Common Issues with Mock-Based Unit Testing
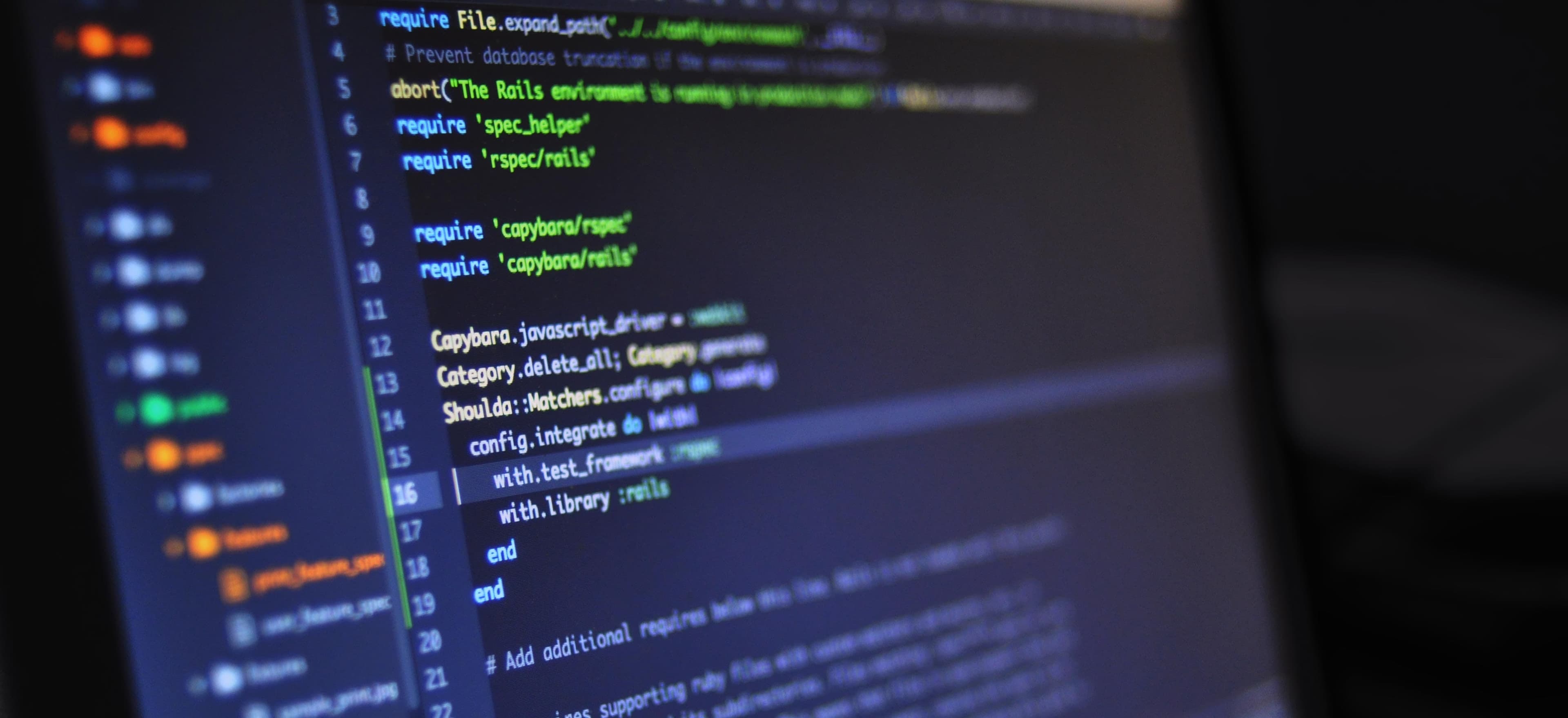
- Published on
Common Issues with Mock-Based Unit Testing
Mock-based unit testing is a popular technique in Java for testing individual components in isolation. While it has numerous benefits, such as enabling early bug detection and facilitating the testing of edge cases, it also comes with its own set of challenges. In this blog post, we'll explore some of the common issues associated with mock-based unit testing in Java and discuss potential solutions.
Issue 1: Tight Coupling
One of the primary challenges with mock-based unit testing is the potential for tight coupling between the test code and the implementation details of the component being tested. When writing mock-based tests, it's easy to inadvertently couple the test to the internal behavior of the component, making the test brittle and prone to breaking with any minor changes to the implementation.
Solution: Use Mocking Frameworks
To mitigate this issue, leverage mocking frameworks, such as Mockito, EasyMock, or PowerMock, to create mocks for dependencies. By using these frameworks, you can define the behavior of the mocks independently of the implementation details of the component, reducing the risk of tight coupling.
Here's an example using Mockito to create a mock and define its behavior:
// Creating a mock
SomeInterface mock = mock(SomeInterface.class);
// Defining mock behavior
when(mock.someMethod()).thenReturn(someValue);
By defining the behavior of the mock explicitly, you can decouple the test from the internal details of the component.
Issue 2: Over-Specification
Another common issue with mock-based unit testing is over-specifying the behavior of the mocks. When tests become too focused on verifying the exact interactions between the component and its dependencies, they can become overly rigid and fail to accommodate legitimate changes in the implementation.
Solution: Focus on Outcomes Rather Than Interactions
Instead of specifying the exact sequence of method calls on the mocks, focus on defining the expected outcomes of the interactions. This approach allows the implementation to evolve without breaking the tests as long as the expected outcomes are still achieved.
Here's an example illustrating how to focus on outcomes using Mockito:
// Verifying expected outcome
verify(mock, times(1)).someMethod();
By verifying the outcome of the interaction, you can maintain flexibility in the implementation while still ensuring the intended behavior.
Issue 3: Maintenance Overhead
Maintaining a large number of mock-based tests can become cumbersome, especially as the codebase evolves. Tests that are overly reliant on mocks may require frequent updates to reflect changes in the implementation or the dependencies, leading to increased maintenance overhead.
Solution: Use a Combination of Testing Approaches
While mock-based testing is valuable, it's essential to complement it with other testing approaches, such as integration testing and contract testing. By employing a diverse set of testing techniques, you can distribute the testing responsibilities across different types of tests, reducing the maintenance burden on mock-based tests.
Additionally, consider using tools like SonarQube to analyze code quality and identify areas where the testing approach may be overly reliant on mocks.
Issue 4: Fragile Tests
Overly-specific mock behavior definitions can lead to fragile tests that break easily when the implementation changes. This fragility can erode confidence in the tests and undermine their effectiveness in providing reliable feedback during development.
Solution: Emphasize High-Level Behavior
When defining mock behaviors, prioritize capturing the high-level behavior of the component rather than getting bogged down in low-level details. By focusing on the overarching behavior, you can create tests that are more resilient to changes in the implementation.
To Wrap Things Up
Mock-based unit testing is a powerful technique for isolating components and verifying their behavior in a controlled environment. However, it's essential to be mindful of the potential pitfalls associated with mock-based testing and employ best practices to mitigate these challenges effectively. By leveraging mocking frameworks, focusing on outcomes, diversifying the testing approach, and emphasizing high-level behavior, you can harness the benefits of mock-based testing while minimizing its drawbacks.
Incorporating these strategies into your testing practices will help you create robust, maintainable tests that provide valuable insights into the behavior of your Java components.
For further reading on mock-based unit testing, consider exploring the official Mockito documentation and Effective Mockito for in-depth insights and best practices.