Optimizing Code Efficiency with Functional Programming
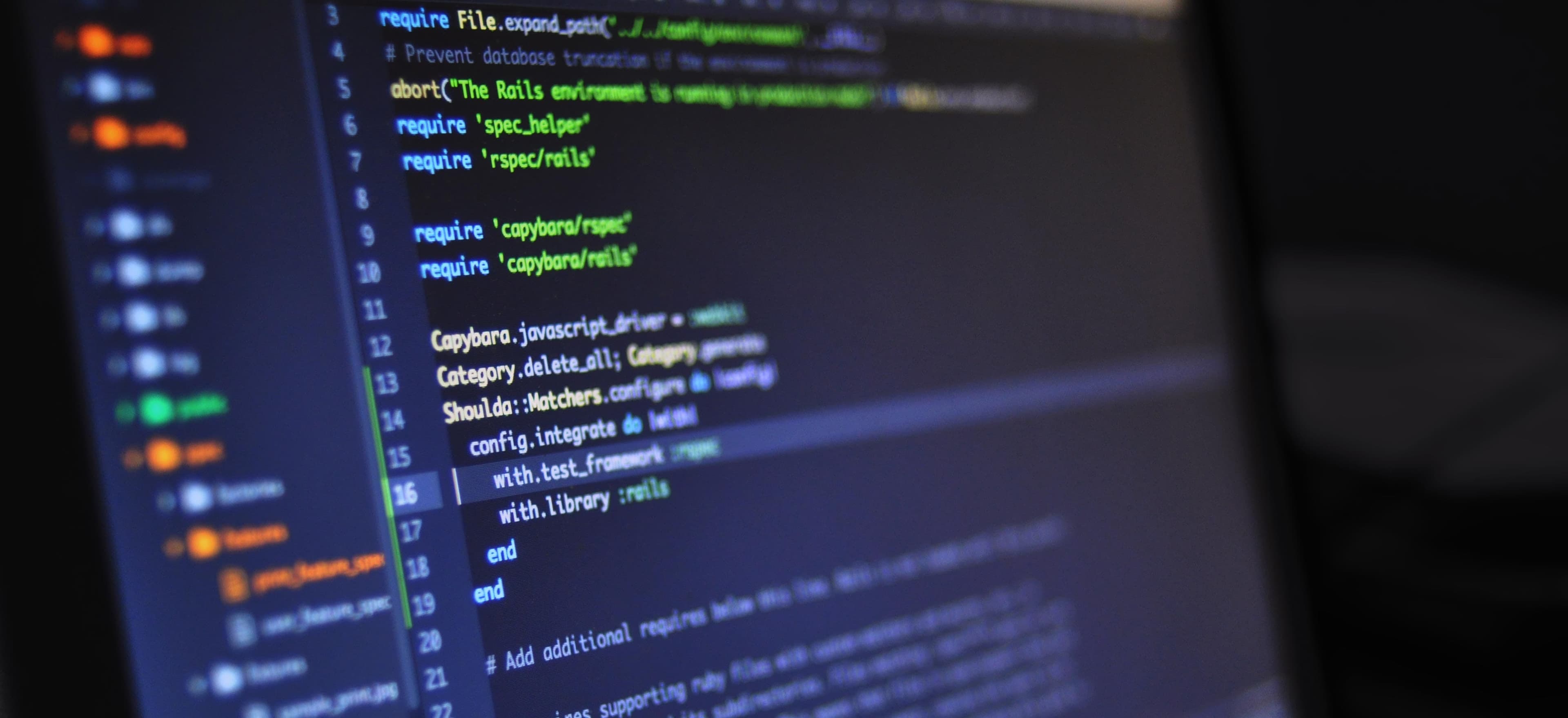
- Published on
Optimizing Code Efficiency with Functional Programming
Writing efficient and clean code is essential for the performance and maintainability of any software application. Java, being one of the most popular programming languages, offers various techniques to achieve code efficiency. In this article, we will explore how functional programming can be used to write more concise, readable, and efficient code in Java.
What is Functional Programming?
