Common Challenges Faced in Java Programming Bootcamps
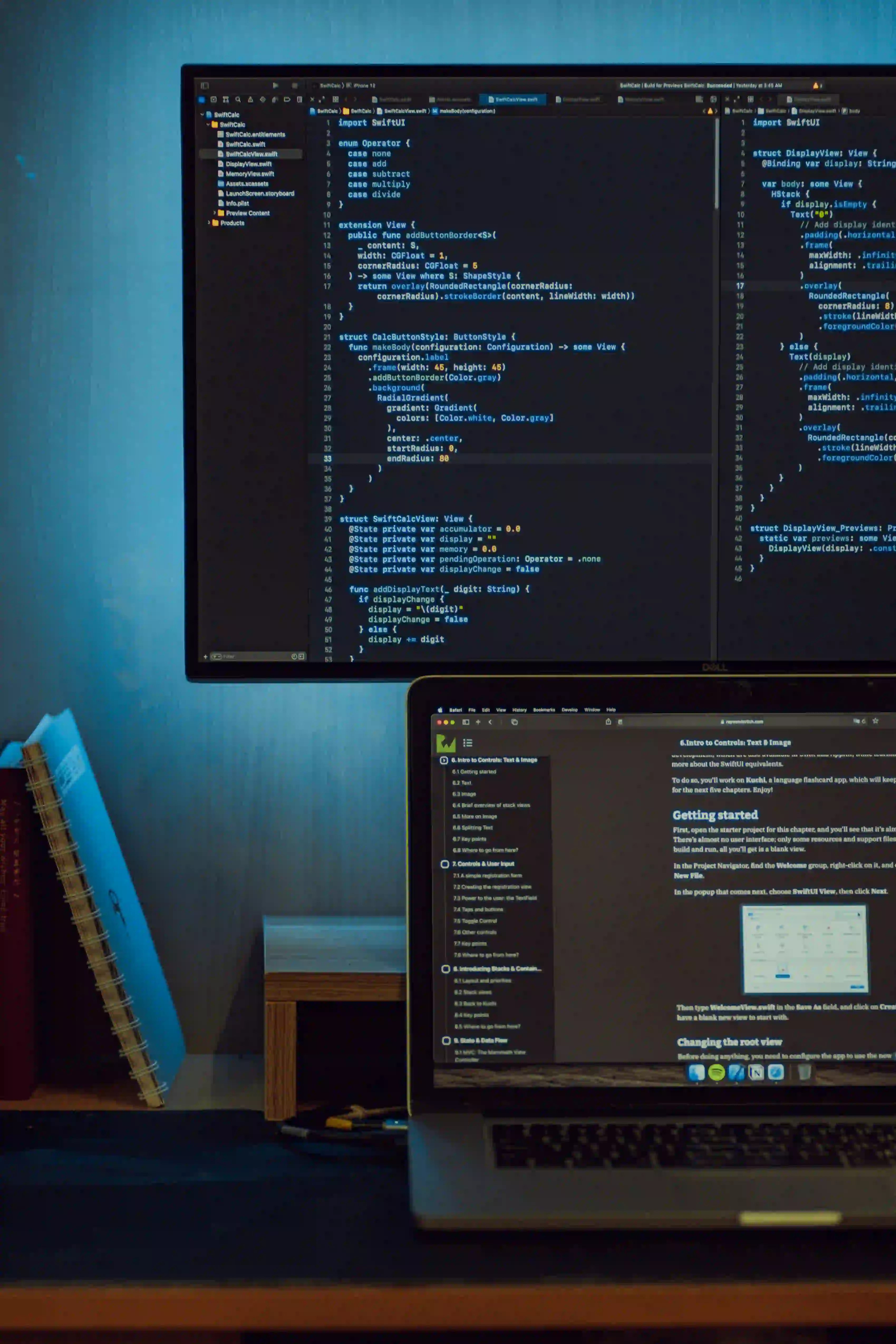
Common Challenges Faced in Java Programming Bootcamps
Java programming bootcamps provide an intensive learning experience for individuals looking to kickstart their careers in software development. While these bootcamps offer a structured environment for learning Java, participants often encounter various challenges that hinder their progress. In this blog post, we will explore some common challenges faced in Java programming bootcamps and discuss strategies to overcome them.
