Demystifying Common HTTP Status Codes
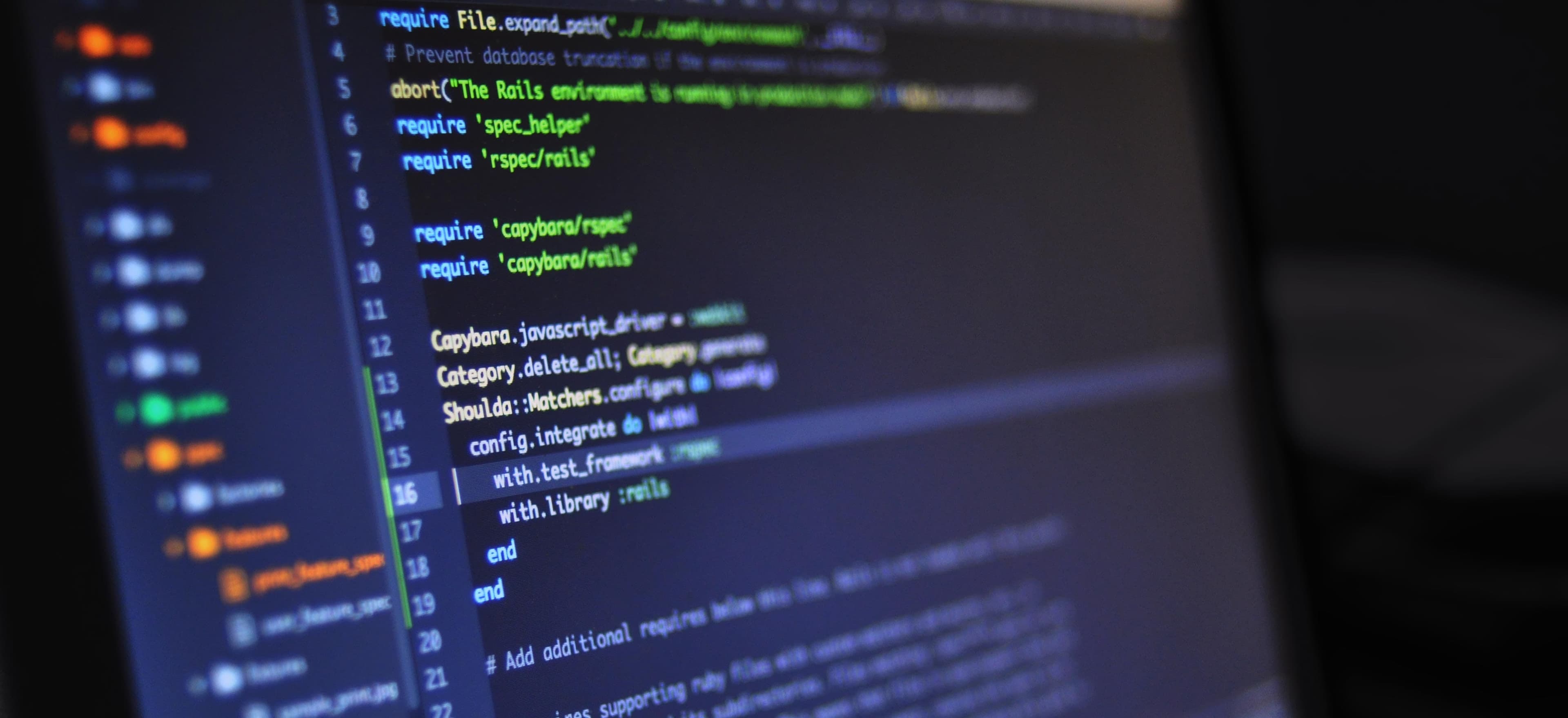
- Published on
Demystifying Common HTTP Status Codes
In the world of web development, understanding HTTP status codes is crucial for building robust and reliable web applications. These status codes are issued by a server in response to a client's request made to the server. They provide valuable information about the outcome of the request, allowing both the client and the server to handle the request appropriately.
