Managing Stateful Session Bean Lifecycle in Java EE
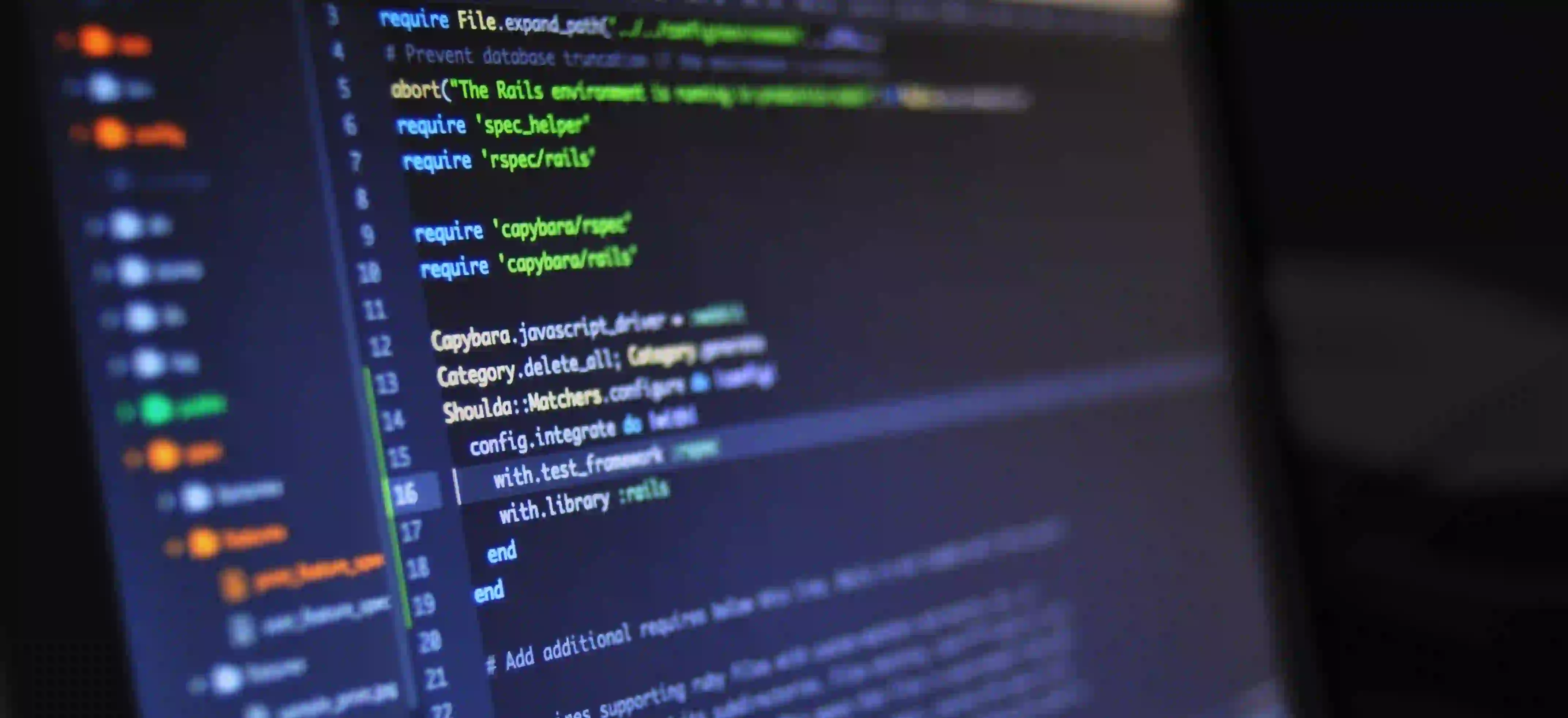
Understanding the Lifecycle of Stateful Session Beans in Java EE
Stateful Session Beans (SFSB) are an essential component of Java Enterprise Edition (Java EE) applications, providing a way to manage the state of a client across multiple method invocations. These beans are useful for maintaining conversational state with clients, making them an integral part of building scalable and reliable enterprise applications.
In this article, we will delve into the lifecycle of Stateful Session Beans in Java EE. We will explore how these beans are created, maintained, and eventually removed, and discuss best practices for managing their lifecycle effectively.
