Maximizing Efficiency: Streamlining Java Annotation Processing
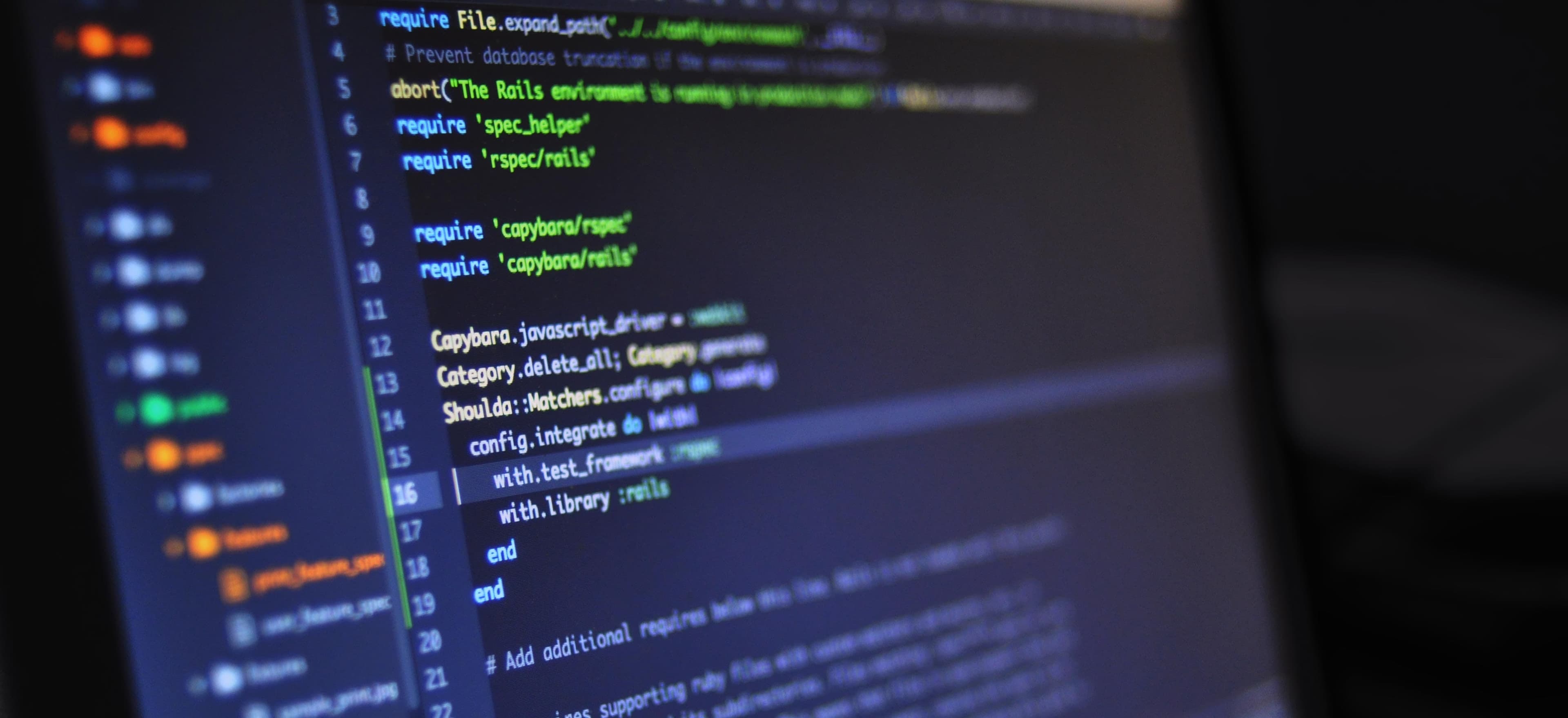
- Published on
In the world of Java development, efficiency is key. As projects grow in size and complexity, it becomes increasingly important to streamline the development process to ensure optimal performance without sacrificing code quality. One area where efficiency can be greatly improved is in Java annotation processing.
