The Pitfalls of Over-Engineering Test Suites
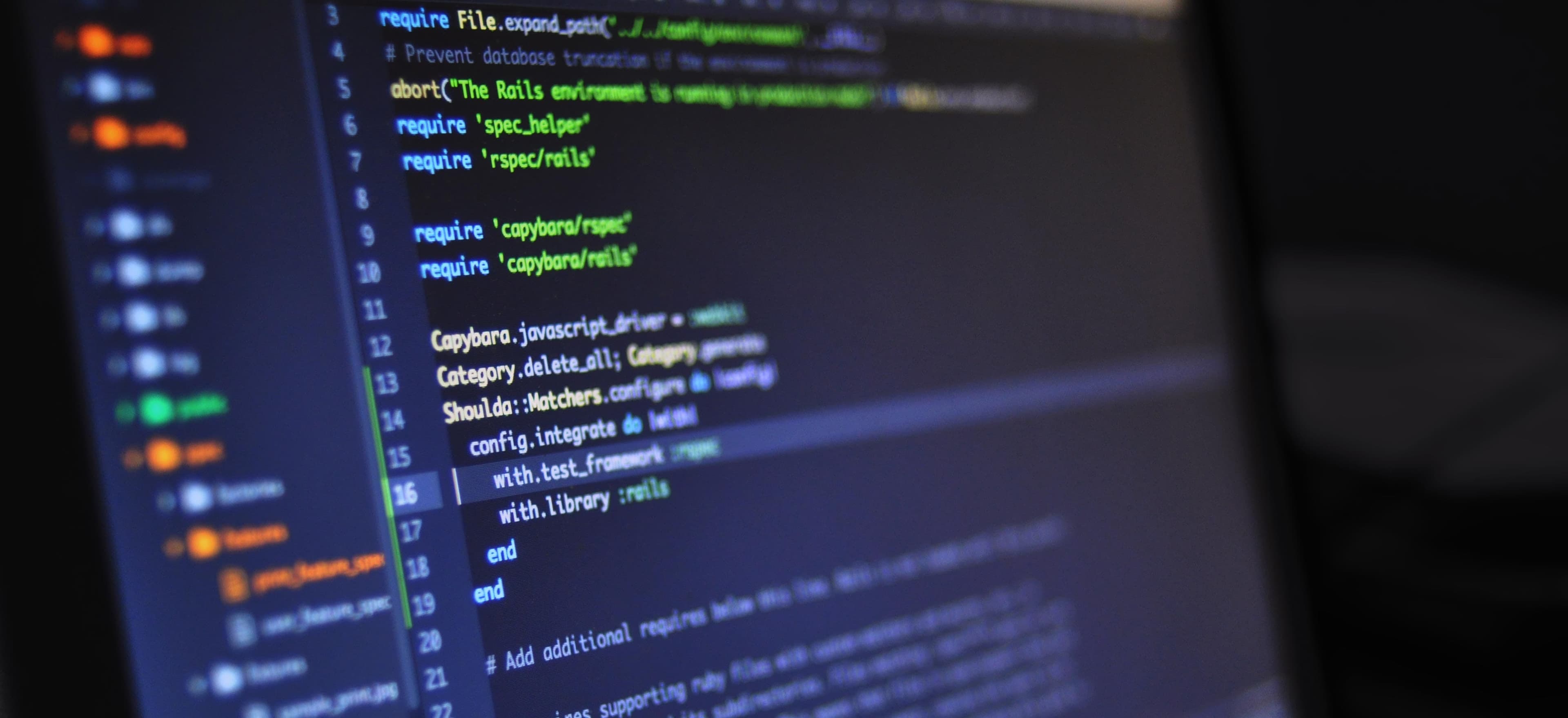
- Published on
The Pitfalls of Over-Engineering Test Suites
In the world of software development, testing is paramount. It ensures that the codebase performs as expected, maintains quality, and prevents regressions. However, there comes a point where test suites can become over-engineered, leading to pitfalls that can hinder rather than help the development process.
What is Over-Engineering in Test Suites?
Over-engineering occurs when developers create overly complex solutions to simple problems. In the context of test suites, this can manifest in a variety of ways, such as excessive use of mocking, intricate setup and teardown procedures, and an overreliance on advanced testing libraries.
The Downside of Over-Engineering
While it's essential to have a comprehensive test suite, over-engineering can lead to several negative outcomes:
-
Increased Maintenance Burden: Overly complex tests are harder to maintain. When the production code changes, the tests must be updated accordingly, which becomes more challenging with intricate test setups.
-
Slower Test Execution: Complex test setups and excessive mocking can lead to slower test execution times. This can discourage developers from running the tests frequently, diminishing their effectiveness.
-
Reduced Readability: Test suites that are over-engineered are often harder to read and understand. This can lead to confusion among developers and make it difficult to identify the purpose of specific tests.
-
Brittleness: Overly complex tests are more prone to breakage, especially when the system undergoes changes. This can lead to false positives, where tests fail not because of bugs in the code but due to changes in the test setup.
How to Avoid Over-Engineering Test Suites
To prevent the pitfalls of over-engineering, developers can follow a set of best practices when creating and maintaining test suites:
1. Keep It Simple
Maintain a simple and straightforward approach to testing. Use complex solutions only when the problem genuinely requires them. Strive for clarity and readability in test code.
2. Embrace the TDD Approach
Adopt a Test-Driven Development (TDD) approach, where tests are written before the production code. This ensures that tests focus on the specific requirements and behaviors of the code, rather than unnecessary abstractions.
3. Limit the Use of Mocking
While mocking has its place in testing, excessive use can lead to overly complex and brittle tests. Reserve mocking for external dependencies and focus on writing integration and end-to-end tests when possible.
4. Refactor Regularly
Just as production code needs refactoring, so do test suites. Regularly revisit and refactor test code to ensure it remains clean, concise, and aligned with the changes in the production code.
5. Leverage Built-In Testing Frameworks
Modern programming languages, including Java, provide robust and built-in testing frameworks such as JUnit. Leverage these frameworks effectively, as they often offer a good balance between simplicity and functionality.
Let's illustrate some of these best practices using Java as an example.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class SimpleTest {
@Test
public void testAddition() {
int result = Calculator.add(3, 2);
assertEquals(5, result);
}
}
In this example, we're using JUnit 5 to write a simple test for an add
method in a Calculator
class. The test is concise, readable, and focuses on the specific behavior of the method.
6. Strive for Balance
It's essential to strike a balance between ensuring comprehensive test coverage and avoiding overcomplication. Focus on testing critical and complex logic while keeping the overall test suite simple and maintainable.
Bringing It All Together
While a robust test suite is crucial for high-quality software, over-engineering test suites can lead to more harm than good. By embracing simplicity, TDD, judicious use of mocking, and regular refactoring, developers can avoid the pitfalls of over-engineering and maintain an effective and maintainable test suite.
By following these best practices, developers can strike a balance between thorough testing and pragmatic simplicity, leading to more reliable and maintainable codebases.
In summary, keeping your test suites simple and focused can yield significant long-term benefits for the development process, making it more efficient, maintainable, and less error-prone.
To delve deeper into effective testing practices and frameworks in Java, consider exploring JUnit's official documentation. Furthermore, for insights into the Test-Driven Development approach, Martin Fowler's comprehensive guide provides valuable insights.
Checkout our other articles