Handling Asynchronous Tasks with Java FutureTask
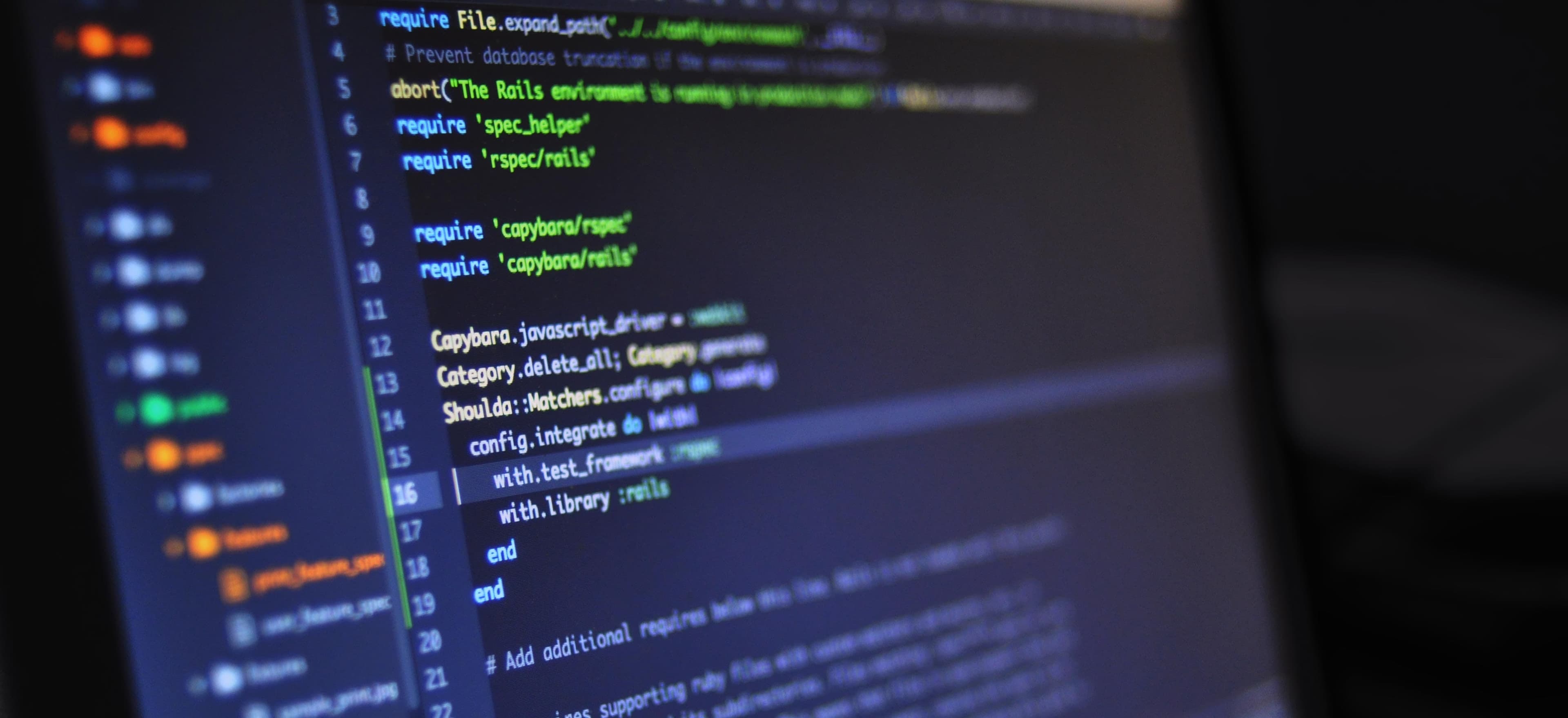
- Published on
Handling Asynchronous Tasks with Java FutureTask
In the world of programming, asynchronous tasks are crucial for building responsive and scalable applications. Java provides several mechanisms for executing tasks asynchronously, and one such mechanism is the FutureTask
class. In this post, we will explore how to use FutureTask
to handle asynchronous tasks in Java.
