Creating Custom Client Events in ADF Faces
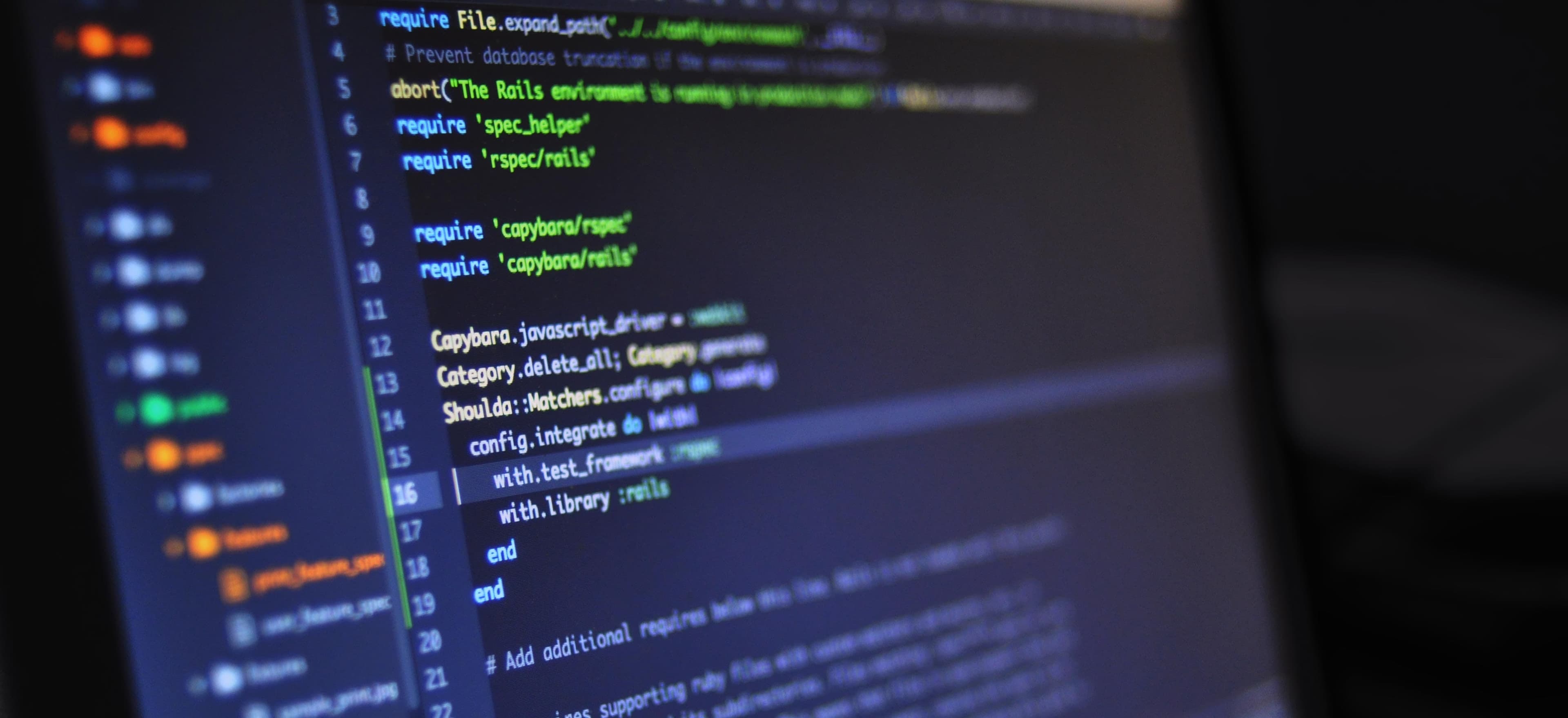
- Published on
Improving User Experience with Custom Client Events in ADF Faces
In the realm of web applications, user experience plays a pivotal role in the success and adoption of a system. As developers, we constantly strive to enhance user interaction and responsiveness. Oracle Application Development Framework (ADF) Faces provides a robust set of tools for building modern web applications. In this blog post, we will explore how to create custom client events in ADF Faces to improve user experience and application functionality.
