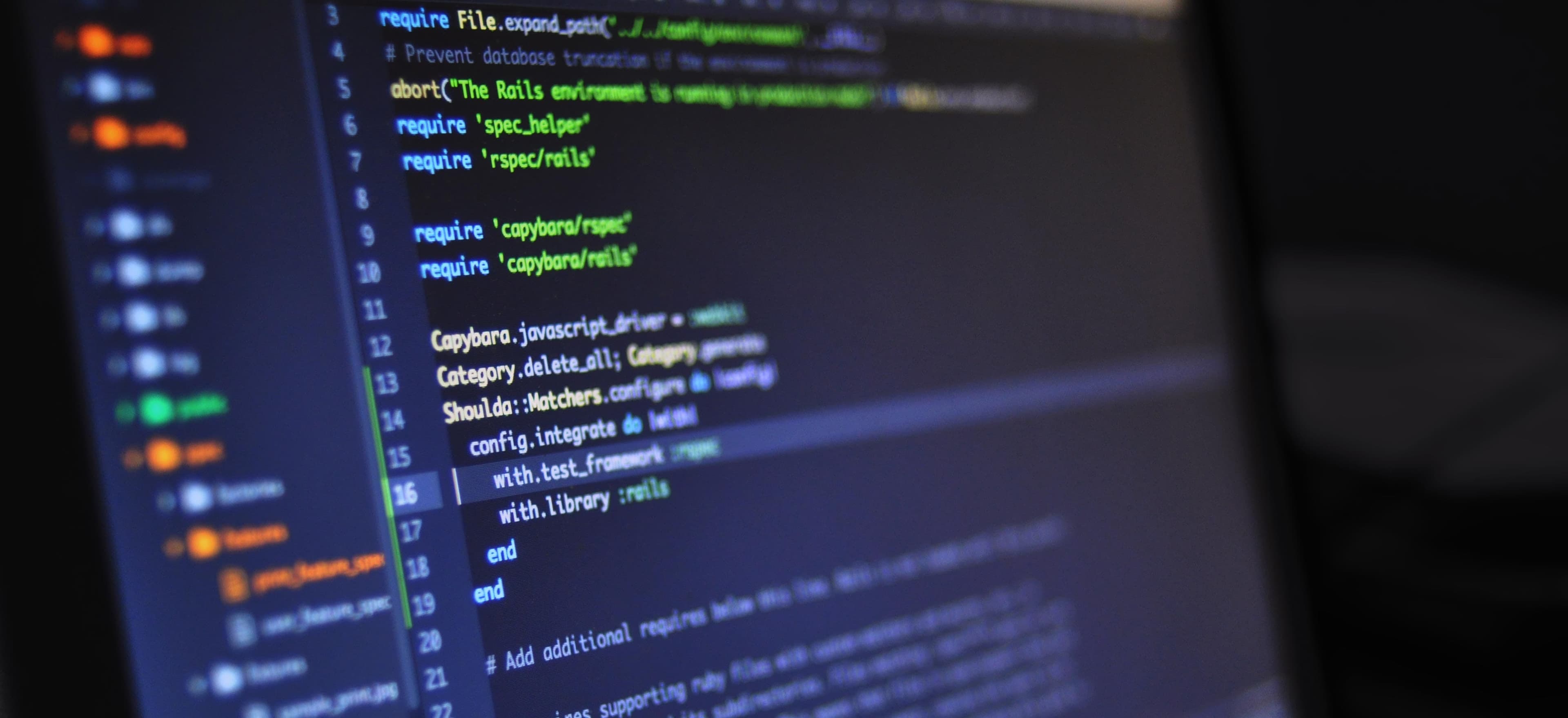
- Published on
Securing DevOps: Effective Authorization Management
In today's fast-paced and dynamic software development environment, DevOps practices have become the cornerstone of efficient and collaborative workflows. However, with the increased emphasis on rapid deployment and continuous integration, security can sometimes take a backseat. In this blog post, we will explore the crucial aspect of authorization management in DevOps and how Java provides robust solutions for effective authorization.
