Effective Testing Strategies for EJB 3.1 in Java EE 6
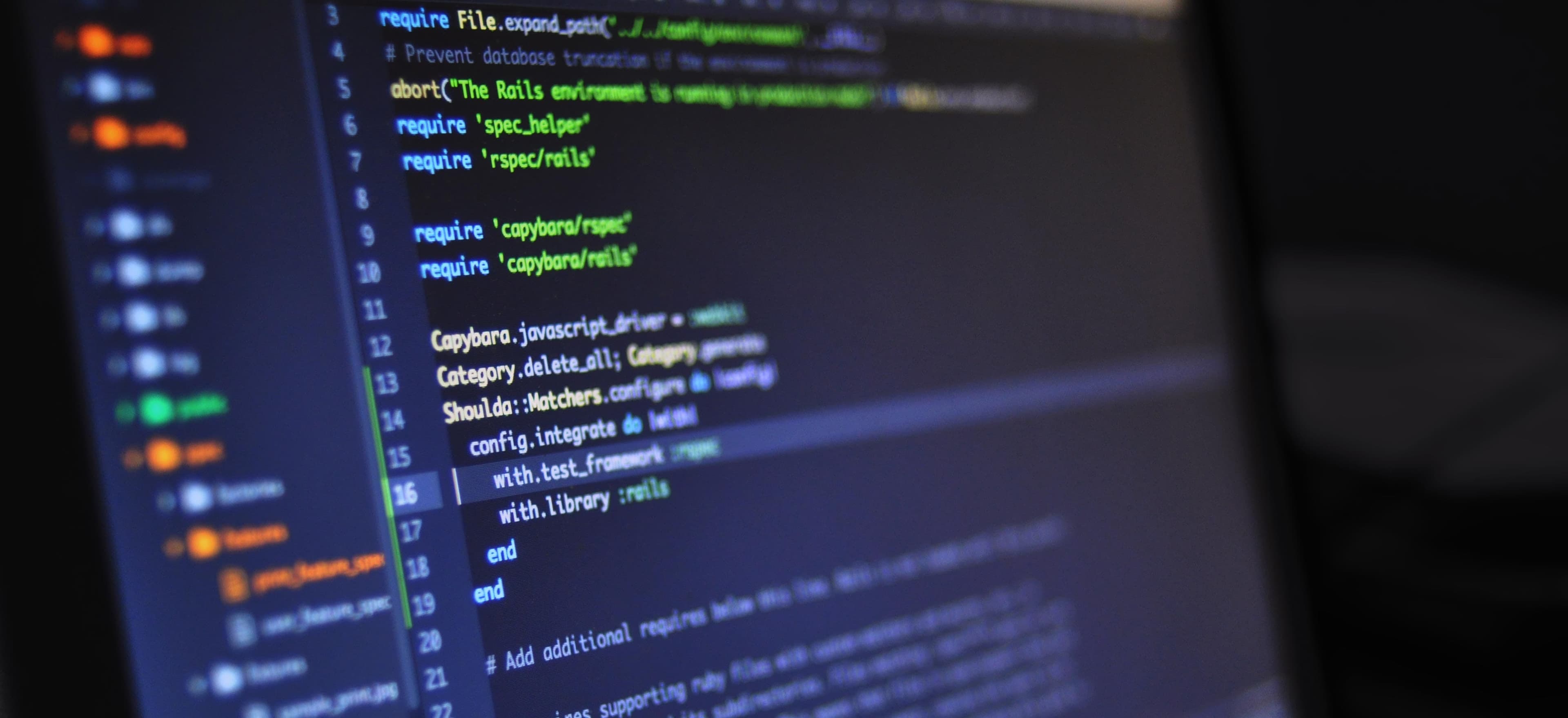
- Published on
Effective Testing Strategies for EJB 3.1 in Java EE 6
Testing Enterprise JavaBeans (EJB) 3.1 in Java EE 6 is crucial to ensure the reliability and stability of your enterprise applications. With the right testing strategies, you can identify and fix issues early in the development lifecycle, leading to higher quality software. In this article, we'll explore some effective testing strategies for EJB 3.1 in Java EE 6.
