Choosing Between Server Components vs Server-Side Rendering
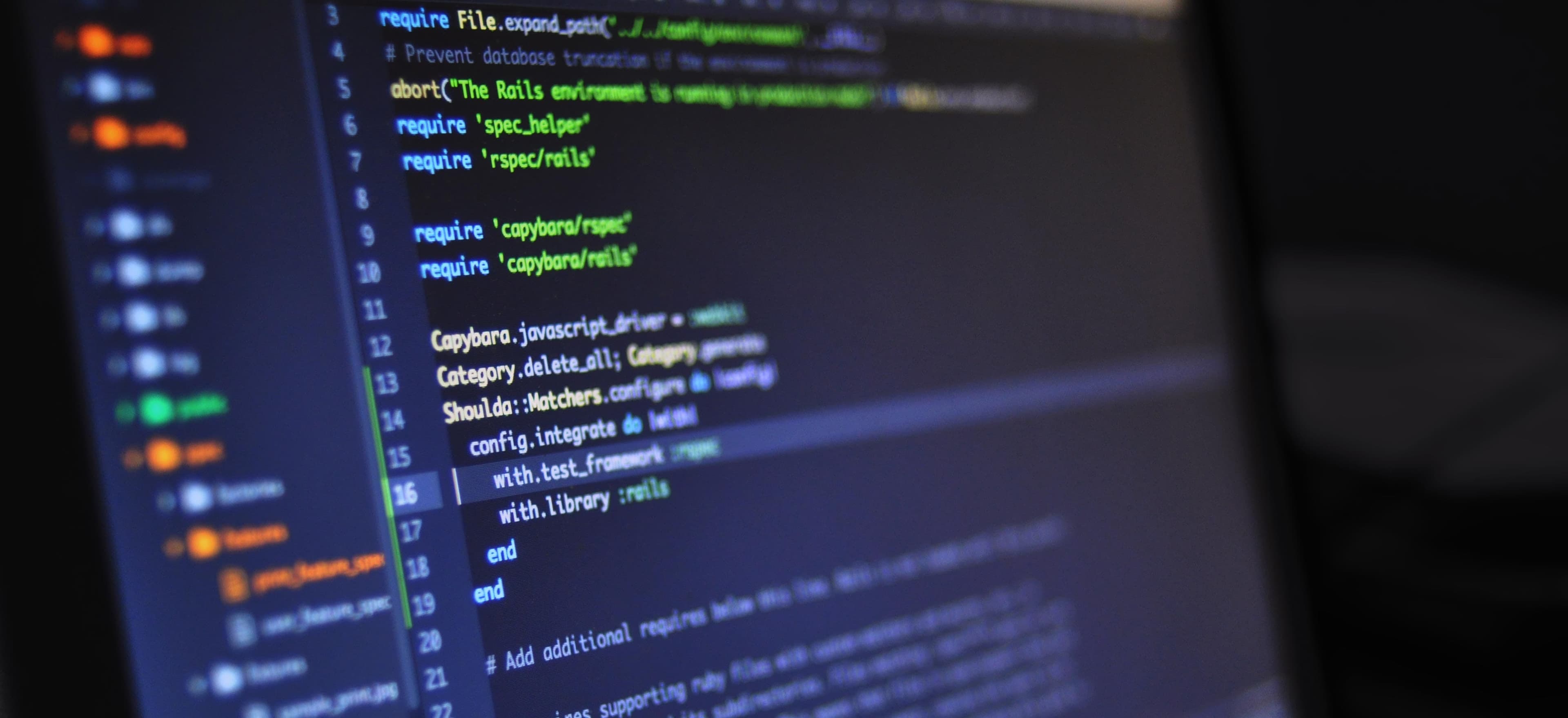
- Published on
Understanding the Difference: Server Components vs Server-Side Rendering
When it comes to developing web applications in Java, making the right architectural choices is crucial for the performance, scalability, and maintainability of the application. One of the key decisions developers often face is choosing between server components and server-side rendering. Both approaches have their own advantages and use cases, but understanding the differences between them is essential for selecting the most suitable option for your project.
Server Components: A Closer Look
Server components, also known as server-side components, are reusable UI components that are rendered on the server side and sent to the client as HTML. This approach is particularly popular in modern web development frameworks like Vaadin, where the UI components are defined and managed on the server, and the server takes care of rendering the UI and handling user interactions.
One of the primary advantages of server components is their ability to encapsulate both the UI logic and server interaction within a single component. This makes it easier to maintain and reason about complex UI elements, as the component's logic is centralized on the server, reducing the complexity and potential bugs in the client-side JavaScript code.
Sample Server Component Code snippet:
// Java code for a server component using Vaadin framework
public class MyServerComponent extends Div {
public MyServerComponent() {
// Server-side logic and UI definition
setText("Hello, I am a server component!");
}
}
In the above code snippet, the MyServerComponent
class encapsulates the UI logic and rendering on the server side, providing a clean and maintainable way to define UI components.
Server-Side Rendering: Exploring its Benefits
On the other hand, Server-Side Rendering (SSR) involves rendering the UI on the server and sending the fully rendered page to the client. This approach is commonly associated with frameworks like Spring MVC, where JSP (JavaServer Pages) or Thymeleaf templates are used to render the HTML content on the server before sending it to the client.
The most significant advantage of server-side rendering is its ability to improve initial page load performance and search engine optimization (SEO). By sending pre-rendered HTML content to the client, SSR reduces the client-side processing and enhances the perceived performance of the web application, leading to better user experience and SEO rankings.
Example of Server-Side Rendering with Thymeleaf:
// Controller code using Thymeleaf for server-side rendering
@GetMapping("/products")
public String getProductPage(Model model) {
List<Product> products = productService.getAllProducts();
model.addAttribute("products", products);
return "product-list"; // Renders product-list.html on the server
}
In the above code snippet, the getProductPage
method retrieves the product data from the server and renders the product-list.html
template using Thymeleaf, demonstrating the server-side rendering approach in Java.
Choosing the Right Approach for Your Project
Both server components and server-side rendering offer unique benefits, and choosing the right approach depends on various factors such as the nature of the application, performance requirements, and development team's familiarity with the chosen technology.
For applications requiring high interactivity and complex UI behaviors, server components can provide a more robust and maintainable solution by centralizing the UI logic on the server and leveraging the power of Java for handling user interactions and data processing.
On the other hand, for content-focused applications where the initial page load performance and SEO are crucial, server-side rendering shines by delivering optimized HTML content to the client, improving user experience and search engine visibility.
Wrapping Up
In conclusion, the decision between server components and server-side rendering boils down to the specific requirements and priorities of your web application. By understanding the strengths and use cases of each approach, you can make an informed decision that aligns with your project goals and delivers a high-performing, scalable, and maintainable web application.
In the rapidly evolving landscape of web development, staying abreast of architectural choices and best practices is essential for building successful applications. Whether you opt for the encapsulation power of server components or the performance benefits of server-side rendering, making an informed choice will set the foundation for a robust and efficient web application.
Ready to dive deeper into Java web development? Check out this guide to building RESTful APIs with Spring Boot, and explore the endless possibilities of Java in web development.