Writing Clean and Readable Code
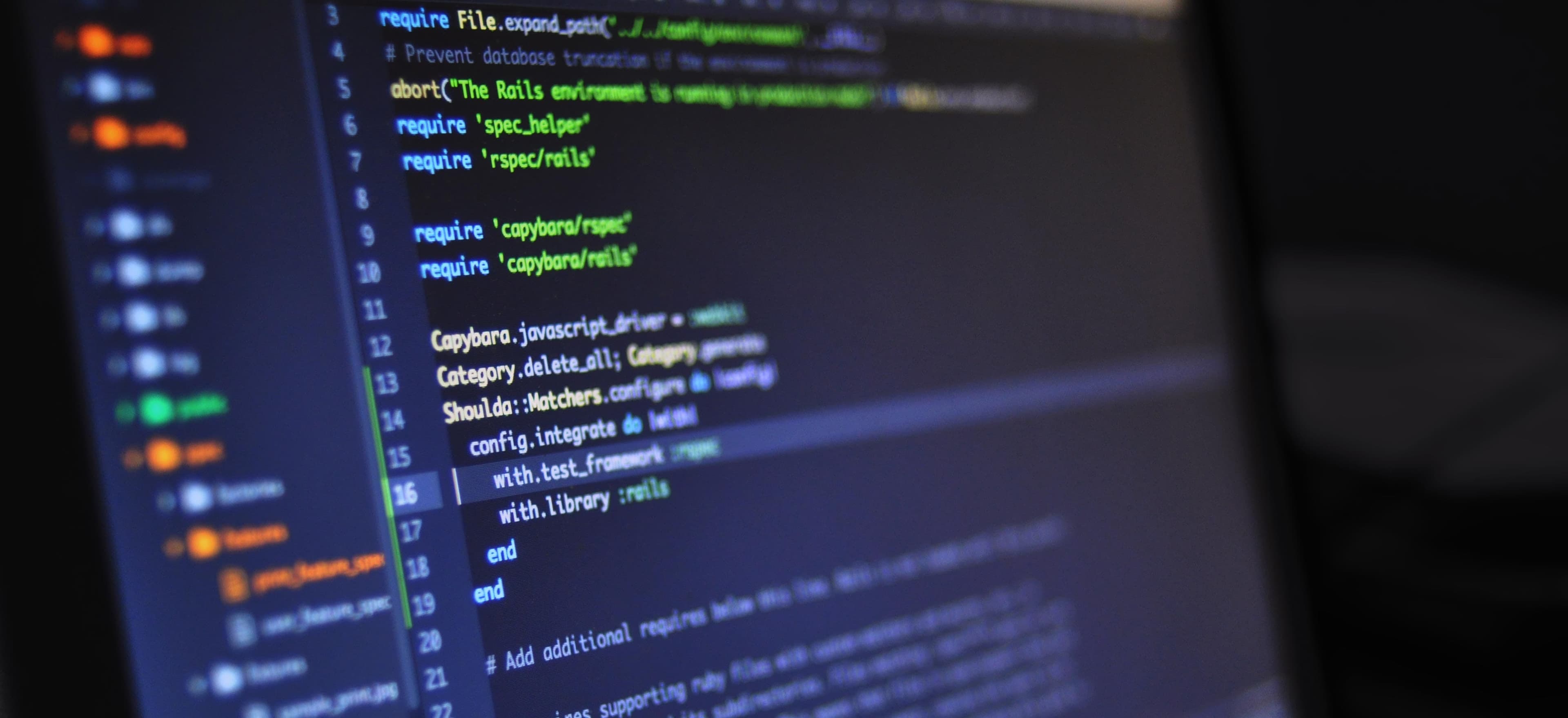
- Published on
Writing Clean and Readable Code
In the world of software development, writing clean and readable code is not just a good practice, but a necessity. Clear and concise code not only benefits the original developer but also anyone else who might work on the codebase in the future. In this post, we will discuss the importance of writing clean code and explore some best practices to achieve it in the context of Java programming.
Why Clean Code Matters
Writing clean and readable code has numerous benefits, such as:
1. Maintainability
- Clean code is easier to maintain and modify. When a bug needs to be fixed or a new feature added, clean code allows developers to understand the existing codebase quickly and accurately.
2. Collaboration
- Clean code improves collaboration among development teams. When code is easy to read and understand, it facilitates better communication among team members and reduces the likelihood of misinterpretation.
3. Scalability
- Clean code is more scalable. As the codebase grows, clean code makes it easier to extend and build upon existing functionality without introducing new bugs or unexpected behaviors.
4. Debugging
- Clean code reduces the time and effort required for debugging. Code that is well-organized and follows best practices can help in identifying and resolving issues more efficiently.
Best Practices for Writing Clean Code in Java
1. Descriptive and Intention-Revealing Names
- Use meaningful and descriptive names for variables, methods, and classes. Names should reveal the purpose or intent, making the code self-explanatory.
2. Proper Indentation and Formatting
- Follow consistent indentation and formatting standards. This improves the visual structure of the code, making it easier to navigate and understand.
3. Keep Methods Small and Cohesive
- Aim to keep methods small, focused, and cohesive. Each method should have a single responsibility, making it easier to comprehend and maintain.
4. Comments for Clarity, Not Explanation
- Use comments sparingly and focus on why rather than what. Comments should provide insight into the rationale behind certain approaches or decisions, rather than reiterating the code's functionality.
5. Error Handling
- Implement clear and concise error handling. This involves using meaningful exceptions, providing informative error messages, and avoiding unnecessary nesting of try-catch blocks.
6. Use Standard Naming Conventions
- Adhere to standard naming conventions for classes, methods, variables, and packages as prescribed by the Java community (e.g., camelCase for variables, PascalCase for class names, etc.).
// Example of Descriptive Naming
public class Car {
private String make;
private String model;
public void setMake(String make) {
this.make = make;
}
public String getMake() {
return make;
}
// ...
}
// Example of Proper Indentation and Formatting
public class Example {
public void performTask() {
if (condition) {
// do something
} else {
// do something else
}
}
// ...
}
By applying these best practices consistently, developers can create code that is easy to understand, maintain, and build upon.
Tools for Ensuring Code Quality
In addition to following best practices, developers can utilize various tools to ensure code quality and adherence to standards. Some of these tools include:
1. Checkstyle
- Checkstyle is a development tool that helps programmers write Java code that adheres to coding standards. It automates the process of checking code against a set of rules, providing instant feedback on style and formatting.
2. PMD
- PMD is a source code analyzer that finds common programming flaws like unused variables, empty catch blocks, and unnecessary object creation. It is highly configurable and can be adapted to specific coding guidelines.
3. FindBugs
- FindBugs is a program that uses static analysis to look for bugs in Java code. It can identify a wide range of potential issues, providing valuable insights into areas for improvement.
The Bottom Line
Writing clean and readable code is a fundamental aspect of professional software development, and it is particularly crucial in the context of Java programming. By adhering to best practices, utilizing appropriate tools, and maintaining a commitment to clarity and simplicity, developers can create code that is not only functional but also maintainable, scalable, and easy to collaborate on.
Adopting these principles and practices requires discipline and ongoing effort, but the long-term benefits of clean code far outweigh the initial investment. Ultimately, striving for clean and readable code leads to more efficient development processes, fewer bugs, and a more enjoyable experience for everyone involved in the software development lifecycle.
So, remember, the next time you write a piece of code, ask yourself: Is it clean? Is it readable? If not, it might be time for a refactor.
Happy coding!