In this post, we'll delve into some of the most common HTTP status codes, their meanings, and how they can be handled in Java web applications.
1. 200 OK
The 200 OK
status code is the standard response for a successful HTTP request. It indicates that the request has succeeded, and the server has returned the requested content. This status code is the desired outcome for most HTTP requests.
In Java, a simple example of handling a successful request with a 200 OK
status code in a servlet might look like this:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Process the request
// ...
response.setStatus(HttpServletResponse.SC_OK);
// ...
}
Here, HttpServletResponse.SC_OK
is used to set the status code to 200 OK
before sending the response back to the client. This informs the client that the request was successful.
2. 404 Not Found
The 404 Not Found
status code is returned when the server cannot find the requested resource. This often occurs when a client attempts to access a URL that does not exist on the server.
In Java, handling a 404 Not Found
error can be done by setting the status code in a servlet as follows:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Check if the requested resource exists
// ...
if (resourceNotFound) {
response.setStatus(HttpServletResponse.SC_NOT_FOUND);
// Optionally, you can customize the error page or message
// ...
}
// ...
}
By setting the status code to HttpServletResponse.SC_NOT_FOUND
, the server informs the client that the requested resource was not found.
3. 500 Internal Server Error
The 500 Internal Server Error
status code indicates that the server encountered an unexpected condition that prevented it from fulfilling the request. This could be due to a bug in the server-side logic or an issue with the server's configuration.
In Java, handling a server error can be achieved as follows:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
try {
// Server logic that may throw an exception
// ...
response.setStatus(HttpServletResponse.SC_OK);
// ...
} catch (Exception e) {
response.setStatus(HttpServletResponse.SC_INTERNAL_SERVER_ERROR);
// Log the exception for debugging and troubleshooting
// ...
}
}
By setting the status code to HttpServletResponse.SC_INTERNAL_SERVER_ERROR
within a catch
block, the server indicates to the client that an unexpected error occurred while processing the request.
4. 302 Found (Redirect)
The 302 Found
status code is used to inform the client that the requested resource has been temporarily moved to a different URL. This is often used for URL redirection.
In Java, redirecting a request with a 302 Found
status code can be done as follows:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Redirect to a new URL
// ...
response.setStatus(HttpServletResponse.SC_FOUND);
response.setHeader("Location", "https://new-url.com");
// ...
}
By setting the status code to HttpServletResponse.SC_FOUND
and providing the new location in the Location
header, the server instructs the client to issue a new request to the specified URL.
5. 401 Unauthorized
The 401 Unauthorized
status code indicates that the client must authenticate itself to get the requested response. This is commonly used when access to a resource requires authentication credentials that the client has failed to provide.
In Java, handling an unauthorized request can be accomplished as follows:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Check if the request includes valid authentication credentials
// ...
if (authenticated) {
// Process the request
// ...
} else {
response.setStatus(HttpServletResponse.SC_UNAUTHORIZED);
// Optionally, provide a custom message or challenge for authentication
// ...
}
}
By setting the status code to HttpServletResponse.SC_UNAUTHORIZED
, the server informs the client that it needs to provide valid credentials to access the requested resource.
Closing Remarks
Understanding and appropriately handling HTTP status codes is fundamental to building resilient and user-friendly web applications. In Java, servlets and JSPs provide a straightforward way to control the status codes returned to clients based on the outcome of server-side logic.
By mastering the handling of status codes, developers can effectively communicate the results of HTTP requests to clients, thereby enhancing the overall user experience.
In summary, HTTP status codes are vital communication tools between servers and clients, and knowing how to handle them in Java web applications is a significant aspect of web development.
By familiarizing yourself with the common HTTP status codes and their handling in Java, you're better equipped to build robust and reliable web applications. Connect with our Java development experts for further insights on enhancing your web development skills.