Effective Software Debugging Techniques
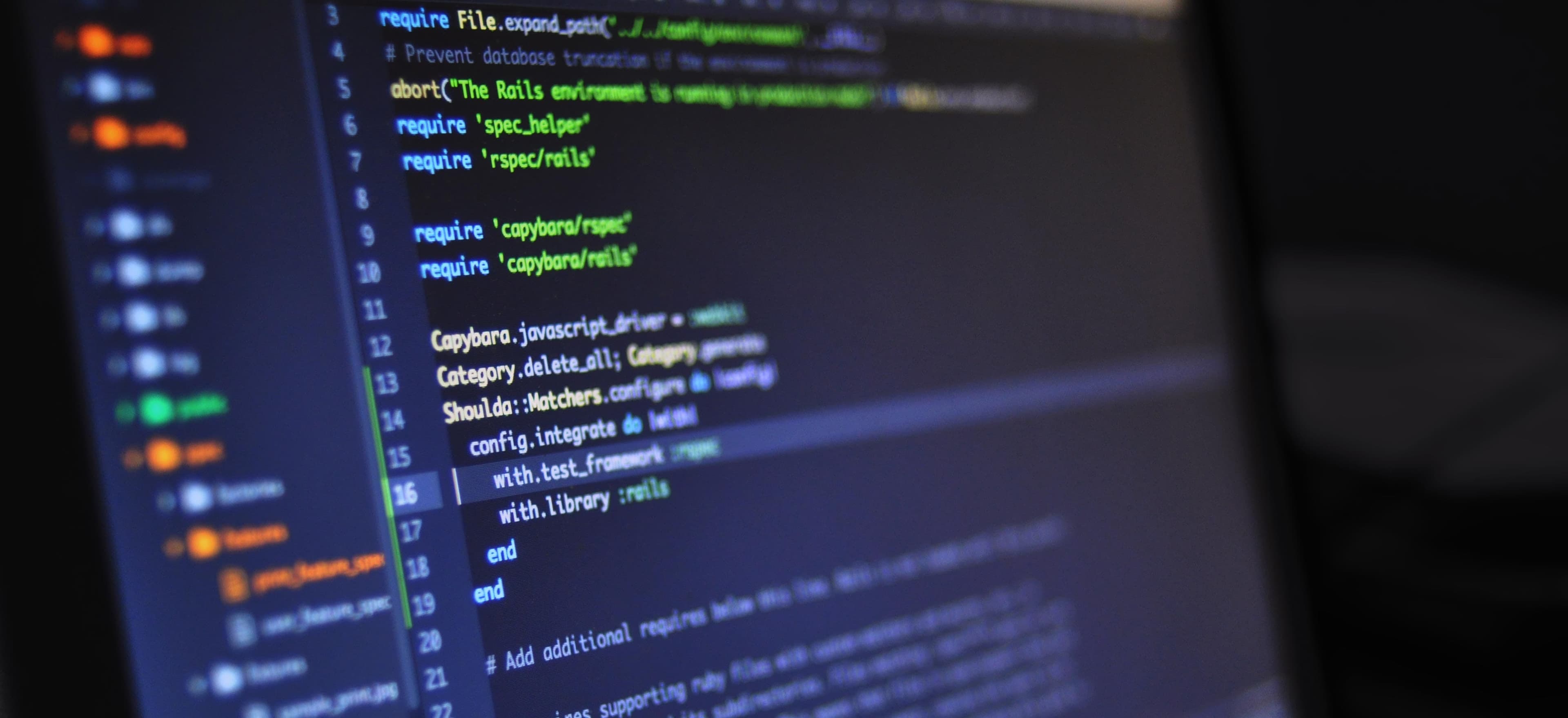
- Published on
Effective Software Debugging Techniques
Debugging is an essential aspect of software development that entails identifying and resolving issues in a program's code. Quality debugging is crucial to ensuring the smooth operation of an application, and proficient debugging skills are a hallmark of a skilled developer. This post delves into various effective and practical techniques for debugging Java applications.
1. Understand the Problem
Before delving into debugging, it is crucial to comprehend the issue at hand. This involves reproducing the problem and understanding its scope. Utilize logging frameworks such as Log4j or SLF4J to capture relevant information, such as stack traces, variable states, and intermediate outputs.
2. Utilize Integrated Development Environments (IDEs)
IDEs provide robust debugging tools that facilitate efficient issue resolution. Popular Java IDEs like IntelliJ IDEA and Eclipse offer features such as breakpoints, step-through execution, variable inspection, and expression evaluation, which are indispensable for pinpointing flaws in the code.
3. Use System.out.println() for Quick Checks
While logging frameworks are ideal for comprehensive debugging, employing System.out.println()
statements enables quick checks during the development phase. These statements can help ascertain the state of variables or the flow of execution at specific points in the code.
int result = someComplexCalculation();
System.out.println("Result: " + result);
4. Leverage Exception Handling
Appropriate exception handling can aid in identifying and handling runtime errors. Utilize try-catch blocks to catch exceptions and log pertinent details. Additionally, employing custom exception classes can enhance error traceability.
try {
// Code that may throw an exception
} catch (CustomException ex) {
logger.error("CustomException occurred: " + ex.getMessage());
}
5. Employ Unit Testing
Unit tests are invaluable for uncovering defects early in the development cycle. Utilize frameworks like JUnit to create comprehensive test suites that evaluate the functionality of individual units of code. By automating tests, developers can identify issues swiftly and accurately.
6. Perform Binary Search Debugging
When dealing with extensive codebases, isolating the problematic segment is challenging. Employ a binary search approach by systematically narrowing down the potential problematic areas. Comment out sections, utilize breakpoints, or employ logging to locate the specific code fragment causing the issue.
7. Utilize Remote Debugging
Debugging issues occurring in a different environment, such as a production server, can be daunting. With remote debugging, developers can connect an IDE to a running Java process, enabling real-time inspection and modification of code. This technique is invaluable for resolving elusive bugs.
8. Employ Memory Analysis Tools
Memory leaks and suboptimal memory usage can lead to performance degradation and application instability. Profilers like VisualVM and YourKit can be employed to analyze memory consumption, identify memory leaks, and optimize memory usage.
9. Version Control for Debugging
Version control systems like Git offer invaluable support for debugging. By using branches, developers can isolate specific changes and test hypotheses without affecting the main codebase. Moreover, version control facilitates the identification of the origin of issues by tracking code modifications.
10. Collaborate and Seek Fresh Perspectives
Seeking input from peers can provide novel insights into perplexing issues. Engaging in code reviews and seeking assistance from fellow developers fosters a collaborative environment where diverse perspectives can lead to innovative solutions.
The Closing Argument
Effective debugging is a combination of technical prowess, systematic approaches, and the utilization of appropriate tools. By comprehensively understanding the problem, leveraging advanced debugging features, and collaborating with peers, developers can adeptly resolve issues in Java applications. As software systems grow in complexity, honing debugging skills proves to be a continual and indispensable endeavor for developers.
In conclusion, proficient debugging skills are a hallmark of a capable Java developer and are indispensable for maintaining the operational stability of applications. By leveraging a combination of comprehensive understanding, robust tools, and systematic approaches, developers can adeptly resolve issues in their Java applications.