Generating Class Diagrams from Java Code
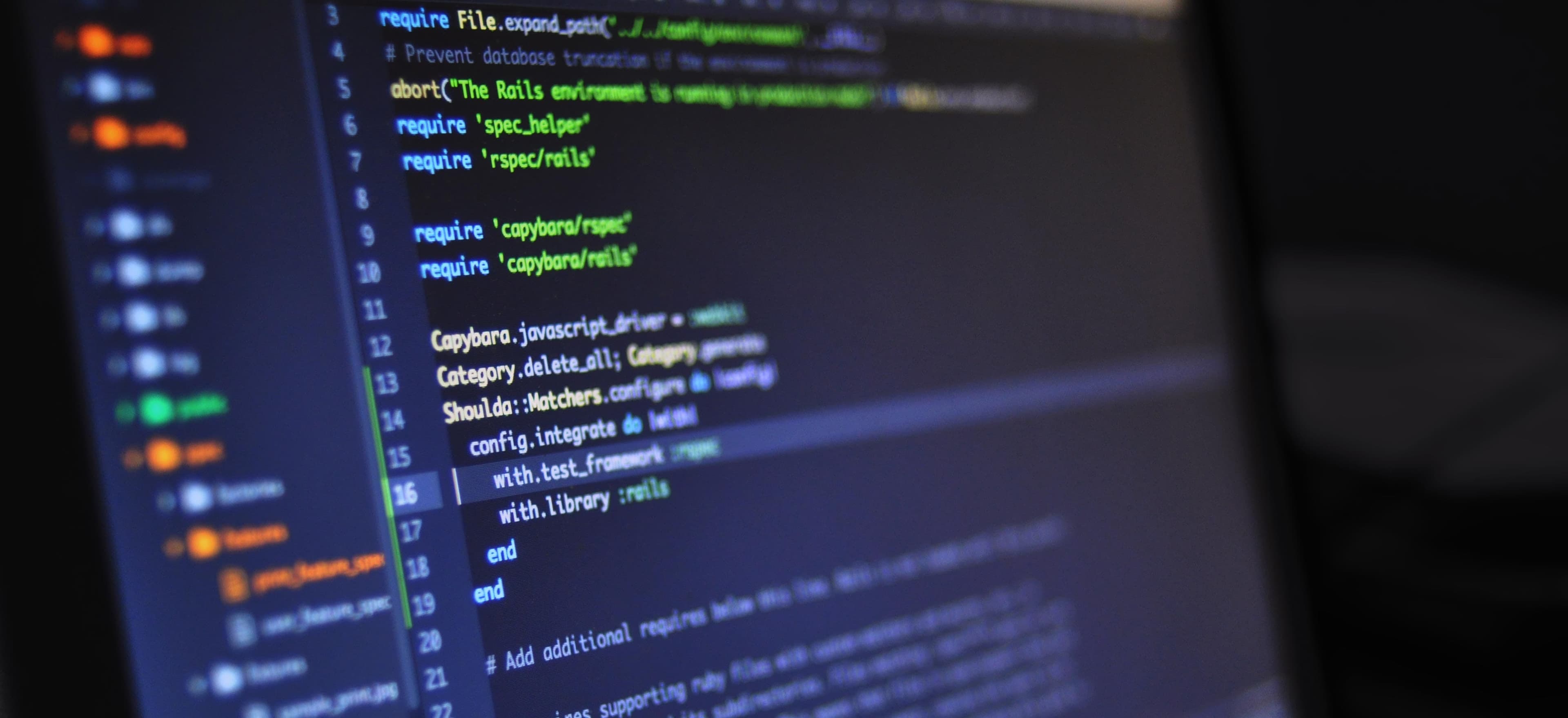
- Published on
Understanding the Importance of Class Diagrams in Java Development
When it comes to Java development, having a clear understanding of the relationships and structure of classes is crucial. This is where class diagrams come into play, providing a visual representation of the classes, interfaces, and their associations within the system. Class diagrams not only aid in comprehending the architecture of a system but also facilitate communication among stakeholders, making them an indispensable tool in software development.
In this blog post, we will delve into the significance of class diagrams in Java development and explore how to generate them from Java code, using popular tools and techniques.
Importance of Class Diagrams
Visualizing Classes and Relationships
Class diagrams offer a way to visualize the classes and their relationships, including inheritance, associations, and dependencies. This visual representation helps developers gain insights into the overall structure of the system, making it easier to comprehend and maintain large codebases.
Designing and Refactoring
In the initial phase of software development, class diagrams aid in designing the system architecture. They provide a blueprint for structuring classes and interactions before diving into the actual implementation. Furthermore, during refactoring, class diagrams serve as a guide for restructuring classes and relationships, ensuring that the changes align with the system's design.
Communication Tool
Class diagrams act as a communication tool among developers, architects, and stakeholders. They enable effective discussion of the system's architecture, making it easier to convey complex relationships and dependencies in a clear, visual manner.
Generating Class Diagrams from Java Code
Now let's explore how we can generate class diagrams from Java code. There are several tools and libraries available to accomplish this task, each offering its own set of features and capabilities. We will focus on using the popular tool, PlantUML, to generate class diagrams from Java code.
PlantUML
PlantUML is a powerful open-source tool that uses simple textual descriptions to generate UML diagrams, including class diagrams. It supports various diagram types and provides a straightforward syntax for specifying the relationships between classes, making it ideal for generating class diagrams from Java code.
Setting Up PlantUML with IntelliJ IDEA
IntelliJ IDEA, a widely used integrated development environment for Java, offers seamless integration with PlantUML through the use of plugins such as "PlantUML integration." After installing the plugin, you can easily create PlantUML files (.puml) and visualize the class diagrams directly within IntelliJ IDEA.
Generating Class Diagrams
Let's dive into generating a class diagram from Java code using PlantUML within IntelliJ IDEA. Consider the following Java classes:
// Car.java
public class Car {
private String make;
private String model;
public Car(String make, String model) {
this.make = make;
this.model = model;
}
public String getMake() {
return make;
}
public String getModel() {
return model;
}
}
// Main.java
public class Main {
public static void main(String[] args) {
Car myCar = new Car("Toyota", "Camry");
System.out.println("My car is a " + myCar.getMake() + " " + myCar.getModel());
}
}
The next step is to create a PlantUML file to represent the class diagram for the Car
and Main
classes. We can use the following PlantUML code to achieve this:
@startuml
class Car {
-make: String
-model: String
+Car(make: String, model: String)
+getMake(): String
+getModel(): String
}
class Main {
+main(String[] args): void
}
Car --|> Main
@enduml
In this PlantUML code, we define the classes Car
and Main
, along with their attributes and methods. Additionally, we specify the relationship between the Car
and Main
classes, indicating that Main
has a dependency on Car
.
By utilizing the PlantUML integration in IntelliJ IDEA, we can visualize the generated class diagram directly within the IDE, providing a clear representation of the classes and their relationships.
A Final Look
In the realm of Java development, class diagrams play a pivotal role in understanding, designing, and communicating the architecture of a system. With tools like PlantUML and seamless integrations with popular IDEs, generating class diagrams from Java code has become more accessible than ever.
Incorporating class diagrams into the development process not only enhances the clarity of system architecture but also fosters effective communication and streamlined design and refactoring efforts. By harnessing the power of visual representation, developers can navigate complex systems with ease, ultimately contributing to the overall success of the software projects.
As you continue your journey in Java development, consider integrating class diagrams into your workflow, and witness the impact they can have on the understanding and evolution of your software systems.