Overcoming Challenges in Documenting Microservices
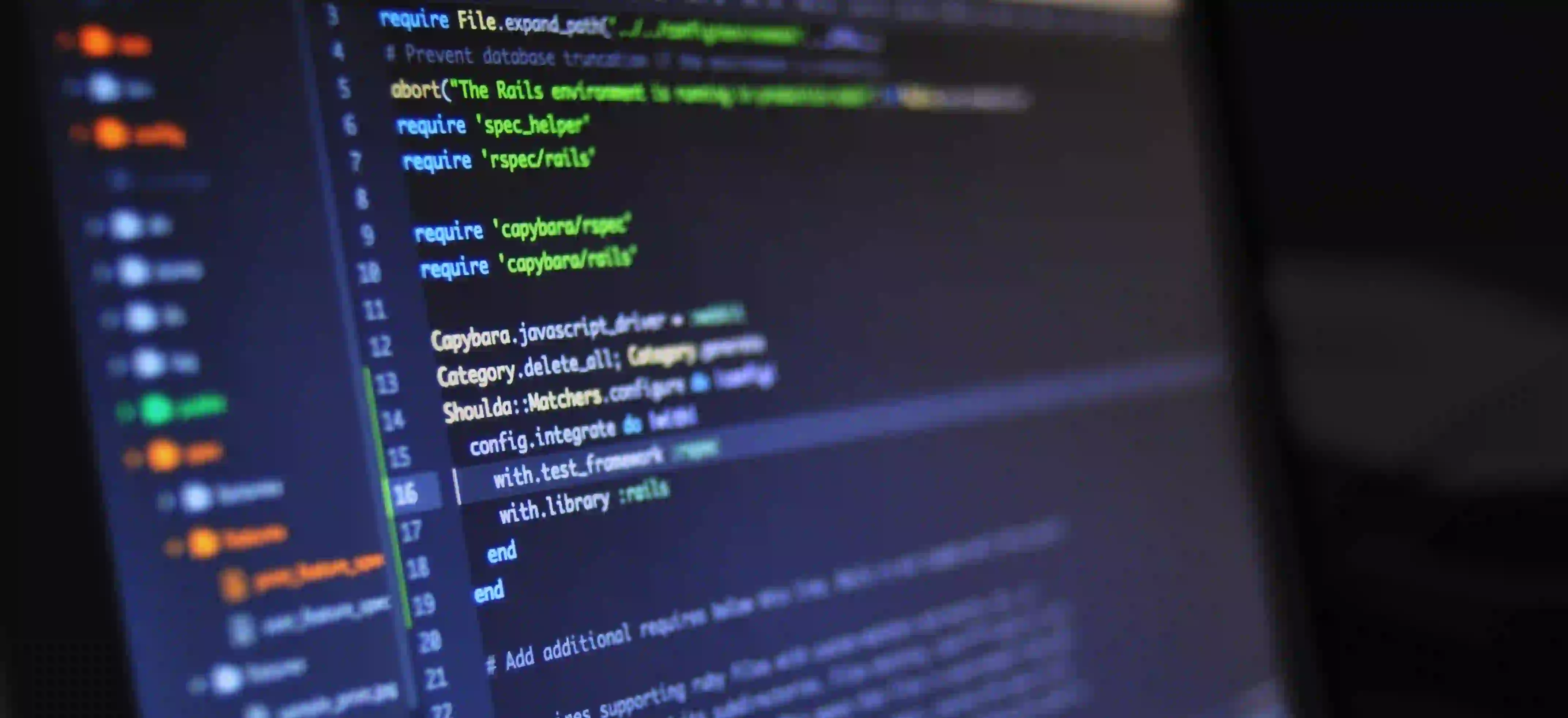
Best Practices for Documenting Microservices in Java
Microservices architecture has gained significant popularity due to its flexibility, scalability, and the ability to enable continuous delivery. However, as the number of microservices within an architecture grows, so does the complexity of documenting them. In this article, we'll discuss the importance of documenting microservices and explore best practices for effectively documenting microservices in a Java-based architecture.
Why Document Microservices?
Documenting microservices is crucial for maintaining a clear understanding of the architecture, especially as teams and projects evolve over time. Proper documentation facilitates seamless onboarding of new team members, aids in debugging and troubleshooting, and ensures efficient collaboration across teams.
