Enhancing Code Modularity with Facade Pattern
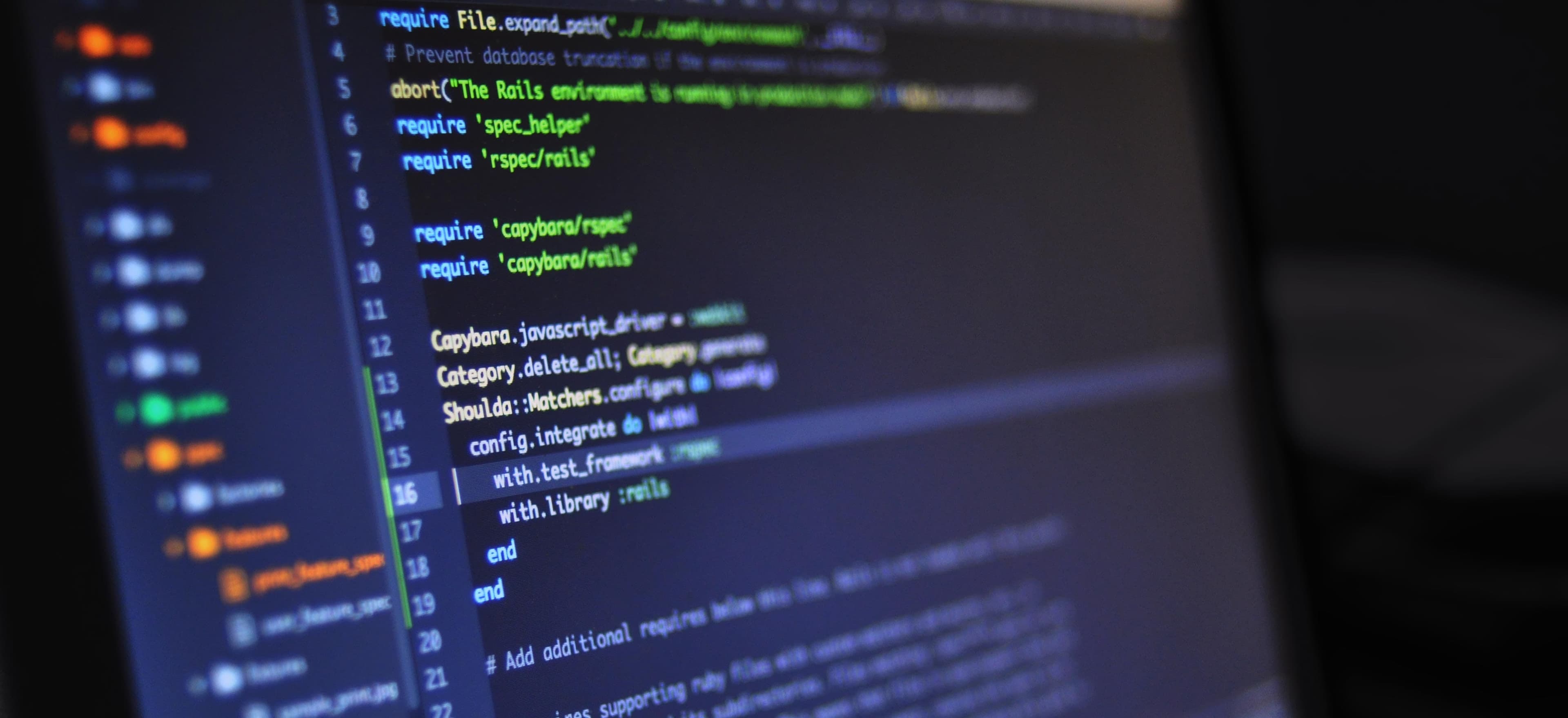
- Published on
Enhancing Code Modularity with Facade Pattern
When it comes to writing clean, modular, and maintainable Java code, patterns play a pivotal role. One such pattern that significantly enhances code modularity is the Facade pattern. In this blog post, we'll delve into the Facade pattern, its implementation in Java, and its benefits.
What is the Facade Pattern?
The Facade pattern is a structural design pattern that provides a simplified interface to a set of interfaces in a subsystem. It acts as a higher-level interface that makes the subsystem easier to use. This pattern promotes loose coupling, improves readability, and simplifies the usage of complex subsystems.
The Need for the Facade Pattern
In software development, especially in large projects, systems are often composed of multiple subsystems with numerous interconnected classes. This complexity can lead to code that's difficult to understand, maintain, and extend. Here's where the Facade pattern comes into play. It shields the client from the complexities of the subsystem and provides a simplified interface, thus enabling better modularity and encapsulation.
Implementing the Facade Pattern in Java
Let's illustrate the implementation of the Facade pattern in a Java application. Suppose we have a complex subsystem that involves multiple classes and interfaces. We can create a facade that provides a simple interface to interact with this subsystem.
// Complex subsystem interface
interface Engine {
void start();
void stop();
}
// Complex subsystem implementation
class CarEngine implements Engine {
public void start() {
System.out.println("Engine started");
}
public void stop() {
System.out.println("Engine stopped");
}
}
// Facade providing a simplified interface
class Car {
private Engine engine;
public Car() {
this.engine = new CarEngine();
}
public void start() {
engine.start();
}
public void stop() {
engine.stop();
}
}
// Client code
class Client {
public static void main(String[] args) {
Car car = new Car();
car.start();
car.stop();
}
}
In the above example, the Car
class acts as a facade, providing a simplified interface to start and stop the engine. The client code interacts with the Car
facade without needing to know the complexities of the CarEngine
subsystem.
Benefits of the Facade Pattern
Improved Modularity
The Facade pattern improves modularity by providing a single, simplified interface to a complex subsystem. This allows client code to interact with the subsystem through the facade, without needing to understand its internal workings.
Simplified Usage
By encapsulating the complexities of the subsystem, the Facade pattern simplifies the usage of the subsystem. Client code can utilize the facade without the need to manage numerous subsystem components separately.
Reduced Dependency
Facade reduces the dependency of client code on the subsystem. Any changes in the subsystem's structure or behavior can be confined within the facade, minimizing the impact on the client code.
Enhanced Readability
With the Facade pattern, the overall readability of the code improves. Client code becomes more comprehensible as it interacts with a simple, high-level interface, rather than delving into the intricacies of the subsystem.
Easy Refactoring
Since client code is decoupled from the subsystem, refactoring the subsystem's implementation becomes more manageable. The facade shields the client from the changes in the subsystem, as long as the facade's interface remains consistent.
When to Use the Facade Pattern
The Facade pattern is beneficial in various scenarios:
- When working with complex subsystems, such as libraries with numerous classes and interfaces.
- When aiming to provide a simpler interface to a complex system.
- When seeking to reduce the dependencies of client code on the subsystem.
A Final Look
The Facade pattern offers a compelling solution to enhance code modularity, simplify usage, and promote a more maintainable codebase. By encapsulating the complexities of a subsystem behind a simplified interface, the Facade pattern enables better modularity and encapsulation. Thus, it is a valuable addition to the toolbox of any Java developer working on building scalable, maintainable, and readable codebases.
In summary, the Facade pattern acts as a facilitator, transforming intricate subsystems into manageable and user-friendly components, while significantly contributing to the overall modularity of the codebase.
So, next time you find yourself grappling with a complex subsystem, consider employing the Facade pattern to streamline its usage and improve the modularity of your Java code.