What is FutureTask?
FutureTask
is a part of the java.util.concurrent
package and represents a computation that will eventually produce a result. It is a convenient way to encapsulate a computation that can be executed asynchronously. The result of the computation can be retrieved using the get
method, which will block until the computation is complete.
Why Use FutureTask?
FutureTask
provides a way to run tasks asynchronously and retrieve their results when needed. It is particularly useful when dealing with long-running operations that should not block the main thread. By using FutureTask
, you can delegate the task to another thread and continue with other operations while waiting for the result.
Creating a FutureTask
To create a FutureTask
, you can either extend the FutureTask
class or pass a Callable
to its constructor. Here's an example of creating a FutureTask
using a Callable
:
import java.util.concurrent.Callable;
import java.util.concurrent.FutureTask;
public class MyTask implements Callable<String> {
@Override
public String call() throws Exception {
// Perform some long-running task
return "Task result";
}
}
public class Main {
public static void main(String[] args) {
Callable<String> callable = new MyTask();
FutureTask<String> futureTask = new FutureTask<>(callable);
// Execute the task in a separate thread
new Thread(futureTask).start();
// Continue with other operations
// Retrieve the result once the task is complete
try {
String result = futureTask.get();
System.out.println("Task result: " + result);
} catch (Exception e) {
// Handle exceptions
}
}
}
In this example, we define a Callable
implementation MyTask
and create a FutureTask
with it. We then execute the FutureTask
in a separate thread and retrieve the result once the task is complete using the get
method.
Handling Task Completion
FutureTask
provides several methods to check the status of the task and handle its completion. The isDone
method can be used to check if the task has completed, and the cancel
method can be used to cancel the task if it has not started.
Here's an example of using isDone
and cancel
with FutureTask
:
// Assume futureTask is already created and executed
if (!futureTask.isDone()) {
// Task is not yet completed
}
// Cancel the task if it has not started
boolean cancelled = futureTask.cancel(false);
By checking the status of the task and potentially cancelling it, you can effectively manage the execution of asynchronous tasks using FutureTask
.
Handling Dependencies Between Tasks
In some cases, you may have tasks that depend on the results of other tasks. FutureTask
allows you to chain tasks together to enforce dependencies. By using the get
method of a FutureTask
within another FutureTask
, you can wait for the result of the first task before executing the dependent task.
Here's an example of chaining FutureTask
instances to handle task dependencies:
FutureTask<Integer> task1 = new FutureTask<>(...);
FutureTask<String> task2 = new FutureTask<>(...);
new Thread(task1).start();
// Waits for the result of task1 before executing task2
task2 = new FutureTask<>(() -> "Result: " + task1.get());
new Thread(task2).start();
In this example, task2
waits for the result of task1
by calling its get
method within its own computation. This allows task2
to depend on the result of task1
before executing its own logic.
Error Handling
When using FutureTask
, it's essential to handle potential exceptions that may occur during the execution of the task. The get
method throws an ExecutionException
if the computation threw an exception, and the cancel
method returns true
if the task was successfully cancelled.
try {
String result = futureTask.get();
System.out.println("Task result: " + result);
} catch (ExecutionException e) {
// Handle exception thrown by the task
} catch (InterruptedException e) {
// Handle interruption of the waiting thread
}
boolean cancelled = futureTask.cancel(false);
if (cancelled) {
// Task was successfully cancelled
}
By catching ExecutionException
and InterruptedException
, you can gracefully handle exceptions and interruptions that may occur during the execution of a FutureTask
.
The Closing Argument
In conclusion, FutureTask
is a powerful tool for handling asynchronous tasks in Java. It allows you to run tasks asynchronously, retrieve their results, manage dependencies between tasks, and handle errors gracefully. By incorporating FutureTask
into your Java applications, you can improve responsiveness and scalability, making your applications more robust and efficient.
Ready to dive deeper into asynchronous programming in Java? Check out this guide for additional insights into Java Future
. Also, don't miss out on exploring CompletableFuture for more advanced asynchronous task handling.
Happy coding!