Unit Testing EJBs
Unit testing is the foundation of any robust testing strategy. When it comes to EJBs, it's important to focus on testing the business logic encapsulated within the beans. Using a framework like JUnit, you can write unit tests to validate the behavior of your EJB methods.
Example of Unit Testing an EJB with JUnit
import javax.ejb.EJB;
import static org.junit.Assert.assertEquals;
import org.junit.Test;
import com.example.MyEJB;
public class MyEJBTest {
@EJB
private MyEJB myEJB;
@Test
public void testSomeMethod() {
String expected = "Expected Result";
String actual = myEJB.someMethod();
assertEquals(expected, actual);
}
}
In this example, we're using JUnit to test the someMethod
of the MyEJB
EJB. We're asserting that the result of the method matches the expected result.
Integration Testing with Embedded EJB Container
Integration testing is essential to validate the interaction between EJBs and other components within the Java EE application. Using an embedded EJB container, such as Arquillian, you can perform comprehensive integration testing of your EJBs.
Example of Integration Testing with Arquillian
@RunWith(Arquillian.class)
public class MyEJBIntegrationTest {
@EJB
private MyEJB myEJB;
@Deployment
public static JavaArchive createDeployment() {
return ShrinkWrap.create(JavaArchive.class)
.addClass(MyEJB.class)
.addAsManifestResource(EmptyAsset.INSTANCE, "beans.xml");
}
@Test
public void testSomeMethod() {
String expected = "Expected Result";
String actual = myEJB.someMethod();
assertEquals(expected, actual);
}
}
In this example, we're using Arquillian to perform integration testing of the MyEJB
EJB. We're deploying the EJB to an embedded container and then testing its behavior.
Using Mocking Frameworks for Collaborator Testing
EJBs often depend on external collaborators, such as DAOs or other EJBs. To test the EJB's behavior in isolation, you can use mocking frameworks like Mockito or EasyMock to mock the collaborators.
Example of Collaborator Testing with Mockito
@RunWith(MockitoJUnitRunner.class)
public class MyEJBTest {
@InjectMocks
private MyEJB myEJB;
@Mock
private MyCollaboratorDAO collaboratorDAO;
@Test
public void testEJBBehavior() {
when(collaboratorDAO.getData()).thenReturn("Mocked Data");
String result = myEJB.doSomethingWithCollaborator();
assertEquals("Expected Result", result);
}
}
In this example, we're using Mockito to mock the MyCollaboratorDAO
collaborator and test the behavior of the MyEJB
EJB in isolation.
End-to-End Testing with Arquillian and Selenium
End-to-end testing is crucial to validate the entire application stack, including the EJBs and the web interface. By combining Arquillian for EJB testing and Selenium for web UI testing, you can perform comprehensive end-to-end testing of your Java EE application.
Example of End-to-End Testing with Arquillian and Selenium
@RunWith(Arquillian.class)
public class EndToEndTest {
@EJB
private MyEJB myEJB;
@Deployment(testable = false)
public static Archive<?> createDeployment() {
return ShrinkWrap.create(WebArchive.class)
.addClass(MyEJB.class)
.addAsWebInfResource(EmptyAsset.INSTANCE, "beans.xml")
.addAsWebResource(new File("src/main/webapp/index.html"));
}
@Test
public void testEndToEndScenario() {
// Use Selenium to interact with the web interface
// Call EJB methods and validate the results
}
}
In this example, we're using Arquillian to deploy the EJB and Selenium to interact with the web interface for end-to-end testing of the Java EE application.
Closing Remarks
Testing EJB 3.1 in Java EE 6 requires a combination of unit testing, integration testing, collaborator testing, and end-to-end testing. By leveraging tools and frameworks like JUnit, Arquillian, Mockito, and Selenium, you can ensure the reliability and robustness of your enterprise applications.
By incorporating these effective testing strategies into your development process, you can detect and address issues early, leading to higher quality, more stable, and reliable EJB-based applications.
Remember, effective testing is not just about finding bugs; it's about building confidence in the correctness and reliability of your software.
To learn more about testing EJBs in Java EE, check out Oracle's official documentation on EJB 3.1 and Arquillian's guide to testing Java EE applications.
Happy testing!