The Significance of Authorization Management
Authorization plays a pivotal role in ensuring that the right individuals or systems have appropriate access to resources within an application. Without effective authorization management, sensitive data and critical functionalities are at risk of being compromised. In the context of DevOps, where multiple teams work collaboratively on a shared codebase and infrastructure, the need for precise authorization mechanisms becomes even more pronounced.
Java and Authorization Management
Java, as a versatile and widely-used programming language, offers a rich set of tools and frameworks for implementing robust authorization management within DevOps processes. From role-based access control to fine-grained permissions, Java provides developers with the building blocks to enforce security measures effectively.
Implementing Role-Based Access Control (RBAC) in Java
Role-based access control is a tried-and-tested approach to authorization, where access decisions are based on the roles that individual users hold within an organization. Java's enterprise frameworks, such as Spring Security, offer seamless support for implementing RBAC. Let's take a look at a simplified example of defining roles and securing a REST endpoint using Spring Security:
@RestController
public class UserController {
@PreAuthorize("hasRole('ADMIN')")
@GetMapping("/users")
public List<User> getAllUsers() {
// Logic to fetch and return all users
}
}
In this snippet, the @PreAuthorize
annotation from Spring Security is used to restrict access to the /users
endpoint to users with the ADMIN
role. This declarative approach simplifies the integration of authorization rules within the codebase, promoting maintainability and clarity.
Fine-Grained Authorization with Java
In addition to traditional RBAC, Java enables developers to implement fine-grained authorization, where access control decisions are made based on specific attributes or conditions. The Java Authorization Contract for Containers (JACC) provides a standard API for implementing fine-grained authorization in Java EE containers.
public class CustomPermissionCheck implements javax.security.jacc.EJBRoleRefPermission {
@Override
public boolean implies(Permission permission) {
// Custom logic to check fine-grained permission
}
}
In this example, a custom permission check class implementing EJBRoleRefPermission
can define intricate authorization logic tailored to the specific requirements of the application. Java's support for fine-grained authorization empowers developers to enforce precise access controls, thereby enhancing the overall security posture of DevOps processes.
Best Practices for Authorization Management in DevOps
While Java equips developers with powerful tools for authorization management, it is essential to adhere to best practices to ensure the effectiveness of these security measures within DevOps environments.
Principle of Least Privilege
Adhering to the principle of least privilege dictates that individuals should only be granted the minimum level of access required to perform their duties. In the context of DevOps, this principle should be diligently applied to ensure that each team member, automated process, or system component has precisely the necessary permissions, mitigating the potential impact of a security breach.
Continuous Monitoring and Logging
Incorporating robust monitoring and logging mechanisms into DevOps processes allows for the continuous observation of authorization events. Java's logging frameworks, such as Log4j or SLF4J, can be leveraged to capture authorization-related activities, providing valuable insights into access patterns and potential anomalies.
Automation of Authorization Policies
With the proliferation of infrastructure as code (IaC) and automated deployment pipelines, the automation of authorization policies becomes imperative. Java's integration with configuration management tools, such as Ansible or Puppet, facilitates the codification and enforcement of authorization policies throughout the DevOps lifecycle.
Key Takeaways
In conclusion, effective authorization management is a critical component of securing DevOps practices, and Java offers a robust toolkit for implementing and enforcing authorization controls. By leveraging Java's support for role-based access control, fine-grained authorization, and adherence to best practices, organizations can fortify their DevOps processes against potential security threats.
Implementing a comprehensive authorization management strategy not only safeguards sensitive resources but also fosters a culture of security awareness within DevOps teams.
For further reading on the topic of DevOps security and authorization management, check out the OWASP DevSecOps initiative and the Java Authorization Overview from Oracle.
Remember, the strength of a DevOps pipeline is only as robust as its security measures. Incorporating effective authorization management is not simply a best practice – it is a necessity in today's threat landscape.