Protect Your Database: Salted Security in GlassFish JDBC
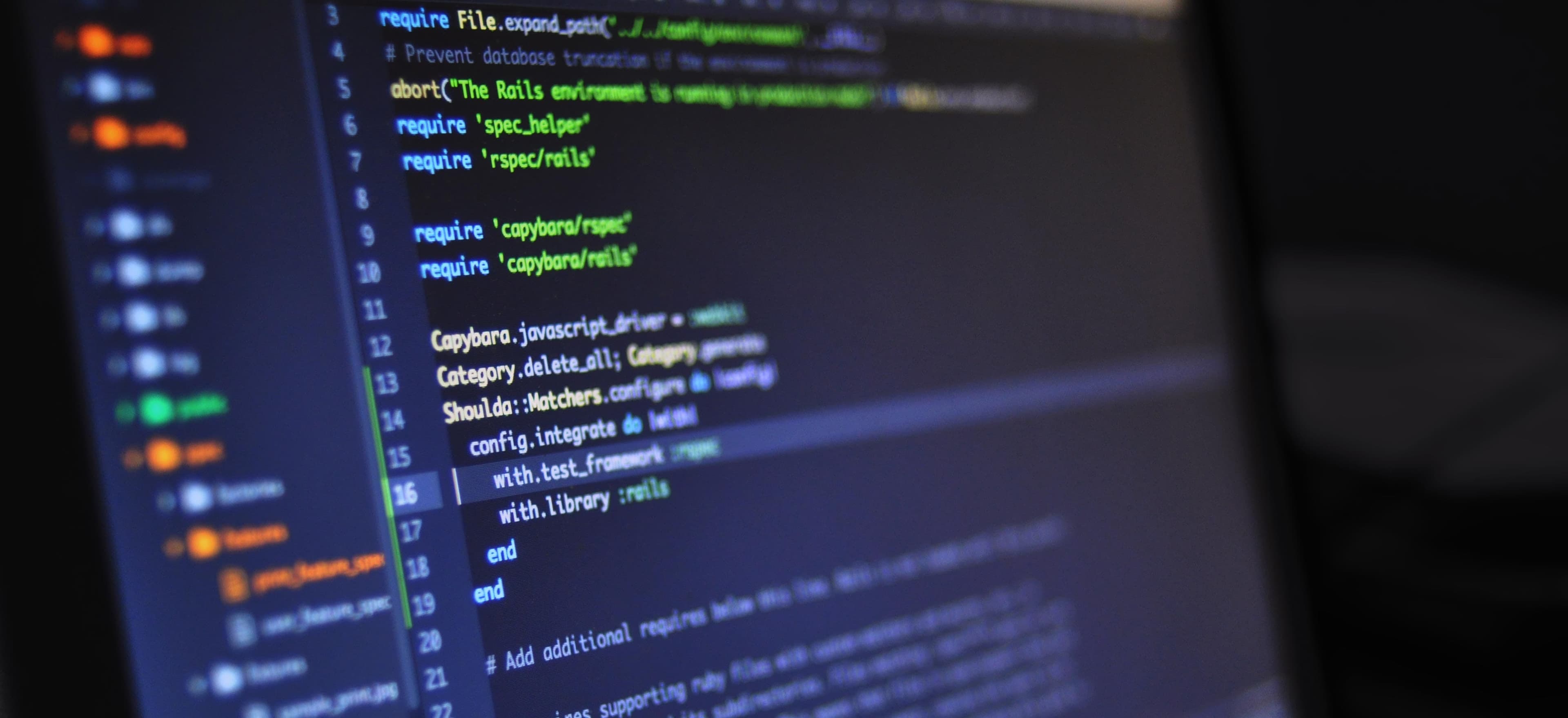
- Published on
Protect Your Database: Salted Security in GlassFish JDBC
In today's digital age, securing your database is more essential than ever. With the increasing number of security breaches, it becomes imperative to safeguard critical data. One effective method for enhancing your database security is by implementing salted hashing in your JDBC (Java Database Connectivity) setup on GlassFish. This article will explore what salted security entails, how to implement it in a GlassFish environment, and why it's crucial for protecting sensitive user information.
Understanding Salted Hashing
Salted hashing is a technique used in cryptography to secure passwords. The core principle is simple: rather than storing plain text passwords, which are easily compromised, we store a hash of the password combined with a random string, known as a “salt.”
Why Use Salted Hashing?
-
Defense Against Rainbow Table Attacks: Rainbow tables are precomputed tables for reversing cryptographic hash functions. By adding a unique salt to each password, we mitigate the risk of using such tables.
-
Unique Password Hashing: Even if two users have the same password, the salted hashes will differ because the salts are unique. This uniqueness is crucial for enhancing security.
