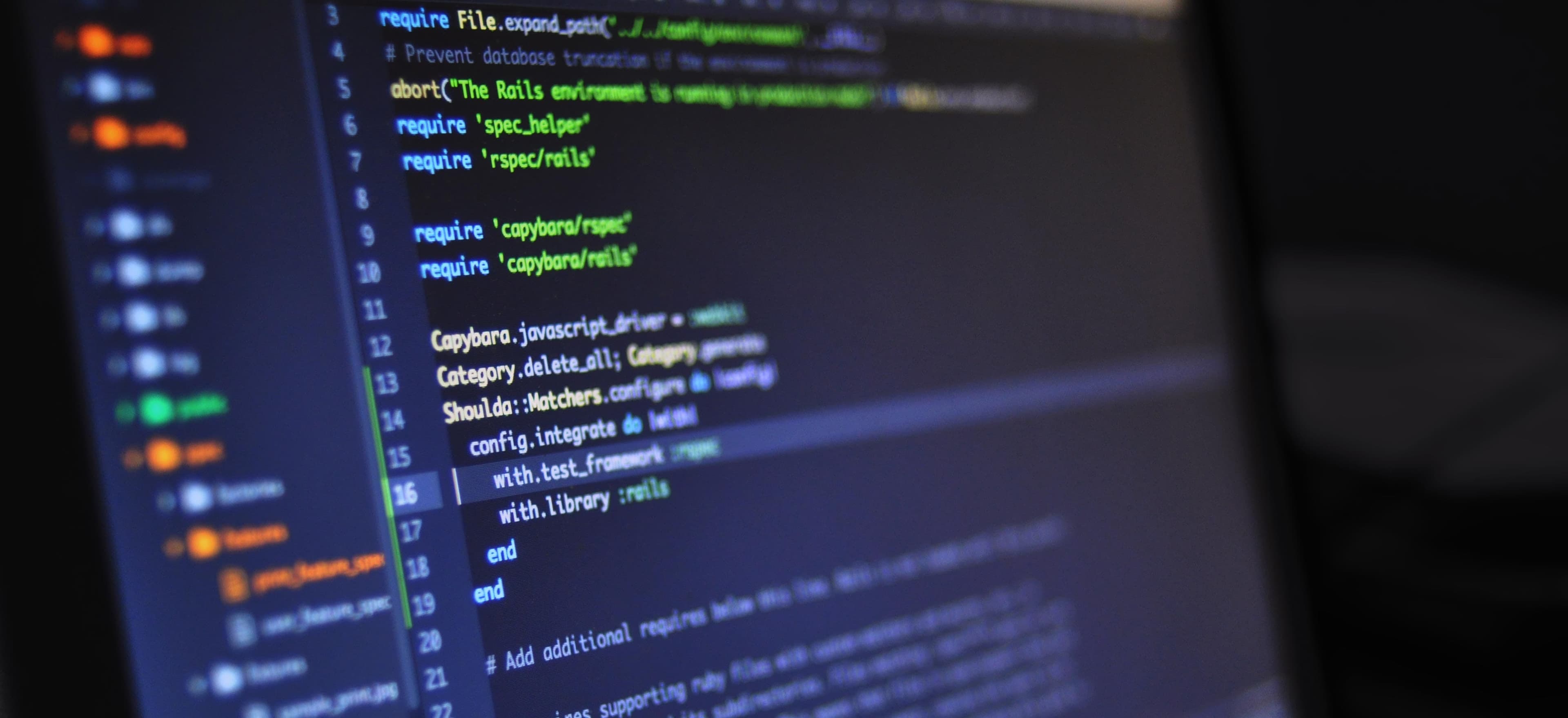
- Published on
Preventing OutOfMemoryError in Java Applications
Java applications are known for their robustness and reliability, but occasionally they can encounter memory-related issues, such as OutOfMemoryError
. This error occurs when the Java Virtual Machine (JVM) runs out of memory. While this error can be caused by a variety of factors, such as large heap sizes, memory leaks, and inefficient coding practices, there are several techniques and best practices that can help prevent OutOfMemoryError
from occurring.
Understanding OutOfMemoryError
Before delving into prevention strategies, it's essential to understand the different scenarios in which OutOfMemoryError
may occur.
Types of OutOfMemoryError
- Java Heap Space: This error occurs when the JVM cannot allocate an object in the Java heap due to lack of space.
- Java Metaspace: In Java 8 and later, the
OutOfMemoryError
can occur if the Metaspace is full and cannot be expanded further to accommodate new classes and metadata. - PermGen Space: In older versions of Java,
OutOfMemoryError
can happen when the permanent generation space is exhausted.
Prevention Strategies
1. Efficient Memory Management
Code Optimization: Review and optimize the code to eliminate memory leaks, unnecessary object creation, and inefficient data structures. This can involve using appropriate data structures, avoiding unnecessary object creation, and ensuring timely release of resources.
Use of Data Structures: Choose the appropriate data structure for the specific use case. For example, using a HashSet
instead of an ArrayList
can minimize memory usage when dealing with a large set of unique elements.
Set<String> uniqueElements = new HashSet<>();
2. Monitoring and Profiling
Memory Profiling Tools: Utilize memory profiling tools like VisualVM, Java Mission Control, or YourKit to monitor memory usage, identify memory leaks, and analyze memory consumption patterns. These tools provide insights into heap memory, object creation, and garbage collection.
3. JVM Configuration
Heap Size: Adjust the heap size based on the application's memory requirements. This can be done using the -Xms
(initial heap size) and -Xmx
(maximum heap size) JVM arguments.
java -Xms512m -Xmx1024m MyApp
Garbage Collection (GC) Tuning: Fine-tune garbage collection settings to match the application's behavior and memory needs. This involves choosing the appropriate garbage collection algorithm and adjusting related parameters.
4. Memory Leaks Detection
Profiling and Analysis: Regularly analyze heap dumps to detect memory leaks and inefficient memory usage. Tools like Eclipse Memory Analyzer (MAT) can be used to analyze heap dumps and identify potential memory leaks.
5. Proper Resource Handling
Closing Resources: Always close resources (file handles, database connections, etc.) after their use to ensure timely release of system resources. Utilize try-with-resources for automatic resource management.
try (BufferedReader reader = new BufferedReader(new FileReader("file.txt"))) {
// Read file content
} catch (IOException e) {
// Handle exception
}
6. Efficient Data Processing
Chunking: When processing large datasets, consider using chunk-based processing to avoid loading the entire dataset into memory at once. This can be achieved using streaming APIs or batch processing techniques.
// Using Java Streams to process data in chunks
List<Data> chunk = dataList.stream()
.skip(startIndex)
.limit(chunkSize)
.collect(Collectors.toList());
7. Utilizing Soft References
SoftReference Class: In scenarios where memory resources are not critical, utilizing SoftReference
can help prevent OutOfMemoryError
by allowing the garbage collector to clear objects held only by soft references.
SoftReference<Object> softRef = new SoftReference<>(new Object());
Closing the Chapter
Preventing OutOfMemoryError
in Java applications involves a combination of efficient coding practices, proper memory management, monitoring, and tuning JVM settings. By adhering to these preventive strategies and keeping an eye on memory usage, developers can create more robust and stable Java applications.
Taking a proactive approach to memory management not only prevents crashes and downtime but also results in more efficient and reliable Java applications.
References:
- Understanding Java OutOfMemoryError
- VisualVM
- Eclipse Memory Analyzer
- SoftReference Class - Java Documentation