Creating Stateful Session Beans
When a client requests a new instance of a Stateful Session Bean, the container creates one specifically for that client. This instantiation process involves invoking the bean’s constructor and setting up any necessary resources. The client then holds a reference to this bean instance for the duration of the conversation.
Let's take a look at a simple example of a Stateful Session Bean and its lifecycle methods:
@Stateful
public class ShoppingCartBean {
// Lifecycle callback methods
@PostConstruct
public void initialize() {
// Initialization code here
}
@PreDestroy
public void cleanup() {
// Cleanup code here
}
// Other business methods
public void addItemToCart(Item item) {
// Add item to the cart
}
// Other business methods
}
In the example above, the ShoppingCartBean
class is annotated with @Stateful
, indicating that it is a Stateful Session Bean. Additionally, it contains lifecycle callback methods initialize()
and cleanup()
annotated with @PostConstruct
and @PreDestroy
respectively. These methods are called by the container during the bean’s lifecycle.
Lifecycle Callback Methods
@PostConstruct
The @PostConstruct
annotated method is called by the container after the bean has been instantiated, and all dependencies have been injected. This makes it an ideal place to perform any initial setup required by the bean, such as initializing resources or establishing connections. It is important to note that the @PostConstruct
method is guaranteed to be called only once in the bean’s lifecycle.
@PreDestroy
Conversely, the @PreDestroy
annotated method is invoked by the container before the bean instance is destroyed and removed from the pool. This provides an opportunity to release any acquired resources or perform cleanup operations before the bean is no longer accessible. Similar to @PostConstruct
, the @PreDestroy
method is also guaranteed to be called only once during the bean’s lifecycle.
Using these lifecycle callback methods can help in managing resource allocation and deallocation, leading to more efficient usage of resources in the application server.
Managing Stateful Session Bean State
Stateful Session Beans, as the name suggests, are designed to maintain state across multiple client invocations. This means that the state of the bean instance needs to be managed diligently to prevent memory leaks and ensure the integrity of the application’s data.
Passivation and Activation
In situations where the application server needs to free up resources, such as when the bean is idle for an extended period, it can passivate the bean instance. Passivation involves serializing the state of the bean to secondary storage, effectively “freezing” it until it is needed again. When a client invokes a method on a passivated bean, the container activates the bean, restoring its state from the serialized representation.
It is crucial to design the Stateful Session Bean in a way that allows for efficient passivation and activation. This includes properly managing the bean’s dependencies and ensuring that its state can be safely serialized and deserialized.
@PostActivate and @PrePassivate
To handle custom logic during activation and passivation, Stateful Session Beans can define the methods annotated with @PostActivate
and @PrePassivate
. These methods are called by the container after activation and before passivation, enabling the bean to perform any necessary state-related operations.
Removing Stateful Session Beans
At some point, a client’s conversation with a Stateful Session Bean will come to an end, and the bean instance will need to be removed. This can occur when the client explicitly requests the removal or when the container decides to remove it to free up resources.
Client-Initiated Removal
Clients can explicitly request the removal of a Stateful Session Bean by invoking the @Remove
annotated method. This signals to the container that the client no longer requires the bean and initiates the removal process, allowing the bean instance to be reclaimed and its resources released.
@Stateful
public class ShoppingCartBean {
// Other business methods
@Remove
public void checkout() {
// Perform checkout operations
}
// Other business methods
}
In this example, the checkout()
method is annotated with @Remove
, indicating that it triggers the removal of the Stateful Session Bean instance.
Container-Initiated Removal
When the application server determines that a Stateful Session Bean instance is no longer in use or needs to be passivated, it can remove the bean to reclaim resources. This automatic removal process helps in managing memory and prevents resource exhaustion in the application server.
Final Thoughts
Understanding the lifecycle of Stateful Session Beans is crucial for developing robust and efficient Java EE applications. By comprehending how these beans are created, managed, and removed, developers can design their applications to handle stateful interactions with clients effectively.
In this article, we have explored the creation of Stateful Session Beans, the significance of lifecycle callback methods, the management of bean state through passivation and activation, and the removal of bean instances. By leveraging the lifecycle management features provided by Java EE, developers can build scalable and reliable applications that cater to the conversational state requirements of their clients.