-
Impact on Password Cracking: Cracking a salted password becomes computationally intensive and time-consuming, which deters many attackers.
Terminology
- Hash: A fixed-length string derived from input data using a hash function.
- Salt: A unique, random value added to the input before hashing.
Setting Up Salted Hashing in GlassFish JDBC
To implement salted hashing in your GlassFish JDBC setup, you will follow several steps:
- Environment Setup: Ensure you have a GlassFish server and a Java environment set up.
- Database Configuration: You'll need a MySQL or PostgreSQL database configured via JDBC.
- Code Implementation: This is the crux where we will implement the salted hashing logic.
Step 1: Configure JDBC in GlassFish
To start, ensure your GlassFish server is running, and you have connected your database. The following steps assume you are configuring a MySQL database.
- Log into your GlassFish admin console (usually at
http://localhost:4848
). - Navigate to Resources > JDBC > JDBC Resources.
- Click on New and add your database connection details.
<jdbc-connection-pool name="MyPool" res-type="javax.sql.DataSource" datasource-class="com.mysql.jdbc.jdbc2.optional.MysqlDataSource">
<property name="User" value="username"/>
<property name="Password" value="password"/>
<property name="URL" value="jdbc:mysql://localhost:3306/mydatabase"/>
</jdbc-connection-pool>
Make sure to replace username
, password
, and the database URL accordingly.
Step 2: Create the User Table
In your database, create a users table where the hashed password will be stored.
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(255) NOT NULL UNIQUE,
password_hash VARCHAR(255) NOT NULL,
salt VARCHAR(255) NOT NULL
);
Step 3: Java Code Implementation
Now, let's implement the code that will handle hashing and storing user passwords. We'll utilize Java's MessageDigest
for hashing:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.security.SecureRandom;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import javax.sql.DataSource;
public class UserService {
private DataSource dataSource;
public UserService(DataSource dataSource) {
this.dataSource = dataSource;
}
public void registerUser(String username, String password) throws Exception {
// Generate a random salt
byte[] salt = createSalt();
// Hash the password with the salt
String passwordHash = hashPassword(password, salt);
// Save the username, passwordHash, and salt to the database
try (Connection conn = dataSource.getConnection()) {
String sql = "INSERT INTO users (username, password_hash, salt) VALUES (?, ?, ?)";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setString(1, username);
stmt.setString(2, passwordHash);
stmt.setBytes(3, salt);
stmt.executeUpdate();
}
}
private byte[] createSalt() {
SecureRandom sr = new SecureRandom();
byte[] salt = new byte[16];
sr.nextBytes(salt);
return salt;
}
private String hashPassword(String password, byte[] salt) throws NoSuchAlgorithmException {
MessageDigest md = MessageDigest.getInstance("SHA-256");
md.update(salt);
return bytesToHex(md.digest(password.getBytes()));
}
private String bytesToHex(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02x", b));
}
return sb.toString();
}
public boolean validateUser(String username, String password) throws Exception {
try (Connection conn = dataSource.getConnection()) {
String sql = "SELECT password_hash, salt FROM users WHERE username=?";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setString(1, username);
ResultSet rs = stmt.executeQuery();
if (rs.next()) {
String storedHash = rs.getString("password_hash");
byte[] salt = rs.getBytes("salt");
// Hash the provided password with the stored salt
String passwordHash = hashPassword(password, salt);
return storedHash.equals(passwordHash);
}
return false;
}
}
}
Code Explanation
- DataSource: Used to manage connection pooling. Make sure your application can acquire connections from the GlassFish JDBC resource.
- Salt Generation: We use
SecureRandom
to create a unique salt. - Password Hashing: The password is hashed using
SHA-256
along with the salt. This means while the salt can be stored with the hashed password, the real password is never stored. - Registration and Validation: These methods allow an application user to register and validate their credentials securely.
Securing Your Application
By incorporating this salted hashing technique, you’re creating a barrier against various attacks that target user authentication. However, security isn't solely about password storage; consider including measures like:
- Using HTTPS: Encrypt data in transit.
- Two-Factor Authentication: Add an extra layer of security.
- Input Validation: Prevent SQL injection attacks using prepared statements.
The Bottom Line
Implementing salted security in your GlassFish JDBC setup is a pivotal step toward protecting sensitive user data. Not only does it make it significantly harder for attackers to compromise user credentials, but it also sends a clear message about your commitment to security.
For more information on database security best practices, check out OWASP's guidelines which can provide valuable insights.
In summary, security is an ongoing process, and now is the time to ensure your approach to managing user data is comprehensive. With careful planning and execution, you can protect your users and enhance the trustworthiness of your application.