Troubleshooting NFC Read Issues on Android Devices
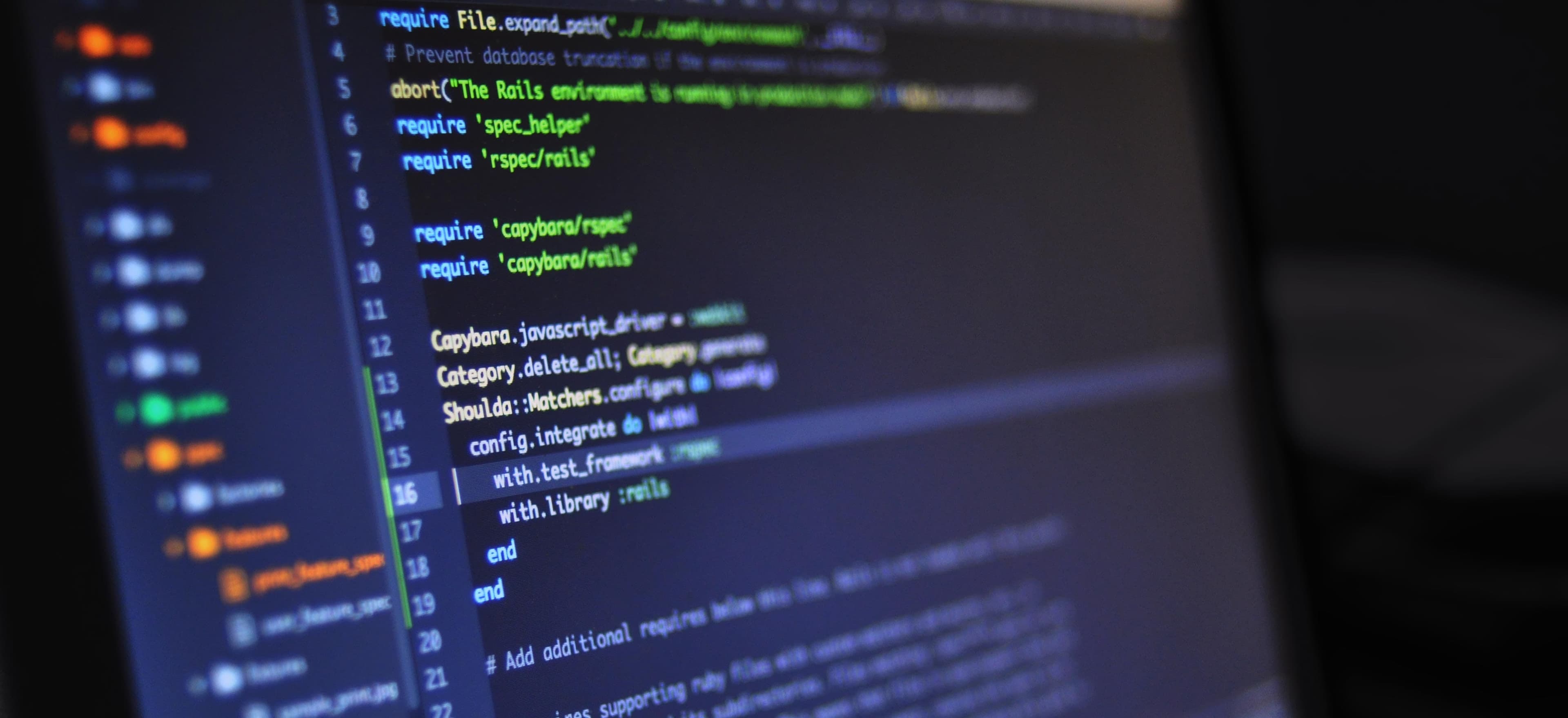
- Published on
Troubleshooting NFC Read Issues on Android Devices
NFC (Near Field Communication) technology is widely used on Android devices for various purposes, such as contactless payments, data exchange, and access control. However, users may encounter issues when trying to read NFC tags. In this blog post, we will explore common problems related to NFC tag reading on Android devices and provide troubleshooting steps to resolve these issues.
Understanding NFC Tag Reading
Before delving into troubleshooting, it is important to understand the process of NFC tag reading on Android devices. When an NFC-enabled Android device is brought close to an NFC tag, the device should automatically detect and read the information stored on the tag. This information could include URLs, contact details, or other types of data.
