Dynamically Generating Criteria Queries in GORM
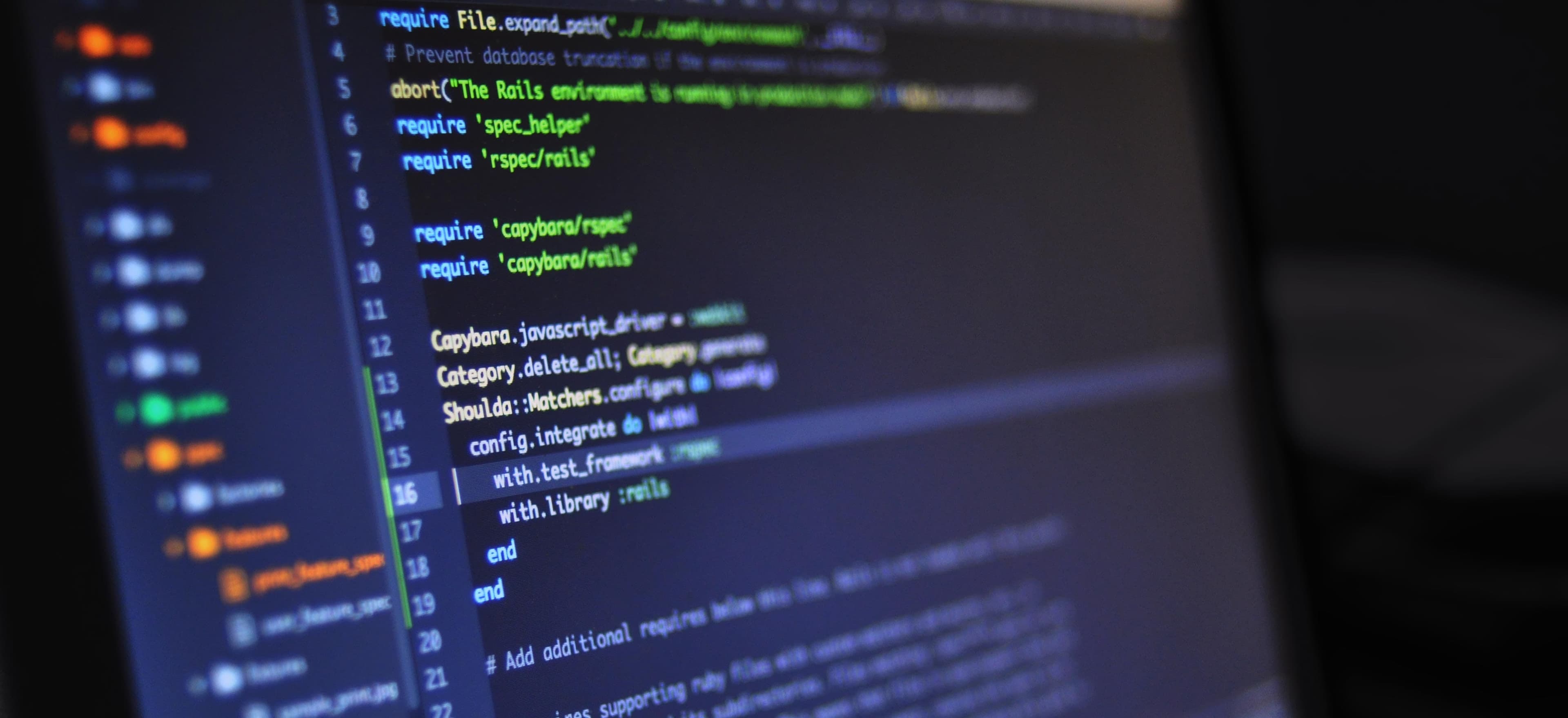
- Published on
Dynamically Generating Criteria Queries in GORM: A Java Developer's Guide
When it comes to working with databases in Java, GORM (Grails Object Relational Mapping) is a widely-used tool for simplifying the process. One powerful feature of GORM is its support for creating dynamic queries using criteria. In this article, we will explore how to dynamically generate criteria queries in GORM, giving you the flexibility to build complex queries at runtime.
What are Criteria Queries?
Before delving into dynamic query generation, it's essential to understand what criteria queries are. In GORM, criteria queries are a programmatic way of building database queries using a fluent API. This approach allows for the construction of queries based on various conditions and criteria, such as filtering, ordering, and pagination.
The Need for Dynamic Query Generation
Sometimes, the requirements of a database query are not known at the time of development. For instance, you may need to allow users to apply multiple filters based on their preferences. In such cases, statically defining all the possible combinations of criteria queries is impractical. This is where dynamic query generation comes into play.
Generating Criteria Queries Dynamically
Let's consider a hypothetical scenario where we have a Product
domain class, and we want to retrieve products based on user-defined filters such as name, price range, and category. We can dynamically construct the criteria query to accommodate these filters.
Step 1: Build the Base Query
To start, we create a base query using the GORM criteria API:
import grails.gorm.DetachedCriteria
DetachedCriteria<Product> criteria = Product.createCriteria()
Now we have a base criteria object that represents the Product
domain class.
Step 2: Adding Dynamic Conditions
Next, we can add dynamic conditions to the criteria based on the user's filters. For example, if the user specifies a product name filter, we can add it to the criteria as follows:
if (productName != null) {
criteria.add(Restrictions.ilike("name", "%" + productName + "%"))
}
In this snippet, productName
is the user-provided value, and we use the ilike
method to perform a case-insensitive, partial match search on the product names. We only add this condition if the productName
is provided.
Step 3: Executing the Query
After applying all the necessary dynamic conditions, we can execute the query to retrieve the results:
List<Product> results = criteria.list {
maxResults(max)
firstResult(offset)
}
Here, we apply additional settings such as maxResults
and firstResult
for pagination. Finally, we obtain the list of products that match the dynamically generated criteria.
Why Use Dynamic Query Generation?
Dynamic query generation offers several advantages. Firstly, it allows for the construction of queries based on user input, providing a more interactive and personalized experience. Secondly, it eliminates the need to write and maintain numerous query methods for different combinations of criteria. This leads to cleaner, more maintainable code.
Additionally, dynamic query generation can enhance performance by enabling the application to dynamically adapt to changing query requirements without the overhead of predefined queries for every possible combination of filters.
Lessons Learned
In this article, we've explored the concept of dynamically generating criteria queries in GORM. We've seen how to build a base query and add dynamic conditions based on user input to create flexible and powerful database queries. By leveraging dynamic query generation, you can cater to a wide range of user requirements while maintaining a clean and efficient codebase.
To dive deeper into GORM and dynamic query generation, check out the Grails documentation here.
Now that you have a solid understanding of dynamic query generation in GORM, it's time to apply these concepts to your own projects and unlock the full potential of flexible and user-driven database queries!