Effective Logging in Test Automation: A How-To Guide
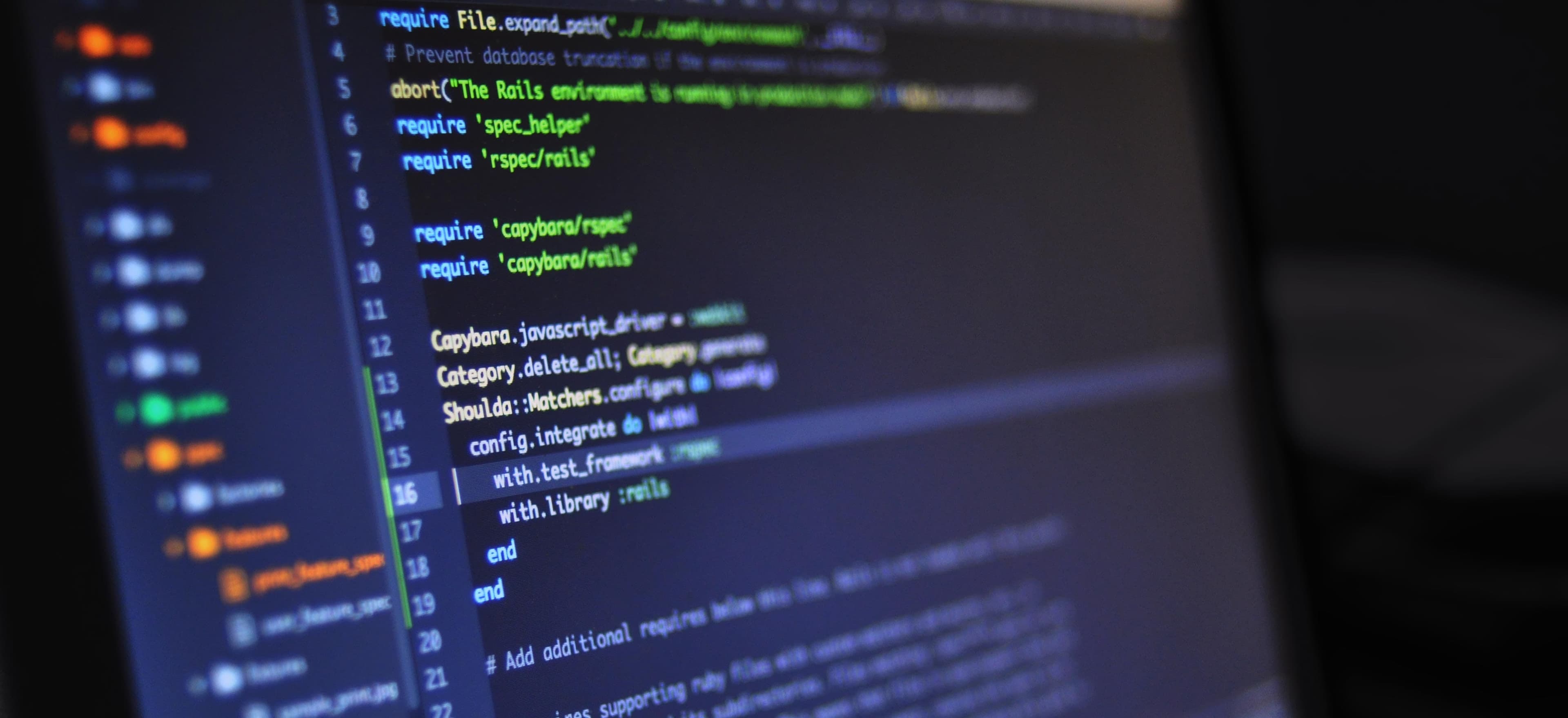
- Published on
Effective Logging in Test Automation: A How-To Guide
In the realm of test automation, logging plays a vital role in providing insight into the execution of test cases, facilitating debugging, and generating reports. Despite its significance, logging is often overlooked or not given the attention it deserves.
Why Logging Matters in Test Automation
Logging serves as a crucial tool for understanding the behavior of automated test scripts. It enables testers and developers to track the flow of the test execution, identify issues, and diagnose failures. Additionally, comprehensive and well-structured logs can provide valuable information for troubleshooting and analysis.
Choosing the Right Logging Framework
When it comes to logging in Java-based test automation frameworks, selecting the appropriate logging framework is paramount. The two most popular choices are Log4j and Logback. Both offer powerful features for capturing log output, defining log levels, and organizing log statements.
For instance, in Log4j, the configuration file log4j2.xml
or log4j.properties
allows customization of log levels for different packages and classes, while Logback's logback.xml
provides similar flexibility in configuration.
The choice between Log4j and Logback usually hinges on factors like ease of configuration, performance, and ecosystem support.
Implementing Logging in Test Automation
Let's delve into an example of implementing logging using Log4j in a test automation framework.
Assuming we have a test scenario where we want to log the steps of a user authentication process using Selenium WebDriver, we can integrate Log4j to capture and report the details.
First, ensure that the Log4j library is included in your project's dependencies. For a Maven project, you can add the following dependency to your pom.xml
:
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.14.1</version>
</dependency>
Next, create a log4j2.xml
configuration file in the resources
directory to define the appender and loggers. Here’s a basic example:
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="WARN">
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
</Console>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
</Configuration>
In the test class, initialize the logger and use it to record the steps of the authentication process:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class AuthenticationTest {
private static final Logger logger = LogManager.getLogger(AuthenticationTest.class);
public void authenticateUser(String username, String password) {
logger.info("Initiating user authentication process");
// Perform authentication steps
logger.debug("Entered username: " + username);
logger.debug("Entered password: " + password);
// Simulate authentication process
boolean isAuthenticated = true; // Replace with actual authentication logic
if (isAuthenticated) {
logger.info("User authentication successful");
} else {
logger.error("User authentication failed");
}
}
}
In this example, the Logger
instance is used to report the initiation of the authentication process, input values, and the outcome of the authentication attempt.
Best Practices for Logging in Test Automation
To optimize the effectiveness of logging in test automation, it's essential to follow best practices:
-
Use Descriptive Log Messages: Craft log messages that convey meaningful information, aiding in comprehension when reviewing the logs.
-
Utilize Log Levels: Leverage log levels such as
info
,debug
,warn
, anderror
appropriately to differentiate between different types of messages. -
Contextual Logging: Include contextual details in log messages to provide a holistic view of the test execution flow and outcome.
-
Centralized Log Handling: Design a centralized approach for handling and storing logs to ensure uniformity and ease of access across test runs.
The Last Word
In conclusion, effective logging is indispensable in test automation for enhancing visibility, diagnosing issues, and facilitating analysis. By selecting the right logging framework, implementing logging in test scripts, and adhering to best practices, you can harness the power of logging to bolster the robustness of your automated test suite.
Remember, robust logging is not just about recording events; it's about crafting a narrative of the test execution that enables seamless comprehension and actionable insights.
Start implementing effective logging in your test automation endeavors today, and witness the profound impact it can have on the reliability and maintainability of your test suite.
To deepen your understanding of logging frameworks in Java, check out Log4j documentation and Logback documentation.