Common Issues with NFC Tag Reading
1. Inconsistent Tag Detection
Sometimes, Android devices may exhibit inconsistent behavior when detecting NFC tags. This could lead to situations where the device fails to recognize the tag even when it is placed within close proximity.
2. Slow Read Speed
Another common issue is slow read speed. When an NFC tag is presented to the device, the reading process should be quick and seamless. However, users may experience delays in reading the tag, impacting the overall user experience.
3. Unsupported Tag Format
Certain NFC tag formats may not be fully supported by all Android devices. This could result in the device failing to read the tag or misinterpreting the data stored on it.
Troubleshooting NFC Tag Reading Issues
1. Ensure NFC is Enabled
The first step in troubleshooting NFC tag reading issues is to ensure that NFC is enabled on the Android device. Users can check this by going to the device settings and looking for the NFC option. It should be turned on for NFC tag reading to work.
2. Check for Physical Obstructions
Physical obstructions, such as metallic objects or electronic interference, can hinder the NFC signal, leading to issues with tag detection. Users should ensure that there are no obstructions between the NFC tag and the device when attempting to read the tag.
3. Use Compatible Tags
To avoid compatibility issues, it is recommended to use NFC tags that are known to be compatible with a wide range of Android devices. Not all tags may work seamlessly with every device, so choosing tags from reputable manufacturers can help mitigate potential compatibility problems.
4. Verify Tag Encoding
Sometimes, issues with tag reading can be attributed to improper encoding of the NFC tag. Users should verify that the information encoded on the tag complies with NFC standards and is formatted correctly. This ensures that the device can read the tag's data accurately.
5. Update Android System Software
Outdated system software on Android devices can sometimes lead to NFC-related issues. Users should check for software updates in the device settings and ensure that the device is running the latest available version of Android.
6. Test with Different Apps
If issues persist, testing the NFC tag with different apps can help determine if the problem is app-specific. There are various NFC reading apps available on the Google Play Store, and using alternative apps for tag reading can provide insights into the root cause of the problem.
7. Reset NFC Preferences
Resetting NFC preferences on the Android device can help eliminate any potential configuration errors that might be causing the tag reading issues. This can be done by navigating to the device settings, locating the NFC preferences, and choosing the option to reset them to default.
8. Check for Interference from Other Apps
Certain apps running in the background on Android devices may interfere with the NFC functionality, leading to issues with tag reading. Users should check for any recently installed apps and consider temporarily disabling them to see if it resolves the problem.
Example Code for NFC Tag Reading
public class MainActivity extends AppCompatActivity {
NfcAdapter nfcAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
nfcAdapter = NfcAdapter.getDefaultAdapter(this);
if (nfcAdapter == null) {
// NFC is not supported on this device
Toast.makeText(this, "NFC is not supported on this device", Toast.LENGTH_SHORT).show();
finish();
return;
}
if (!nfcAdapter.isEnabled()) {
// NFC is disabled
Toast.makeText(this, "Please enable NFC", Toast.LENGTH_SHORT).show();
} else {
// NFC is enabled
// Handle NFC intent
handleNfcIntent(getIntent());
}
}
@Override
protected void onNewIntent(Intent intent) {
super.onNewIntent(intent);
handleNfcIntent(intent);
}
private void handleNfcIntent(Intent intent) {
if (NfcAdapter.ACTION_TAG_DISCOVERED.equals(intent.getAction())) {
Tag tag = intent.getParcelableExtra(NfcAdapter.EXTRA_TAG);
// Process the tag here
// ...
}
}
}
In the above code snippet, we demonstrate how to handle NFC tag discovery in an Android app. The onCreate
method initializes the NFC adapter and checks whether NFC is supported and enabled on the device. The handleNfcIntent
method is responsible for processing the discovered NFC tag when a corresponding intent is received.
The Last Word
NFC tag reading issues on Android devices can be frustrating for users, but by following the troubleshooting steps outlined in this blog post, many of these issues can be resolved. It is important to ensure that NFC is enabled, check for physical obstructions, use compatible tags, verify tag encoding, update the Android system software, test with different apps, reset NFC preferences, and identify potential interference from other apps. By addressing these factors, users can enhance the reliability and performance of NFC tag reading on their Android devices.
By incorporating the provided example code and following the troubleshooting steps, users can effectively address NFC tag reading issues on Android devices, ultimately providing a seamless and efficient NFC experience.