Functional programming is a paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. In functional programming, functions are first-class citizens, meaning they can be passed as arguments to other functions, returned as values from other functions, and assigned to variables.
In Java, functional programming capabilities were greatly enhanced with the introduction of lambda expressions and the Stream API in Java 8. These features allow developers to write code in a more declarative style, focusing on what the code should accomplish rather than how it should be done.
Benefits of Functional Programming in Java
Concise and Readable Code
Functional programming encourages writing code in a more declarative and expressive manner, which often leads to more concise and readable code. By using lambda expressions and the Stream API, Java developers can eliminate boilerplate code and focus on the core logic of their algorithms.
Immutability
Immutability is a fundamental concept in functional programming. By avoiding mutable state, the code becomes less error-prone and easier to reason about. In Java, the final
keyword can be used to create immutable variables, and libraries like Immutables can further facilitate immutable object creation.
Parallelism
Functional programming provides a natural way to support parallelism. With the Stream API, developers can easily parallelize operations, taking advantage of multi-core processors and improving overall performance.
Example: Refactoring Imperative Code to Functional Code
Let's consider a simple example where we have a list of numbers and we want to filter out the even numbers and then double each remaining number.
Imperative Style
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> doubledEvens = new ArrayList<>();
for (int number : numbers) {
if (number % 2 == 0) {
doubledEvens.add(number * 2);
}
}
Functional Style
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
List<Integer> doubledEvens = numbers.stream()
.filter(n -> n % 2 == 0)
.map(n -> n * 2)
.collect(Collectors.toList());
In the functional style, the code is more expressive and eliminates the need for mutable state and explicit looping. The filter
and map
operations clearly define the logic, making the code easier to understand and maintain.
Leveraging Functional Interfaces
Functional interfaces, which have exactly one abstract method, are instrumental in functional programming in Java. They enable the use of lambda expressions and method references, making it easier to work with functional constructs.
Example: Consumer Functional Interface
The Consumer
functional interface represents an operation that accepts a single input argument and returns no result. It is often used when iterating through a collection and performing an operation on each element.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println("Hello, " + name));
By leveraging the Consumer
interface and lambda expressions, the code is succinct and expressive, achieving the goal of printing each name with minimal ceremony.
Lazy Evaluation with Streams
Streams in Java provide a powerful way to work with sequences of elements. One of the key advantages of streams is their support for lazy evaluation, which means that intermediate operations are only evaluated when terminal operations are invoked. This allows for more efficient processing of data.
Example: Lazy Evaluation
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David", "Eve");
Optional<String> firstMatch = names.stream()
.filter(name -> name.startsWith("C"))
.findFirst();
In this example, the filter
operation uses lazy evaluation, only processing elements until the first match is found. This can be especially beneficial when working with large datasets or expensive computation.
Use Cases for Functional Programming in Java
Data Transformation and Processing
Functional programming is well-suited for data transformation and processing tasks, such as filtering, mapping, and reducing collections of data. The Stream API in Java provides a concise and expressive way to perform these operations.
Asynchronous Programming
The CompletableFuture class introduced in Java 8 enables asynchronous programming with a functional approach. By leveraging methods like thenApply
and thenCompose
, developers can chain asynchronous computations and handle results without dealing with low-level threading.
Event Handling and Callbacks
Functional interfaces and lambda expressions are instrumental in implementing event handling and callbacks in Java. They provide a more elegant and compact way to define actions that should be executed in response to events.
Bringing It All Together
Functional programming offers valuable tools and techniques to write more efficient and maintainable code in Java. By leveraging features like lambda expressions, the Stream API, and functional interfaces, developers can express their intent more clearly, reduce boilerplate code, and take advantage of parallelism and lazy evaluation.
As software applications continue to grow in complexity and scale, functional programming principles can play a pivotal role in ensuring code efficiency and resilience. Embracing functional programming in Java can lead to more robust, scalable, and maintainable systems.
In conclusion, functional programming in Java is not just a trend, but a valuable approach for crafting high-performance, clean, and elegant code.
By incorporating functional programming concepts and best practices into your Java development workflow, you can elevate the quality and efficiency of your codebase, empowering you to tackle complex problems with clarity and precision.