What are Java annotations?
Annotations are a form of metadata that provide information about the code they annotate. They can be used to provide instructions to the compiler, to generate code, or to specify run-time behavior. Java annotations are widely used in frameworks like Spring, Hibernate, JUnit, and many others.
The power of Java annotation processing
Annotation processing is a powerful tool that allows developers to analyze and process annotations at compile time. This can be used to generate code, validate classes, or perform other custom operations based on the annotations present in the codebase.
While the standard Java annotation processing API provides a solid foundation for working with annotations, there are a number of best practices and advanced techniques that can significantly improve the efficiency and maintainability of annotation processors.
Leveraging the javax.annotation.processing package
The javax.annotation.processing
package provides the necessary tools for creating annotation processors. The AbstractProcessor
class can be extended to create custom annotation processors. This is the starting point for creating an annotation processor.
Here's an example of a simple annotation processor that validates the usage of a custom annotation:
@SupportedAnnotationTypes("com.example.CustomAnnotation")
@SupportedSourceVersion(SourceVersion.RELEASE_8)
public class CustomAnnotationProcessor extends AbstractProcessor {
@Override
public boolean process(Set<? extends TypeElement> annotations, RoundEnvironment roundEnv) {
// Perform validation and processing here
return true;
}
}
In this example, the CustomAnnotationProcessor
extends AbstractProcessor
and overrides the process
method. The @SupportedAnnotationTypes
annotation specifies which annotations this processor should handle, and @SupportedSourceVersion
specifies the source version this processor supports.
Utilizing processing rounds for incremental processing
One important concept in annotation processing is processing rounds. During each round, the annotation processor is given the opportunity to process the annotations present in the code. By leveraging the concept of rounds, developers can implement incremental processing to only process the annotations that have changed since the last round. This can significantly improve build times for large projects.
Generating code with JavaPoet
In some cases, annotation processing is used to generate code. JavaPoet is a convenient library that can be used to generate Java source code. It provides a fluent API for building Java code and handles the necessary formatting and escaping.
Here's an example of using JavaPoet to generate a simple Java class:
MethodSpec main = MethodSpec.methodBuilder("main")
.addModifiers(Modifier.PUBLIC, Modifier.STATIC)
.returns(void.class)
.addParameter(String[].class, "args")
.addStatement("$T.out.println($S)", System.class, "Hello, World!")
.build();
TypeSpec helloWorld = TypeSpec.classBuilder("HelloWorld")
.addModifiers(Modifier.PUBLIC, Modifier.FINAL)
.addMethod(main)
.build();
JavaFile javaFile = JavaFile.builder("com.example", helloWorld)
.build();
javaFile.writeTo(System.out);
In this example, we use JavaPoet to generate a simple HelloWorld
class with a main
method that prints "Hello, World!" to the console.
Implementing conditional processing with Java 8's javax.lang.model.element.Element
and TypeMirror
Java 8 introduced the javax.lang.model.element.Element
and TypeMirror
interfaces, which provide a more precise way to work with program elements and types during annotation processing. By using these interfaces, developers can implement more complex logic based on the types and elements present in the code, enabling conditional processing and more advanced validation scenarios.
Unit testing annotation processors
Testing annotation processors is crucial to ensure their correctness and reliability. Libraries like javac testing provide utilities for writing unit tests for annotation processors. By writing comprehensive unit tests, developers can catch potential issues early and ensure their annotation processors behave as expected.
Wrapping Up
Efficient annotation processing is crucial for maintaining a smooth and fast development workflow in Java projects. By leveraging best practices, advanced techniques, and relevant libraries, developers can streamline the annotation processing pipeline, thus optimizing the overall development process.
In conclusion, Java annotation processing, when done right, can greatly enhance the development experience, enabling developers to generate code, validate classes, and implement custom operations with ease. By following the best practices outlined in this article, developers can maximize the efficiency of their annotation processing workflow, ultimately leading to more maintainable and performant codebases.