Challenges in Top-Down Web Service Development
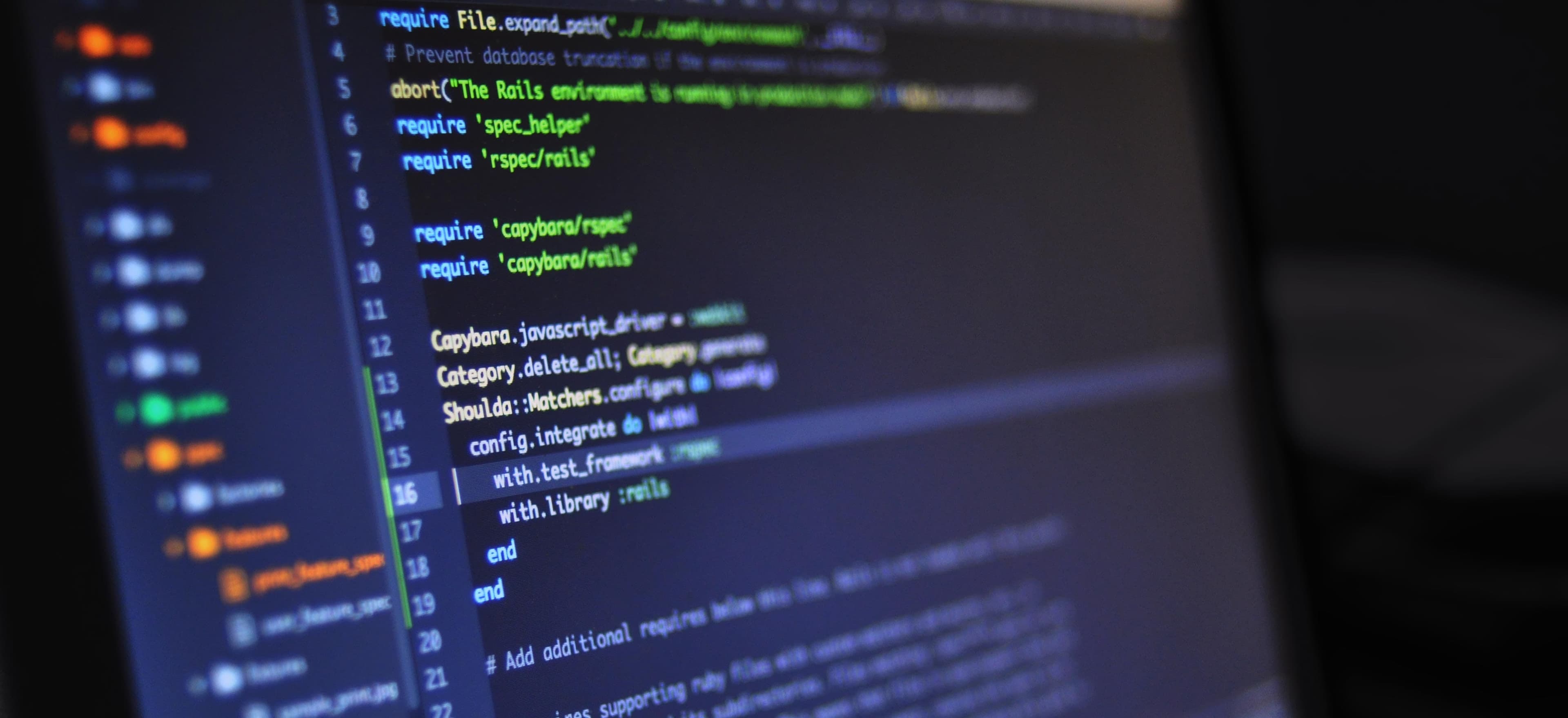
- Published on
Challenges in Top-Down Web Service Development
Developing web services using a top-down approach involves starting with a contract (WSDL) and then implementing this contract. This approach may seem straightforward in theory, but in practice, it comes with its own set of challenges. In this article, we’ll explore the common challenges faced in top-down web service development and how to overcome them using Java.
Understanding Top-Down Web Service Development
Top-down web service development involves creating the contract (WSDL) first and then implementing it. This often involves using tools that generate code from the WSDL, which can be a double-edged sword. While it provides a quick way to get started, it can also lead to unexpected complications and limitations.
Challenges Faced
1. Tight Coupling
When starting with a WSDL contract, there's a risk of tightly coupling the service implementation to this contract. Any changes in the WSDL can ripple through and impact the Java implementation, making maintenance a cumbersome task.
2. Tool Limitations
Using tools to generate code from WSDL can lead to code that is difficult to customize or extend. This can be particularly challenging when requirements evolve beyond the initial contract.
3. Testing Complexities
Testing a top-down web service can be challenging due to its close ties with the WSDL contract. This can lead to difficulties in creating mock services or stubs for testing.
4. Versioning
Versioning a top-down web service, especially when the WSDL contract changes, can be a daunting task. Ensuring backward compatibility while evolving the service can become a major challenge.
Overcoming the Challenges with Java
1. Using Interfaces
In Java, use interfaces to define the contract instead of generating code from WSDL directly. By programming to an interface, you can minimize the impact of WSDL changes on the implementation classes. This promotes looser coupling and better maintainability.
2. Manual Code Generation
Consider manually writing the service implementation classes instead of relying completely on tool-generated code. This provides more control over the codebase and facilitates easier customization and extension as requirements change.
// Example of manually implementing a web service from an interface
public class MyWebService implements MyServiceInterface {
// Implement the methods defined in the interface
}
3. Mocking Frameworks
Utilize Java mocking frameworks such as Mockito or EasyMock to create mock services for testing. This allows for more flexibility in testing the service without being tightly bound to the WSDL contract.
// Example of using Mockito to create a mock service for testing
MyServiceInterface mockService = Mockito.mock(MyServiceInterface.class);
4. Versioning Strategies
Implement versioning strategies such as URI versioning or versioning through request headers to handle changes in the WSDL contract. This enables maintaining backward compatibility while evolving the service.
To Wrap Things Up
Top-down web service development in Java comes with its own set of challenges, but by following best practices and utilizing the features Java offers, these challenges can be effectively mitigated.
By using interfaces, writing manual code when necessary, leveraging mocking frameworks, and implementing versioning strategies, developers can tackle the complexities of top-down web service development and create robust, maintainable web services.