Understanding Client Events in ADF Faces
Client events in ADF Faces encompass a wide range of interactions such as button clicks, input changes, form submissions, and more. While ADF Faces offers a rich set of built-in client events, there are scenarios where custom events are needed to achieve specific functionalities or integrations.
The Need for Custom Client Events
Custom client events provide developers with the flexibility to implement tailored interactions based on application-specific requirements. Whether it's triggering an action on a certain combination of user inputs or integrating with third-party libraries, creating custom events allows for a finer control over the application's behavior.
Implementing Custom Client Events in ADF Faces
To illustrate the implementation of custom client events, let's consider a scenario where we want to trigger a custom event when a user double-clicks on a specific component. We'll use JavaScript and ADF Faces to achieve this.
Firstly, let's create a custom ADF Faces component that represents the element on which the double-click event will be triggered. Here's an example of how the component can be defined in the ADF Faces page (JSF):
<af:outputText id="customComponent" value="Click me" ondblclick="handleDoubleClick()"/>
In the above code snippet, we've defined an outputText
component with its ID set to "customComponent". We also attach a JavaScript ondblclick
event handler to it, pointing to a function named handleDoubleClick()
.
Moving on, let's create the handleDoubleClick
JavaScript function that will be responsible for triggering our custom event. Here's an example of how this function can be defined:
function handleDoubleClick() {
var customEvent = new CustomEvent('adfCustomDoubleClick', { detail: { message: 'Double-click event occurred' } });
document.getElementById('customComponent').dispatchEvent(customEvent);
}
In the code above, we utilize the CustomEvent
constructor to create a custom event named 'adfCustomDoubleClick'. The event is configured with a detail
object containing relevant information about the event. We then use dispatchEvent
to trigger the custom event on the 'customComponent' element.
Now, let's handle the custom event in our ADF Faces application. We can use ADF Faces' JavaScript API to listen for the custom event and define the action to be taken when the event is fired:
AdfPage.PAGE.findComponent('customComponent').addEventListener('adfCustomDoubleClick', function(event) {
console.log('Custom double-click event received: ' + event.detail.message);
// Additional custom logic can be implemented here
});
In the code snippet above, we utilize addEventListener
to listen for the 'adfCustomDoubleClick' event on the 'customComponent'. When the event is fired, we log a message to the console, demonstrating that the custom event was received. Furthermore, we can implement additional custom logic within this listener function.
Advantages of Custom Client Events in ADF Faces
Enhanced Application Interactivity
By creating custom client events, developers can enhance the interactivity of ADF Faces applications, allowing for nuanced user interactions and dynamic behavior.
Integration with External Libraries
Custom events enable seamless integration with third-party JavaScript libraries and frameworks, empowering developers to leverage external capabilities while retaining the robustness of ADF Faces components.
Tailored Functionality
Custom events facilitate the implementation of tailored functionality based on specific user actions, providing a more personalized and responsive user experience.
In Conclusion, Here is What Matters
Custom client events in ADF Faces are a powerful tool for augmenting the interactivity and functionality of web applications. By understanding the need for custom events and following the implementation examples provided in this post, developers can harness the full potential of ADF Faces to create engaging and dynamic user experiences.
In conclusion, the ability to create custom client events in ADF Faces equips developers with the means to craft highly responsive and interactive web applications that resonate with modern user expectations.
By leveraging the concepts and techniques covered in this post, developers can take a significant step towards achieving seamless integration, personalized interaction, and an overall enhanced user experience within ADF Faces applications.
Remember, the key to success lies in tapping into the flexibility and extensibility that custom client events offer, ultimately empowering developers to build applications that truly stand out.
Check out more about Oracle ADF Faces and developing web applications.
Checkout our other articles