In a microservices environment, documentation becomes even more critical due to the distributed nature of the system. Each microservice may have its own technology stack, data model, and communication protocols, making it challenging to comprehend the system as a whole without comprehensive documentation.
Challenges in Documenting Microservices
Before diving into best practices, it's important to understand the challenges associated with documenting microservices in Java.
1. Fragmented Documentation
With each microservice being developed, deployed, and documented independently, it's common to end up with fragmented documentation scattered across different repositories, wikis, and knowledge bases. This fragmentation makes it difficult to maintain consistency and ensures that the documentation is up to date.
2. Service Discovery
In a microservices architecture, services need to dynamically discover and communicate with each other. Properly documenting service discovery mechanisms, such as using Eureka for service registration and discovery, is essential for understanding how different microservices interact with each other.
3. Contract Documentation
Microservices communicate with each other through APIs. Documenting the contracts, including request and response payloads, endpoints, and supported operations, is crucial for seamless integration and avoiding misunderstandings between teams developing different services.
4. Runtime Dependencies
Understanding the runtime dependencies of a microservice, including external services it relies on, database connections, and messaging queues, is vital for troubleshooting and ensuring the reliability of the system.
Best Practices for Documenting Microservices in Java
1. OpenAPI Specification
The OpenAPI Specification (OAS), formerly known as Swagger, is a widely adopted standard for documenting RESTful APIs. Using tools like Springfox in Java, you can automatically generate API documentation from your code. This ensures that your API documentation stays in sync with your codebase and provides a consistent and machine-readable format for describing your API.
Using OAS brings several benefits:
- Consistency: All APIs are documented in a consistent manner, making it easier for developers to understand and consume them.
- Automatic Generation: API documentation is generated directly from code, reducing the manual effort required to maintain it.
- Interactivity: Swagger UI, which comes with Springfox, allows developers to interact with the API documentation, making it easier to explore and understand the endpoints and payloads.
Here's an example of annotating a Java controller method with Springfox annotations to generate OAS documentation:
import io.swagger.annotations.ApiOperation;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@RequestMapping("/products")
public class ProductController {
@ApiOperation(value = "Get a list of products")
@GetMapping
public List<Product> getProducts() {
// Implementation
}
}
The @ApiOperation
annotation provides metadata for the endpoint, describing its purpose and operation.
2. Service Registry Documentation
When using a service registry like Eureka for service discovery, documenting the registration process and how services interact with the registry is crucial. This documentation should include instructions for registering new services, updating service metadata, and consuming services from the registry.
3. Contract-First API Development
Adopting a contract-first approach to API development encourages documenting API contracts before writing code. Tools like Swagger Editor or Stoplight enable you to design and document your API contracts using the OAS. Once the contract is defined, Java developers can use code generation tools like OpenAPI Generator to generate server stubs and client libraries, ensuring that the API implementation aligns with the documented contract.
This approach promotes consistency between the documented contract and the actual implementation and encourages teams consuming the API to have a clear understanding of the expected payloads and behavior.
4. Dependency Graph Visualization
Visualizing the dependencies between microservices and their external dependencies can greatly aid in understanding the system. Tools like Spring Cloud Sleuth can be used to trace and visualize the flow of requests across microservices, providing insights into the runtime dependencies and potential bottlenecks in the system.
5. Centralized Documentation Repository
Establishing a centralized documentation repository, using tools like SwaggerHub, GitBook, or Confluence, consolidates all microservice documentation in one place. This promotes consistency, ease of access, and enables versioning and collaboration among teams.
My Closing Thoughts on the Matter
Effectively documenting microservices in a Java-based architecture is essential for ensuring clarity, consistency, and collaboration across development teams. By leveraging tools like OpenAPI Specification for API documentation, adopting contract-first API development, and visualizing service dependencies, the challenges associated with documenting microservices can be overcome, enabling teams to efficiently work with and evolve the architecture over time.
By incorporating these best practices, teams can streamline the onboarding process, reduce communication overhead, and ensure that the microservices ecosystem remains well-documented and comprehensible for all stakeholders. Remember, comprehensive and up-to-date documentation is not just a nice-to-have, but a fundamental aspect of successful microservices development and maintenance.