1. Understanding Object-Oriented Concepts
One of the fundamental concepts in Java programming is object-oriented programming (OOP). Participants with no prior experience in OOP may struggle to grasp the concepts of classes, objects, inheritance, polymorphism, and encapsulation. Without a solid understanding of OOP, writing efficient and scalable Java code becomes a daunting task.
How to Overcome:
- Interactive Learning: Utilize interactive exercises and projects to reinforce OOP concepts.
- Visualizing Concepts: Use visual aids such as UML diagrams to illustrate class relationships and inheritance hierarchies.
- Encourage Practice: Encourage participants to build small projects that demonstrate OOP principles in action.
2. Handling Exceptions and Error Handling
Java's robust error-handling mechanism, based on exceptions and try-catch blocks, can be challenging for beginners to comprehend. Handling different types of exceptions, knowing when to catch or throw exceptions, and implementing clean error handling logic requires a deep understanding of Java's exception-handling mechanism.
How to Overcome:
- Real-World Examples: Use real-world examples to explain the need for exceptions and how they improve code reliability.
- Practice Sessions: Provide structured exercises that involve handling various types of exceptions.
- Code Reviews: Review and discuss error-handling approaches in code written by participants to identify best practices.
// Example of try-catch block for handling exceptions
try {
// Risky code that may throw an exception
// ...
} catch (SpecificException ex) {
// Handle the specific exception
// ...
} catch (Exception ex) {
// Handle any other exceptions
// ...
} finally {
// Code that always executes, regardless of exceptions
// ...
}
In the above code snippet, the try-catch block is used to handle exceptions that may occur during the execution of the risky code.
3. Mastering Java Collections
Manipulating and working with data collections is a vital part of Java programming. Understanding the various types of collections (lists, sets, maps), knowing when to use each type, and applying the right collection methods can be overwhelming for bootcamp participants.
How to Overcome:
- Hands-On Exercises: Provide hands-on exercises to manipulate different types of collections.
- Performance Considerations: Discuss the performance implications of using specific collection types and methods.
- Utilize Libraries: Introduce popular libraries like Guava or Apache Commons Collections to showcase advanced collection operations.
// Example of using a List collection
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
for (String name : names) {
System.out.println(name);
}
In the code snippet above, an ArrayList is used to store and iterate through a list of names.
4. Grasping Multi-Threading and Concurrency
The concept of multi-threading and concurrent programming is crucial for building responsive and efficient Java applications. However, it is also one of the most complex areas for learners due to the intricacies of thread management, synchronization, and potential race conditions.
How to Overcome:
- Visual Demonstrations: Use visual demonstrations to explain the concept of threads running concurrently.
- Hands-On Projects: Assign projects that involve implementing multi-threaded applications to reinforce learning.
- Focus on Pitfalls: Highlight common pitfalls such as deadlock and thread interference to instill best practices in concurrent programming.
Wrapping Up
Java programming bootcamps offer a fast-paced and immersive learning experience, but they come with their own set of challenges. By addressing these common obstacles and implementing effective learning strategies, participants can enhance their understanding of Java and emerge as proficient programmers.
In conclusion, understanding and overcoming these challenges not only prepares participants for the demands of Java development but also equips them with problem-solving skills crucial for success in the industry.
To delve further into Java programming, consider exploring in-depth resources such as Oracle's official Java documentation. Additionally, Codecademy's Java course provides interactive and comprehensive tutorials for mastering Java programming concepts.
Keep learning, keep coding, and conquer the challenges of Java programming bootcamps!